ODE parameter prediction
We will perform ODE parameter prediction for forecasting of the number of cases. We have two ways for prediction.
Time-series prediction withOUT indicators
Time-series prediction with indicators
The second one uses indicators, including OxCGRT indicators, the number of vaccinations.
[1]:
from datetime import timedelta
import covsirphy as cs
from matplotlib import pyplot as plt
import numpy as np
cs.__version__
[1]:
'3.1.2'
The target of prediction is estimated ODE parameter values. At we will prepare them as explained with tutorials. For example, we can use class method ODEScenario.auto_build()
, specifying location name. “Baseline” scenario will be created automatically with downloaded datasets.
[2]:
snr = cs.ODEScenario.auto_build(geo="Japan", model=cs.SIRFModel)
# Show summary
snr.summary()
2024-10-03 at 13:24:15 | INFO |
<SIR-F Model: parameter estimation>
2024-10-03 at 13:24:15 | INFO | Running optimization with 4 CPUs...
2024-10-03 at 13:26:28 | INFO | Completed optimization. Total: 2 min 12 sec
[2]:
Start | End | Rt | theta | kappa | rho | sigma | alpha1 [-] | 1/alpha2 [day] | 1/beta [day] | 1/gamma [day] | ODE | tau | ||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Scenario | Phase | |||||||||||||
Baseline | 0th | 2020-02-23 | 2020-08-10 | 1.36 | 0.098322 | 0.000018 | 0.001499 | 0.000973 | 0.098 | 937 | 11 | 17 | SIR-F Model | 24 |
1st | 2020-08-11 | 2020-11-16 | 0.81 | 0.007052 | 0.000006 | 0.000903 | 0.001096 | 0.007 | 2845 | 18 | 15 | SIR-F Model | 24 | |
2nd | 2020-11-17 | 2020-12-24 | 1.71 | 0.000613 | 0.000013 | 0.001048 | 0.000599 | 0.001 | 1327 | 16 | 28 | SIR-F Model | 24 | |
3rd | 2020-12-25 | 2021-01-16 | 1.47 | 0.000781 | 0.000013 | 0.001388 | 0.000933 | 0.001 | 1256 | 12 | 18 | SIR-F Model | 24 | |
4th | 2021-01-17 | 2021-02-10 | 0.77 | 0.000047 | 0.000017 | 0.000898 | 0.001154 | 0.0 | 997 | 19 | 14 | SIR-F Model | 24 | |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | |
63rd | 2023-03-12 | 2023-03-27 | 1.68 | 0.007818 | 0.000003 | 0.001113 | 0.000654 | 0.008 | 5738 | 15 | 26 | SIR-F Model | 24 | |
64th | 2023-03-28 | 2023-04-07 | 0.84 | 0.000241 | 0.000004 | 0.001836 | 0.002185 | 0.0 | 4736 | 9 | 8 | SIR-F Model | 24 | |
65th | 2023-04-08 | 2023-04-18 | 1.45 | 0.000587 | 0.000003 | 0.001329 | 0.000912 | 0.001 | 6343 | 13 | 18 | SIR-F Model | 24 | |
66th | 2023-04-19 | 2023-04-27 | 1.81 | 0.000873 | 0.000004 | 0.001388 | 0.000762 | 0.001 | 4087 | 12 | 22 | SIR-F Model | 24 | |
67th | 2023-04-28 | 2023-05-08 | 1.65 | 0.000772 | 0.000001 | 0.00116 | 0.000703 | 0.001 | 14311 | 14 | 24 | SIR-F Model | 24 |
68 rows × 13 columns
For demonstration, we will get the start date of future phases.
[3]:
future_start_date = snr.simulate(display=False).index.max() + timedelta(days=1)
future_start_date
[3]:
Timestamp('2023-05-09 00:00:00')
1. Time-series prediction withOUT indicators
This scenario “Predicted” does not use indicators, using AutoTS package: a time series package for Python designed for rapidly deploying high-accuracy forecasts at scale.
At first, create “Predicted” scenario, copying estimated ODE parameter values of “Baseline” scenario.
[4]:
snr.build_with_template(name="Predicted", template="Baseline");
Then, run ODEScenario().predict(days, name, **kwargs)
. We can apply keyword arguments of autots.AutoTS()
except for forecast_length
(always the same as days
).
[5]:
snr.predict(days=30, name="Predicted");
Using 1 cpus for n_jobs.
Data frequency is: D, used frequency is: D
Model Number: 1 with model AverageValueNaive in generation 0 of 1 with params {"method": "Mean"} and transformations {"fillna": "fake_date", "transformations": {"0": "DifferencedTransformer", "1": "SinTrend"}, "transformation_params": {"0": {}, "1": {}}}
Model Number: 2 with model AverageValueNaive in generation 0 of 1 with params {"method": "Mean"} and transformations {"fillna": "mean", "transformations": {"0": "ClipOutliers", "1": "QuantileTransformer", "2": "DifferencedTransformer"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 3, "fillna": null}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {}}}
Model Number: 3 with model AverageValueNaive in generation 0 of 1 with params {"method": "Mean"} and transformations {"fillna": "rolling_mean_24", "transformations": {"0": "SeasonalDifference", "1": "Round", "2": "Detrend"}, "transformation_params": {"0": {"lag_1": 7, "method": "Mean"}, "1": {"model": "middle", "decimals": 2, "on_transform": true, "on_inverse": false}, "2": {"model": "GLS"}}}
Model Number: 4 with model GLS in generation 0 of 1 with params {} and transformations {"fillna": "rolling_mean", "transformations": {"0": "RollingMeanTransformer", "1": "DifferencedTransformer", "2": "Detrend", "3": "Slice"}, "transformation_params": {"0": {"fixed": true, "window": 3}, "1": {}, "2": {"model": "Linear"}, "3": {"method": 100}}}
Model Number: 5 with model GLS in generation 0 of 1 with params {} and transformations {"fillna": "median", "transformations": {"0": "ClipOutliers", "1": "QuantileTransformer", "2": "RobustScaler", "3": "Round", "4": "MaxAbsScaler"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 3.5, "fillna": null}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {}, "3": {"model": "middle", "decimals": 2, "on_transform": true, "on_inverse": true}, "4": {}}}
Model Number: 6 with model LastValueNaive in generation 0 of 1 with params {} and transformations {"fillna": "mean", "transformations": {"0": "bkfilter", "1": "SinTrend", "2": "Detrend", "3": "PowerTransformer"}, "transformation_params": {"0": {}, "1": {}, "2": {"model": "Linear"}, "3": {}}}
Model Number: 7 with model LastValueNaive in generation 0 of 1 with params {} and transformations {"fillna": "rolling_mean_24", "transformations": {"0": "PositiveShift", "1": "SinTrend", "2": "bkfilter"}, "transformation_params": {"0": {}, "1": {}, "2": {}}}
Model Number: 8 with model LastValueNaive in generation 0 of 1 with params {} and transformations {"fillna": "fake_date", "transformations": {"0": "SeasonalDifference", "1": "SinTrend"}, "transformation_params": {"0": {"lag_1": 7, "method": "LastValue"}, "1": {}}}
Model Number: 9 with model LastValueNaive in generation 0 of 1 with params {} and transformations {"fillna": "mean", "transformations": {"0": "ClipOutliers", "1": "QuantileTransformer"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 1, "fillna": null}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}}}
Model Number: 10 with model SeasonalNaive in generation 0 of 1 with params {"method": "LastValue", "lag_1": 2, "lag_2": 7} and transformations {"fillna": "rolling_mean_24", "transformations": {"0": "SinTrend", "1": "Round", "2": "PowerTransformer"}, "transformation_params": {"0": {}, "1": {"model": "middle", "decimals": 2, "on_transform": false, "on_inverse": true}, "2": {}}}
Template Eval Error: Traceback (most recent call last):
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/tools/transform.py", line 5489, in _fit
df = self._fit_one(df, i)
^^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/tools/transform.py", line 5466, in _fit_one
df = self.transformers[i].fit_transform(df)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/sklearn/utils/_set_output.py", line 313, in wrapped
data_to_wrap = f(self, X, *args, **kwargs)
^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/sklearn/base.py", line 1473, in wrapper
return fit_method(estimator, *args, **kwargs)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/sklearn/preprocessing/_data.py", line 3272, in fit_transform
return self._fit(X, y, force_transform=True)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/sklearn/preprocessing/_data.py", line 3304, in _fit
self.lambdas_[i] = optim_function(col)
^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/sklearn/preprocessing/_data.py", line 3493, in _yeo_johnson_optimize
return optimize.brent(_neg_log_likelihood, brack=(-2, 2))
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/scipy/optimize/_optimize.py", line 2626, in brent
res = _minimize_scalar_brent(func, brack, args, **options)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/scipy/optimize/_optimize.py", line 2663, in _minimize_scalar_brent
brent.optimize()
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/scipy/optimize/_optimize.py", line 2433, in optimize
xa, xb, xc, fa, fb, fc, funcalls = self.get_bracket_info()
^^^^^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/scipy/optimize/_optimize.py", line 2402, in get_bracket_info
xa, xb, xc, fa, fb, fc, funcalls = bracket(func, xa=brack[0],
^^^^^^^^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/scipy/optimize/_optimize.py", line 3032, in bracket
raise e
scipy.optimize._optimize.BracketError: The algorithm terminated without finding a valid bracket. Consider trying different initial points.
The above exception was the direct cause of the following exception:
Traceback (most recent call last):
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 1646, in TemplateWizard
df_forecast = model_forecast(
^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 1493, in model_forecast
model = model.fit(df_train_low, future_regressor_train)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 860, in fit
df_train_transformed = self.transformer_object._fit(df)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/tools/transform.py", line 5494, in _fit
raise Exception(err_str) from e
Exception: Transformer PowerTransformer failed on fit from params rolling_mean_24 {'0': {}, '1': {'model': 'middle', 'decimals': 2, 'on_transform': False, 'on_inverse': True}, '2': {}}
in model 10 in generation 0: SeasonalNaive
Model Number: 11 with model SeasonalNaive in generation 0 of 1 with params {"method": "LastValue", "lag_1": 2, "lag_2": 1} and transformations {"fillna": "rolling_mean_24", "transformations": {"0": "SeasonalDifference", "1": "QuantileTransformer", "2": "Detrend"}, "transformation_params": {"0": {"lag_1": 12, "method": "Median"}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {"model": "GLS"}}}
Model Number: 12 with model SeasonalNaive in generation 0 of 1 with params {"method": "LastValue", "lag_1": 7, "lag_2": 2} and transformations {"fillna": "mean", "transformations": {"0": "QuantileTransformer", "1": "ClipOutliers"}, "transformation_params": {"0": {"output_distribution": "uniform", "n_quantiles": 1000}, "1": {"method": "clip", "std_threshold": 2, "fillna": null}}}
Model Number: 13 with model ConstantNaive in generation 0 of 1 with params {} and transformations {"fillna": "ffill", "transformations": {"0": "PowerTransformer", "1": "QuantileTransformer", "2": "SeasonalDifference"}, "transformation_params": {"0": {}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {"lag_1": 7, "method": "LastValue"}}}
/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/sklearn/preprocessing/_data.py:3438: RuntimeWarning: overflow encountered in power
out[pos] = (np.power(x[pos] + 1, lmbda) - 1) / lmbda
Model Number: 14 with model SeasonalNaive in generation 0 of 1 with params {"method": "lastvalue", "lag_1": 364, "lag_2": 28} and transformations {"fillna": "ffill", "transformations": {"0": "SeasonalDifference", "1": "MaxAbsScaler", "2": "Round"}, "transformation_params": {"0": {"lag_1": 7, "method": "LastValue"}, "1": {}, "2": {"decimals": 0, "on_transform": false, "on_inverse": true}}}
Model Number: 15 with model SectionalMotif in generation 0 of 1 with params {"window": 10, "point_method": "weighted_mean", "distance_metric": "sokalmichener", "include_differenced": true, "k": 20, "stride_size": 1, "regression_type": null} and transformations {"fillna": "zero", "transformations": {"0": null}, "transformation_params": {"0": {}}}
Model Number: 16 with model SectionalMotif in generation 0 of 1 with params {"window": 5, "point_method": "midhinge", "distance_metric": "canberra", "include_differenced": false, "k": 10, "stride_size": 1, "regression_type": null} and transformations {"fillna": "rolling_mean_24", "transformations": {"0": "QuantileTransformer", "1": "QuantileTransformer", "2": "RobustScaler"}, "transformation_params": {"0": {"output_distribution": "uniform", "n_quantiles": 1000}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {}}}
Model Number: 17 with model SeasonalNaive in generation 0 of 1 with params {"method": "lastvalue", "lag_1": 364, "lag_2": 30} and transformations {"fillna": "fake_date", "transformations": {"0": "SeasonalDifference", "1": "MaxAbsScaler", "2": "Round"}, "transformation_params": {"0": {"lag_1": 7, "method": "LastValue"}, "1": {}, "2": {"decimals": 0, "on_transform": false, "on_inverse": true}}}
Model Number: 18 with model SeasonalityMotif in generation 0 of 1 with params {"window": 5, "point_method": "weighted_mean", "distance_metric": "mae", "k": 10, "datepart_method": "common_fourier"} and transformations {"fillna": "nearest", "transformations": {"0": "AlignLastValue"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "multiplicative", "strength": 1.0, "first_value_only": false}}}
Model Number: 19 with model SectionalMotif in generation 0 of 1 with params {"window": 7, "point_method": "median", "distance_metric": "canberra", "include_differenced": true, "k": 5, "stride_size": 1, "regression_type": null} and transformations {"fillna": "ffill", "transformations": {"0": "SeasonalDifference", "1": "AlignLastValue", "2": "SeasonalDifference", "3": "LevelShiftTransformer"}, "transformation_params": {"0": {"lag_1": 7, "method": "Median"}, "1": {"rows": 1, "lag": 2, "method": "additive", "strength": 1.0, "first_value_only": false}, "2": {"lag_1": 12, "method": 5}, "3": {"window_size": 30, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 10, "alignment": "average"}}}
Model Number: 20 with model ConstantNaive in generation 0 of 1 with params {"constant": 1} and transformations {"fillna": "zero", "transformations": {"0": "ClipOutliers", "1": "Detrend", "2": "AnomalyRemoval"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 3, "fillna": null}, "1": {"model": "GLS", "phi": 1, "window": 30, "transform_dict": null}, "2": {"method": "zscore", "method_params": {"distribution": "chi2", "alpha": 0.1}, "fillna": "fake_date", "transform_dict": {"fillna": "quadratic", "transformations": {"0": "AlignLastValue"}, "transformation_params": {"0": {"rows": 4, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}, "isolated_only": false}}}
Model Number: 21 with model LastValueNaive in generation 0 of 1 with params {} and transformations {"fillna": "nearest", "transformations": {"0": "LevelShiftTransformer", "1": "MaxAbsScaler", "2": "AnomalyRemoval", "3": "AlignLastValue", "4": "AlignLastValue"}, "transformation_params": {"0": {"window_size": 90, "alpha": 3.0, "grouping_forward_limit": 3, "max_level_shifts": 5, "alignment": "rolling_diff"}, "1": {}, "2": {"method": "IQR", "method_params": {"iqr_threshold": 1.5, "iqr_quantiles": [0.4, 0.6]}, "fillna": "linear", "transform_dict": null, "isolated_only": true}, "3": {"rows": 1, "lag": 1, "method": "multiplicative", "strength": 0.7, "first_value_only": false, "threshold": 1, "threshold_method": "max"}, "4": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.5, "first_value_only": false, "threshold": 10, "threshold_method": "mean"}}}
Model Number: 22 with model AverageValueNaive in generation 0 of 1 with params {"method": "Median", "window": null} and transformations {"fillna": "ffill", "transformations": {"0": "Detrend", "1": "Detrend"}, "transformation_params": {"0": {"model": "Linear", "phi": 1, "window": null, "transform_dict": null}, "1": {"model": "Linear", "phi": 1, "window": null, "transform_dict": {"fillna": null, "transformations": {"0": "ClipOutliers"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 4}}}}}}
Model Number: 23 with model GLS in generation 0 of 1 with params {} and transformations {"fillna": "akima", "transformations": {"0": "AlignLastValue", "1": "AnomalyRemoval", "2": "Discretize", "3": "Discretize", "4": "DifferencedTransformer"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}, "1": {"method": "IQR", "method_params": {"iqr_threshold": 3.0, "iqr_quantiles": [0.25, 0.75]}, "fillna": "ffill", "transform_dict": null, "isolated_only": false}, "2": {"discretization": "upper", "n_bins": 5}, "3": {"discretization": "center", "n_bins": 5}, "4": {"lag": 1, "fill": "zero"}}}
Model Number: 24 with model SeasonalNaive in generation 0 of 1 with params {"method": "lastvalue", "lag_1": 2, "lag_2": 60} and transformations {"fillna": "ffill", "transformations": {"0": "ClipOutliers", "1": "Detrend"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 4, "fillna": null}, "1": {"model": "GLS", "phi": 1, "window": null, "transform_dict": {"fillna": null, "transformations": {"0": "ClipOutliers"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 4}}}}}}
Model Number: 25 with model SeasonalityMotif in generation 0 of 1 with params {"window": 5, "point_method": "midhinge", "distance_metric": "mae", "k": 1, "datepart_method": "recurring", "independent": false} and transformations {"fillna": "ffill", "transformations": {"0": "AlignLastValue", "1": "HPFilter"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 10, "threshold_method": "max"}, "1": {"part": "trend", "lamb": 1600}}}
Model Number: 26 with model SectionalMotif in generation 0 of 1 with params {"window": 10, "point_method": "mean", "distance_metric": "matching", "include_differenced": true, "k": 10, "stride_size": 1, "regression_type": null} and transformations {"fillna": "pchip", "transformations": {"0": "PCA", "1": "AlignLastValue", "2": "DiffSmoother", "3": "QuantileTransformer", "4": "AlignLastValue"}, "transformation_params": {"0": {"whiten": false, "n_components": null}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}, "2": {"method": "rolling_zscore", "method_params": {"distribution": "gamma", "alpha": 0.1, "rolling_periods": 200, "center": true}, "transform_dict": null, "reverse_alignment": true, "isolated_only": true, "fillna": "linear"}, "3": {"output_distribution": "uniform", "n_quantiles": "quarter"}, "4": {"rows": 1, "lag": 1, "method": "multiplicative", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}}}
Model Number: 27 with model SeasonalNaive in generation 0 of 1 with params {"method": "median", "lag_1": 24, "lag_2": 28} and transformations {"fillna": "ffill_mean_biased", "transformations": {"0": "AlignLastValue"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 10, "threshold_method": "max"}}}
Model Number: 28 with model SectionalMotif in generation 0 of 1 with params {"window": 10, "point_method": "weighted_mean", "distance_metric": "chebyshev", "include_differenced": true, "k": 10, "stride_size": 1, "regression_type": null} and transformations {"fillna": "mean", "transformations": {"0": "ClipOutliers", "1": "Detrend", "2": "PowerTransformer", "3": "AlignLastValue"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 4, "fillna": null}, "1": {"model": "Linear", "phi": 0.999, "window": null, "transform_dict": null}, "2": {}, "3": {"rows": 1, "lag": 28, "method": "multiplicative", "strength": 0.9, "first_value_only": false, "threshold": null, "threshold_method": "mean"}}}
Template Eval Error: Traceback (most recent call last):
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 1646, in TemplateWizard
df_forecast = model_forecast(
^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 1494, in model_forecast
return model.predict(
^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 989, in predict
raise ValueError(
ValueError: Model returned NaN due to a preprocessing transformer {'fillna': 'mean', 'transformations': {'0': 'ClipOutliers', '1': 'Detrend', '2': 'PowerTransformer', '3': 'AlignLastValue'}, 'transformation_params': {'0': {'method': 'clip', 'std_threshold': 4, 'fillna': None}, '1': {'model': 'Linear', 'phi': 0.999, 'window': None, 'transform_dict': None}, '2': {}, '3': {'rows': 1, 'lag': 28, 'method': 'multiplicative', 'strength': 0.9, 'first_value_only': False, 'threshold': None, 'threshold_method': 'mean'}}}. fail_on_forecast_nan=True
in model 28 in generation 0: SectionalMotif
Model Number: 29 with model AverageValueNaive in generation 0 of 1 with params {"method": "Mean", "window": null} and transformations {"fillna": "quadratic", "transformations": {"0": "STLFilter"}, "transformation_params": {"0": {"decomp_type": "STL", "part": "trend", "seasonal": 7}}}
Model Number: 30 with model LastValueNaive in generation 0 of 1 with params {} and transformations {"fillna": "rolling_mean_24", "transformations": {"0": "EWMAFilter", "1": "MinMaxScaler", "2": "LevelShiftTransformer", "3": "AlignLastValue"}, "transformation_params": {"0": {"span": 3}, "1": {}, "2": {"window_size": 90, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 30, "alignment": "last_value"}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "max"}}}
Model Number: 31 with model AverageValueNaive in generation 0 of 1 with params {"method": "Weighted_Mean", "window": 168} and transformations {"fillna": "quadratic", "transformations": {"0": "AlignLastValue", "1": "LevelShiftTransformer", "2": "AlignLastValue", "3": "SeasonalDifference"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}, "1": {"window_size": 90, "alpha": 2.5, "grouping_forward_limit": 4, "max_level_shifts": 5, "alignment": "average"}, "2": {"rows": 1, "lag": 28, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "max"}, "3": {"lag_1": 7, "method": "LastValue"}}}
Model Number: 32 with model AverageValueNaive in generation 0 of 1 with params {"method": "Exp_Weighted_Mean", "window": null} and transformations {"fillna": "nearest", "transformations": {"0": null}, "transformation_params": {"0": {}}}
Model Number: 33 with model SectionalMotif in generation 0 of 1 with params {"window": 50, "point_method": "weighted_mean", "distance_metric": "russellrao", "include_differenced": true, "k": 10, "stride_size": 1, "regression_type": null} and transformations {"fillna": "ffill", "transformations": {"0": "AlignLastValue", "1": "RegressionFilter", "2": "LevelShiftTransformer"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "multiplicative", "strength": 1.0, "first_value_only": true, "threshold": null, "threshold_method": "mean"}, "1": {"sigma": 3, "rolling_window": 90, "run_order": "season_first", "regression_params": {"regression_model": {"model": "ElasticNet", "model_params": {"l1_ratio": 0.5, "fit_intercept": true, "selection": "cyclic"}}, "datepart_method": "recurring", "polynomial_degree": null, "transform_dict": {"fillna": null, "transformations": {"0": "EWMAFilter"}, "transformation_params": {"0": {"span": 7}}}, "holiday_countries_used": false, "lags": null, "forward_lags": null}, "holiday_params": null, "trend_method": "rolling_mean"}, "2": {"window_size": 90, "alpha": 2.5, "grouping_forward_limit": 3, "max_level_shifts": 5, "alignment": "average"}}}
Model Number: 34 with model SectionalMotif in generation 0 of 1 with params {"window": 10, "point_method": "median", "distance_metric": "cityblock", "include_differenced": true, "k": 10, "stride_size": 1, "regression_type": "User"} and transformations {"fillna": "ffill_mean_biased", "transformations": {"0": "EWMAFilter", "1": "StandardScaler"}, "transformation_params": {"0": {"span": 12}, "1": {}}}
Template Eval Error: Traceback (most recent call last):
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 1646, in TemplateWizard
df_forecast = model_forecast(
^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 1493, in model_forecast
model = model.fit(df_train_low, future_regressor_train)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 868, in fit
self.model = self.model.fit(
^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/models/basics.py", line 1828, in fit
raise ValueError(
ValueError: regression_type=='User' but no future_regressor supplied
in model 34 in generation 0: SectionalMotif
Model Number: 35 with model AverageValueNaive in generation 0 of 1 with params {"method": "Weighted_Mean", "window": 2} and transformations {"fillna": "KNNImputer", "transformations": {"0": "Slice", "1": "AlignLastValue"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}
Model Number: 36 with model GLS in generation 0 of 1 with params {} and transformations {"fillna": "linear", "transformations": {"0": "ClipOutliers", "1": "Detrend", "2": "PowerTransformer", "3": "AlignLastValue"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 3, "fillna": null}, "1": {"model": "Linear", "phi": 1, "window": null, "transform_dict": {"fillna": null, "transformations": {"0": "EWMAFilter"}, "transformation_params": {"0": {"span": 2}}}}, "2": {}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.7, "first_value_only": true, "threshold": null, "threshold_method": "max"}}}
Model Number: 37 with model ConstantNaive in generation 0 of 1 with params {"constant": 1} and transformations {"fillna": "fake_date", "transformations": {"0": "AlignLastValue", "1": "KalmanSmoothing"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": true, "threshold": null, "threshold_method": "mean"}, "1": {"model_name": "spline", "state_transition": [[2, -1], [1, 0]], "process_noise": [[1, 0], [0, 0]], "observation_model": [[1, 0]], "observation_noise": 0.1, "em_iter": null, "on_transform": true, "on_inverse": false}}}
Model Number: 38 with model GLS in generation 0 of 1 with params {} and transformations {"fillna": "fake_date", "transformations": {"0": "HolidayTransformer", "1": "FFTFilter", "2": "CumSumTransformer", "3": "SeasonalDifference"}, "transformation_params": {"0": {"threshold": 0.8, "splash_threshold": null, "use_dayofmonth_holidays": true, "use_wkdom_holidays": true, "use_wkdeom_holidays": false, "use_lunar_holidays": false, "use_lunar_weekday": false, "use_islamic_holidays": true, "use_hebrew_holidays": false, "anomaly_detector_params": {"method": "mad", "method_params": {"distribution": "uniform", "alpha": 0.05}, "fillna": "ffill", "transform_dict": {"transformations": {"0": "DatepartRegression"}, "transformation_params": {"0": {"datepart_method": "simple_3", "regression_model": {"model": "FastRidge", "model_params": {}}}}}, "isolated_only": false}, "remove_excess_anomalies": true, "impact": "regression", "regression_params": {}}, "1": {"cutoff": 0.1, "reverse": false}, "2": {}, "3": {"lag_1": 7, "method": "Mean"}}}
Model Number: 39 with model ConstantNaive in generation 0 of 1 with params {"constant": 0} and transformations {"fillna": "ffill", "transformations": {"0": "StandardScaler", "1": "Round", "2": "IntermittentOccurrence"}, "transformation_params": {"0": {}, "1": {"decimals": 1, "on_transform": true, "on_inverse": false}, "2": {"center": "mean"}}}
Model Number: 40 with model GLS in generation 0 of 1 with params {} and transformations {"fillna": "cubic", "transformations": {"0": "ClipOutliers", "1": "Detrend", "2": "DiffSmoother", "3": "AlignLastValue"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 3, "fillna": null}, "1": {"model": "Linear", "phi": 1, "window": 900, "transform_dict": null}, "2": {"method": null, "method_params": null, "transform_dict": null, "reverse_alignment": true, "isolated_only": false, "fillna": 1.0}, "3": {"rows": 7, "lag": 28, "method": "additive", "strength": 0.7, "first_value_only": false, "threshold": null, "threshold_method": "max"}}}
/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/sklearn/linear_model/_ridge.py:216: LinAlgWarning: Ill-conditioned matrix (rcond=9.35282e-26): result may not be accurate.
return linalg.solve(A, Xy, assume_a="pos", overwrite_a=True).T
Model Number: 41 with model AverageValueNaive in generation 0 of 1 with params {"method": "Mean", "window": null} and transformations {"fillna": "akima", "transformations": {"0": "LevelShiftTransformer"}, "transformation_params": {"0": {"window_size": 364, "alpha": 3.0, "grouping_forward_limit": 4, "max_level_shifts": 5, "alignment": "average"}}}
Model Number: 42 with model SeasonalityMotif in generation 0 of 1 with params {"window": 10, "point_method": "median", "distance_metric": "minkowski", "k": 20, "datepart_method": "simple_2", "independent": false} and transformations {"fillna": "time", "transformations": {"0": "bkfilter", "1": "MaxAbsScaler", "2": "StandardScaler", "3": "QuantileTransformer", "4": "cffilter"}, "transformation_params": {"0": {}, "1": {}, "2": {}, "3": {"output_distribution": "uniform", "n_quantiles": 20}, "4": {}}}
Model Number: 43 with model SeasonalityMotif in generation 0 of 1 with params {"window": 10, "point_method": "median", "distance_metric": "mse", "k": 10, "datepart_method": "expanded_binarized", "independent": false} and transformations {"fillna": "fake_date", "transformations": {"0": "MinMaxScaler"}, "transformation_params": {"0": {}}}
Model Number: 44 with model AverageValueNaive in generation 0 of 1 with params {"method": "Mean", "window": 420} and transformations {"fillna": "ffill", "transformations": {"0": "ClipOutliers", "1": "Detrend"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 1, "fillna": null}, "1": {"model": "GLS", "phi": 1, "window": null, "transform_dict": {"fillna": null, "transformations": {"0": "ClipOutliers"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 4}}}}}}
Model Number: 45 with model LastValueNaive in generation 0 of 1 with params {} and transformations {"fillna": "ffill", "transformations": {"0": "AlignLastValue", "1": "DifferencedTransformer", "2": "MinMaxScaler"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "multiplicative", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "max"}, "1": {"lag": 1, "fill": "bfill"}, "2": {}}}
Model Number: 46 with model GLS in generation 0 of 1 with params {} and transformations {"fillna": "fake_date", "transformations": {"0": "LevelShiftTransformer", "1": "FFTDecomposition", "2": "AlignLastValue", "3": "PositiveShift"}, "transformation_params": {"0": {"window_size": 30, "alpha": 3.5, "grouping_forward_limit": 3, "max_level_shifts": 10, "alignment": "average"}, "1": {"n_harmonics": null, "detrend": null}, "2": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 10, "threshold_method": "max"}, "3": {}}}
Model Number: 47 with model AverageValueNaive in generation 0 of 1 with params {"method": "Weighted_Mean", "window": null} and transformations {"fillna": "ffill_mean_biased", "transformations": {"0": "QuantileTransformer", "1": "SeasonalDifference", "2": "cffilter", "3": "AlignLastValue", "4": "PositiveShift"}, "transformation_params": {"0": {"output_distribution": "uniform", "n_quantiles": 1000}, "1": {"lag_1": 7, "method": "Mean"}, "2": {}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "max"}, "4": {}}}
Model Number: 48 with model SeasonalityMotif in generation 0 of 1 with params {"window": 7, "point_method": "median", "distance_metric": "minkowski", "k": 10, "datepart_method": "recurring", "independent": false} and transformations {"fillna": "ffill_mean_biased", "transformations": {"0": "ClipOutliers"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 3, "fillna": null}}}
Model Number: 49 with model SeasonalityMotif in generation 0 of 1 with params {"window": 10, "point_method": "weighted_mean", "distance_metric": "chebyshev", "k": 10, "datepart_method": "expanded_binarized", "independent": false} and transformations {"fillna": "median", "transformations": {"0": "Round", "1": "SeasonalDifference"}, "transformation_params": {"0": {"decimals": -2, "on_transform": true, "on_inverse": true}, "1": {"lag_1": 7, "method": "LastValue"}}}
Model Number: 50 with model ConstantNaive in generation 0 of 1 with params {"constant": 0} and transformations {"fillna": "cubic", "transformations": {"0": "StandardScaler", "1": "DifferencedTransformer", "2": "SinTrend", "3": "AlignLastValue"}, "transformation_params": {"0": {}, "1": {"lag": 1, "fill": "zero"}, "2": {}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "mean"}}}
Model Number: 51 with model LastValueNaive in generation 0 of 1 with params {} and transformations {"fillna": "rolling_mean", "transformations": {"0": "SinTrend", "1": "StandardScaler", "2": "Discretize"}, "transformation_params": {"0": {}, "1": {}, "2": {"discretization": "center", "n_bins": 20}}}
Model Number: 52 with model ConstantNaive in generation 0 of 1 with params {"constant": 0.1} and transformations {"fillna": "mean", "transformations": {"0": "ClipOutliers", "1": "Detrend", "2": "SeasonalDifference", "3": "AlignLastValue"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 2, "fillna": null}, "1": {"model": "GLS", "phi": 1, "window": null, "transform_dict": null}, "2": {"lag_1": 12, "method": "Median"}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}}}
Model Number: 53 with model GLS in generation 0 of 1 with params {} and transformations {"fillna": "ffill", "transformations": {"0": "QuantileTransformer", "1": "EWMAFilter", "2": "MinMaxScaler", "3": "convolution_filter"}, "transformation_params": {"0": {"output_distribution": "normal", "n_quantiles": "quarter"}, "1": {"span": 3}, "2": {}, "3": {}}}
Model Number: 54 with model SeasonalNaive in generation 0 of 1 with params {"method": "mean", "lag_1": 96, "lag_2": 364} and transformations {"fillna": "ffill", "transformations": {"0": "KalmanSmoothing"}, "transformation_params": {"0": {"model_name": "factor", "state_transition": [[1, 1, 0, 0, 0, 0], [0, 1, 0, 0, 0, 0], [0, 0, 1, 0, 0, 0], [0, 0, 0, 1, 1, 0], [0, 0, 0, 0, 1, 0], [0, 0, 0, 0, 0, 1]], "process_noise": [[1, 0, 0, 0, 0, 0], [0, 1, 0, 0, 0, 0], [0, 0, 0, 0, 0, 0], [0, 0, 1, 0, 0, 0], [0, 0, 0, 1, 0, 0], [0, 0, 0, 0, 0, 0]], "observation_model": [[1, 0, 0, 0, 0, 0]], "observation_noise": 0.04, "em_iter": null, "on_transform": false, "on_inverse": true}}}
New Generation: 1 of 1
Model Number: 55 with model ConstantNaive in generation 1 of 1 with params {"constant": 0.1} and transformations {"fillna": "KNNImputer", "transformations": {"0": "Slice", "1": "StandardScaler", "2": "DifferencedTransformer"}, "transformation_params": {"0": {"method": 0.5}, "1": {}, "2": {"lag": 1, "fill": "bfill"}}}
Model Number: 56 with model AverageValueNaive in generation 1 of 1 with params {"method": "Median", "window": null} and transformations {"fillna": "mean", "transformations": {"0": "ClipOutliers", "1": "QuantileTransformer"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 3, "fillna": null}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}}}
Model Number: 57 with model LastValueNaive in generation 1 of 1 with params {} and transformations {"fillna": "zero", "transformations": {"0": "PowerTransformer", "1": "AlignLastValue"}, "transformation_params": {"0": {}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "max"}}}
Model Number: 58 with model GLS in generation 1 of 1 with params {} and transformations {"fillna": "KNNImputer", "transformations": {"0": "Slice", "1": "DifferencedTransformer", "2": "Detrend", "3": "Slice"}, "transformation_params": {"0": {"method": 0.5}, "1": {}, "2": {"model": "Linear"}, "3": {"method": 100}}}
Model Number: 59 with model ConstantNaive in generation 1 of 1 with params {"constant": 0} and transformations {"fillna": "KNNImputer", "transformations": {"0": "Slice", "1": "HolidayTransformer"}, "transformation_params": {"0": {"method": 0.5}, "1": {"threshold": 0.8, "splash_threshold": null, "use_dayofmonth_holidays": false, "use_wkdom_holidays": true, "use_wkdeom_holidays": false, "use_lunar_holidays": false, "use_lunar_weekday": false, "use_islamic_holidays": false, "use_hebrew_holidays": false, "anomaly_detector_params": {"method": "zscore", "method_params": {"distribution": "norm", "alpha": 0.05}, "fillna": "ffill", "transform_dict": {"transformations": {"0": "DatepartRegression"}, "transformation_params": {"0": {"datepart_method": "simple_3", "regression_model": {"model": "DecisionTree", "model_params": {"max_depth": null, "min_samples_split": 0.1}}}}}, "isolated_only": false}, "remove_excess_anomalies": true, "impact": "regression", "regression_params": {}}}}
Model Number: 60 with model SectionalMotif in generation 1 of 1 with params {"window": 10, "point_method": "weighted_mean", "distance_metric": "sokalmichener", "include_differenced": true, "k": 5, "stride_size": 1, "regression_type": null} and transformations {"fillna": "time", "transformations": {"0": "LevelShiftTransformer"}, "transformation_params": {"0": {"window_size": 30, "alpha": 2.5, "grouping_forward_limit": 2, "max_level_shifts": 30, "alignment": "average"}}}
Model Number: 61 with model GLS in generation 1 of 1 with params {} and transformations {"fillna": "KNNImputer", "transformations": {"0": "Slice", "1": "AlignLastValue", "2": "AlignLastValue", "3": "Log"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}, "2": {"rows": 1, "lag": 2, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "mean"}, "3": {}}}
Model Number: 62 with model SeasonalNaive in generation 1 of 1 with params {"method": "lastvalue", "lag_1": 24, "lag_2": 1} and transformations {"fillna": "ffill", "transformations": {"0": "SeasonalDifference", "1": "MaxAbsScaler"}, "transformation_params": {"0": {"lag_1": 7, "method": "LastValue"}, "1": {}}}
Model Number: 63 with model LastValueNaive in generation 1 of 1 with params {} and transformations {"fillna": "ffill", "transformations": {"0": "PowerTransformer", "1": "RegressionFilter", "2": "FFTFilter", "3": "HolidayTransformer"}, "transformation_params": {"0": {}, "1": {"sigma": 0.5, "rolling_window": 90, "run_order": "season_first", "regression_params": {"regression_model": {"model": "DecisionTree", "model_params": {"max_depth": 3, "min_samples_split": 2}}, "datepart_method": "common_fourier", "polynomial_degree": null, "transform_dict": null, "holiday_countries_used": false, "lags": null, "forward_lags": 4}, "holiday_params": null, "trend_method": "local_linear"}, "2": {"cutoff": 0.2, "reverse": false}, "3": {"threshold": 0.8, "splash_threshold": null, "use_dayofmonth_holidays": true, "use_wkdom_holidays": true, "use_wkdeom_holidays": false, "use_lunar_holidays": false, "use_lunar_weekday": false, "use_islamic_holidays": false, "use_hebrew_holidays": false, "anomaly_detector_params": {"method": "zscore", "method_params": {"distribution": "chi2", "alpha": 0.03}, "fillna": "linear", "transform_dict": {"transformations": {"0": "DatepartRegression"}, "transformation_params": {"0": {"datepart_method": "simple_3", "regression_model": {"model": "ElasticNet", "model_params": {}}}}}, "isolated_only": false}, "remove_excess_anomalies": true, "impact": null, "regression_params": {}}}}
Model Number: 64 with model SectionalMotif in generation 1 of 1 with params {"window": 15, "point_method": "median", "distance_metric": "rogerstanimoto", "include_differenced": true, "k": 5, "stride_size": 1, "regression_type": null} and transformations {"fillna": "ffill", "transformations": {"0": "MaxAbsScaler"}, "transformation_params": {"0": {}}}
Model Number: 65 with model SeasonalityMotif in generation 1 of 1 with params {"window": 5, "point_method": "weighted_mean", "distance_metric": "mae", "k": 10, "datepart_method": "common_fourier", "independent": false} and transformations {"fillna": "nearest", "transformations": {"0": "AlignLastValue", "1": "RollingMean100thN"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "multiplicative", "strength": 1.0, "first_value_only": false}, "1": {}}}
Model Number: 66 with model SectionalMotif in generation 1 of 1 with params {"window": 5, "point_method": "midhinge", "distance_metric": "sokalmichener", "include_differenced": true, "k": 20, "stride_size": 1, "regression_type": null} and transformations {"fillna": "KNNImputer", "transformations": {"0": "DifferencedTransformer", "1": "AlignLastValue", "2": "DifferencedTransformer"}, "transformation_params": {"0": {"lag": 1, "fill": "bfill"}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}, "2": {"lag": 1, "fill": "zero"}}}
Model Number: 67 with model AverageValueNaive in generation 1 of 1 with params {"method": "Weighted_Mean", "window": 168} and transformations {"fillna": "KNNImputer", "transformations": {"0": "RobustScaler", "1": "AlignLastValue"}, "transformation_params": {"0": {}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}
Model Number: 68 with model AverageValueNaive in generation 1 of 1 with params {"method": "Mean", "window": 420} and transformations {"fillna": "KNNImputer", "transformations": {"0": "Slice", "1": "Detrend"}, "transformation_params": {"0": {"method": 0.5}, "1": {"model": "GLS", "phi": 1, "window": null, "transform_dict": {"fillna": null, "transformations": {"0": "ClipOutliers"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 4}}}}}}
Model Number: 69 with model LastValueNaive in generation 1 of 1 with params {} and transformations {"fillna": "ffill", "transformations": {"0": "LevelShiftTransformer", "1": "HistoricValues", "2": "AlignLastValue"}, "transformation_params": {"0": {"window_size": 90, "alpha": 3.0, "grouping_forward_limit": 3, "max_level_shifts": 5, "alignment": "rolling_diff"}, "1": {"window": null}, "2": {"rows": 1, "lag": 1, "method": "multiplicative", "strength": 0.7, "first_value_only": false, "threshold": 1, "threshold_method": "max"}}}
Model Number: 70 with model AverageValueNaive in generation 1 of 1 with params {"method": "Median", "window": null} and transformations {"fillna": "ffill_mean_biased", "transformations": {"0": "Slice", "1": "Round"}, "transformation_params": {"0": {"method": 0.5}, "1": {"decimals": 0, "on_transform": false, "on_inverse": true}}}
Model Number: 71 with model SeasonalNaive in generation 1 of 1 with params {"method": "lastvalue", "lag_1": 24, "lag_2": 1} and transformations {"fillna": "ffill_mean_biased", "transformations": {"0": "bkfilter"}, "transformation_params": {"0": {}}}
Model Number: 72 with model LastValueNaive in generation 1 of 1 with params {} and transformations {"fillna": "rolling_mean", "transformations": {"0": "STLFilter", "1": "StandardScaler", "2": "CumSumTransformer", "3": "AlignLastValue"}, "transformation_params": {"0": {"decomp_type": "STL", "part": "trend", "seasonal": 419}, "1": {}, "2": {}, "3": {"rows": 1, "lag": 1, "method": "multiplicative", "strength": 0.7, "first_value_only": false, "threshold": 10, "threshold_method": "max"}}}
Model Number: 73 with model SeasonalNaive in generation 1 of 1 with params {"method": "lastvalue", "lag_1": 28, "lag_2": null} and transformations {"fillna": "ffill", "transformations": {"0": "SeasonalDifference", "1": "AlignLastValue"}, "transformation_params": {"0": {"lag_1": 7, "method": "LastValue"}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}
Model Number: 74 with model AverageValueNaive in generation 1 of 1 with params {"method": "Mean", "window": 288} and transformations {"fillna": "KNNImputer", "transformations": {"0": "Slice", "1": "AlignLastValue", "2": "AlignLastValue"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}, "2": {"rows": 1, "lag": 7, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 3, "threshold_method": "max"}}}
Model Number: 75 with model LastValueNaive in generation 1 of 1 with params {} and transformations {"fillna": "mean", "transformations": {"0": "ClipOutliers", "1": "QuantileTransformer", "2": "FFTFilter"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 1, "fillna": null}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {"cutoff": 0.2, "reverse": false}}}
Model Number: 76 with model SeasonalNaive in generation 1 of 1 with params {"method": "lastvalue", "lag_1": 24, "lag_2": 28} and transformations {"fillna": "ffill", "transformations": {"0": "CumSumTransformer", "1": "MaxAbsScaler", "2": "Round"}, "transformation_params": {"0": {}, "1": {}, "2": {"decimals": 0, "on_transform": false, "on_inverse": true}}}
Model Number: 77 with model GLS in generation 1 of 1 with params {} and transformations {"fillna": "ffill", "transformations": {"0": "ClipOutliers", "1": "EWMAFilter", "2": "MinMaxScaler", "3": "convolution_filter"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 3, "fillna": null}, "1": {"span": 3}, "2": {}, "3": {}}}
Model Number: 78 with model SeasonalityMotif in generation 1 of 1 with params {"window": 5, "point_method": "median", "distance_metric": "mse", "k": 10, "datepart_method": "expanded_binarized", "independent": false} and transformations {"fillna": "ffill", "transformations": {"0": "CumSumTransformer", "1": "BTCD"}, "transformation_params": {"0": {}, "1": {"regression_model": {"model": "LinearRegression", "model_params": {}}, "max_lags": 2}}}
Model Number: 79 with model SeasonalityMotif in generation 1 of 1 with params {"window": 5, "point_method": "midhinge", "distance_metric": "chebyshev", "k": 1, "datepart_method": "recurring", "independent": false} and transformations {"fillna": "ffill", "transformations": {"0": "AlignLastValue", "1": "PowerTransformer", "2": "KalmanSmoothing"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "multiplicative", "strength": 1.0, "first_value_only": false}, "1": {}, "2": {"model_name": "randomly generated", "state_transition": [[-0.02929955845993884, 2.148619816617664, -0.05360999973416989, -0.9728962841739216, -0.3092267307228574, 0.04999054450405363, -0.20633594415327872, -0.1298918390362648], [0.143389116968792, -0.008660204883548853, 0.7741889150837097, 0.11646025187443146, -0.08533964428619355, 0.3515432098624288, -0.03489754708616902, -0.2576815297035731], [0.04086415197457365, -0.7350949266135237, 0.08431880418071928, -0.004984759273971567, 1.3271863359213116, -0.06827634967445095, 0.1483608056757098, 0.19176315672833988], [0.02987485210439378, -0.060249331002909176, 1.5261272262323158, -0.007652564956539951, -0.33174826060558255, 0.4390914942929803, -0.12132132675115952, -2.1427463093127534], [0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0], [-1.7703521002850093, -0.19273050122744415, -0.6216247128171034, -0.44827102007145014, -0.6223974342221994, 0.8903939353597601, -0.22639153876446505, -0.4764137774775346], [-0.7006712076001631, 0.8918683614671437, 0.3643658544667383, 0.0027715841441287165, 1.7372372347622766, -0.28325392205995276, 0.5850182707504655, 1.0014178174384727], [0.02090696518866513, -0.3004419637138319, -0.6400620608542399, 0.0036366406243988486, 0.23502004355381725, -0.042429041867674856, 0.26030579379154467, 0.10622747432879442]], "process_noise": [[0.007, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0], [0.0, 0.001, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0], [0.0, 0.0, 0.002, 0.0, 0.0, 0.0, 0.0, 0.0], [0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0], [0.0, 0.0, 0.0, 0.0, 0.016, 0.0, 0.0, 0.0], [0.0, 0.0, 0.0, 0.0, 0.0, 0.003, 0.0, 0.0], [0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.004, 0.0], [0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.026]], "observation_model": [[0.37642553115562943, -1.0994007905841945, 0.298238174206056, 1.3263858966870303, -0.6945678597313655, -0.14963454032767076, -0.43515355172163744, 1.8492637284793418]], "observation_noise": 1.218796812081237, "em_iter": 10, "on_transform": true, "on_inverse": false}}}
Model Number: 80 with model AverageValueNaive in generation 1 of 1 with params {"method": "Mean", "window": 420} and transformations {"fillna": "KNNImputer", "transformations": {"0": "Slice", "1": "AlignLastValue"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}
Model Number: 81 with model LastValueNaive in generation 1 of 1 with params {} and transformations {"fillna": "quadratic", "transformations": {"0": "DifferencedTransformer"}, "transformation_params": {"0": {"lag": 1, "fill": "zero"}}}
Model Number: 82 with model SeasonalNaive in generation 1 of 1 with params {"method": "lastvalue", "lag_1": 28, "lag_2": null} and transformations {"fillna": "ffill", "transformations": {"0": "Slice", "1": "AlignLastValue"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}
Model Number: 83 with model SectionalMotif in generation 1 of 1 with params {"window": 10, "point_method": "weighted_mean", "distance_metric": "sokalmichener", "include_differenced": true, "k": 10, "stride_size": 1, "regression_type": null} and transformations {"fillna": "zero", "transformations": {"0": "AlignLastValue"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}
Model Number: 84 with model SectionalMotif in generation 1 of 1 with params {"window": 10, "point_method": "median", "distance_metric": "canberra", "include_differenced": true, "k": 100, "stride_size": 1, "regression_type": null} and transformations {"fillna": "KNNImputer", "transformations": {"0": "SeasonalDifference", "1": "AlignLastValue", "2": "SeasonalDifference"}, "transformation_params": {"0": {"lag_1": 7, "method": "Median"}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}, "2": {"lag_1": 12, "method": 5}}}
Model Number: 85 with model SectionalMotif in generation 1 of 1 with params {"window": 10, "point_method": "mean", "distance_metric": "jaccard", "include_differenced": true, "k": 10, "stride_size": 1, "regression_type": "User"} and transformations {"fillna": "pchip", "transformations": {"0": "Slice", "1": "AlignLastValue"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}
Template Eval Error: Traceback (most recent call last):
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 1646, in TemplateWizard
df_forecast = model_forecast(
^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 1493, in model_forecast
model = model.fit(df_train_low, future_regressor_train)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 868, in fit
self.model = self.model.fit(
^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/models/basics.py", line 1828, in fit
raise ValueError(
ValueError: regression_type=='User' but no future_regressor supplied
in model 85 in generation 1: SectionalMotif
Model Number: 86 with model AverageValueNaive in generation 1 of 1 with params {"method": "Weighted_Mean", "window": 12} and transformations {"fillna": "quadratic", "transformations": {"0": "Slice", "1": "AlignLastValue"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}
Model Number: 87 with model AverageValueNaive in generation 1 of 1 with params {"method": "Median", "window": null} and transformations {"fillna": "fake_date", "transformations": {"0": "DifferencedTransformer", "1": "AlignLastValue"}, "transformation_params": {"0": {}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}
Model Number: 88 with model ConstantNaive in generation 1 of 1 with params {"constant": -1} and transformations {"fillna": "KNNImputer", "transformations": {"0": "AlignLastValue", "1": "AlignLastValue", "2": "DifferencedTransformer"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "max"}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}, "2": {"lag": 1, "fill": "bfill"}}}
Model Number: 89 with model ConstantNaive in generation 1 of 1 with params {"constant": 0} and transformations {"fillna": "KNNImputer", "transformations": {"0": "ClipOutliers", "1": "Detrend", "2": "SeasonalDifference", "3": "AlignLastValue"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 2, "fillna": null}, "1": {"model": "GLS", "phi": 1, "window": null, "transform_dict": null}, "2": {"lag_1": 12, "method": "Median"}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}}}
TotalRuntime missing in 2!
Validation Round: 1
Validation train index is DatetimeIndex(['2020-02-23', '2020-02-24', '2020-02-25', '2020-02-26',
'2020-02-27', '2020-02-28', '2020-02-29', '2020-03-01',
'2020-03-02', '2020-03-03',
...
'2023-02-28', '2023-03-01', '2023-03-02', '2023-03-03',
'2023-03-04', '2023-03-05', '2023-03-06', '2023-03-07',
'2023-03-08', '2023-03-09'],
dtype='datetime64[ns]', name='Date', length=1111, freq=None)
Model Number: 1 of 14 with model AverageValueNaive for Validation 1 with params {"method": "Weighted_Mean", "window": 168} and transformations {"fillna": "KNNImputer", "transformations": {"0": "RobustScaler", "1": "AlignLastValue"}, "transformation_params": {"0": {}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}
📈 1 - AverageValueNaive with avg smape 61.34:
Model Number: 2 of 14 with model AverageValueNaive for Validation 1 with params {"method": "Weighted_Mean", "window": 2} and transformations {"fillna": "KNNImputer", "transformations": {"0": "Slice", "1": "AlignLastValue"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}
2 - AverageValueNaive with avg smape 61.53:
Model Number: 3 of 14 with model AverageValueNaive for Validation 1 with params {"method": "Mean", "window": 420} and transformations {"fillna": "KNNImputer", "transformations": {"0": "Slice", "1": "AlignLastValue"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}
📈 3 - AverageValueNaive with avg smape 60.88:
Model Number: 4 of 14 with model LastValueNaive for Validation 1 with params {} and transformations {"fillna": "mean", "transformations": {"0": "ClipOutliers", "1": "QuantileTransformer"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 1, "fillna": null}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}}}
4 - LastValueNaive with avg smape 61.38:
Model Number: 5 of 14 with model LastValueNaive for Validation 1 with params {} and transformations {"fillna": "zero", "transformations": {"0": "PowerTransformer", "1": "AlignLastValue"}, "transformation_params": {"0": {}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "max"}}}
5 - LastValueNaive with avg smape 61.53:
Model Number: 6 of 14 with model ConstantNaive for Validation 1 with params {"constant": 0.1} and transformations {"fillna": "mean", "transformations": {"0": "ClipOutliers", "1": "Detrend", "2": "SeasonalDifference", "3": "AlignLastValue"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 2, "fillna": null}, "1": {"model": "GLS", "phi": 1, "window": null, "transform_dict": null}, "2": {"lag_1": 12, "method": "Median"}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}}}
6 - ConstantNaive with avg smape 61.82:
Model Number: 7 of 14 with model ConstantNaive for Validation 1 with params {"constant": 0} and transformations {"fillna": "KNNImputer", "transformations": {"0": "ClipOutliers", "1": "Detrend", "2": "SeasonalDifference", "3": "AlignLastValue"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 2, "fillna": null}, "1": {"model": "GLS", "phi": 1, "window": null, "transform_dict": null}, "2": {"lag_1": 12, "method": "Median"}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}}}
7 - ConstantNaive with avg smape 61.82:
Model Number: 8 of 14 with model SeasonalityMotif for Validation 1 with params {"window": 5, "point_method": "midhinge", "distance_metric": "mae", "k": 1, "datepart_method": "recurring", "independent": false} and transformations {"fillna": "ffill", "transformations": {"0": "AlignLastValue", "1": "HPFilter"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 10, "threshold_method": "max"}, "1": {"part": "trend", "lamb": 1600}}}
📈 8 - SeasonalityMotif with avg smape 58.14:
Model Number: 9 of 14 with model GLS for Validation 1 with params {} and transformations {"fillna": "KNNImputer", "transformations": {"0": "Slice", "1": "AlignLastValue", "2": "AlignLastValue", "3": "Log"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}, "2": {"rows": 1, "lag": 2, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "mean"}, "3": {}}}
9 - GLS with avg smape 61.54:
Model Number: 10 of 14 with model GLS for Validation 1 with params {} and transformations {"fillna": "akima", "transformations": {"0": "AlignLastValue", "1": "AnomalyRemoval", "2": "Discretize", "3": "Discretize", "4": "DifferencedTransformer"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}, "1": {"method": "IQR", "method_params": {"iqr_threshold": 3.0, "iqr_quantiles": [0.25, 0.75]}, "fillna": "ffill", "transform_dict": null, "isolated_only": false}, "2": {"discretization": "upper", "n_bins": 5}, "3": {"discretization": "center", "n_bins": 5}, "4": {"lag": 1, "fill": "zero"}}}
10 - GLS with avg smape 60.85:
Model Number: 11 of 14 with model SectionalMotif for Validation 1 with params {"window": 15, "point_method": "median", "distance_metric": "rogerstanimoto", "include_differenced": true, "k": 5, "stride_size": 1, "regression_type": null} and transformations {"fillna": "ffill", "transformations": {"0": "MaxAbsScaler"}, "transformation_params": {"0": {}}}
11 - SectionalMotif with avg smape 80.47:
Model Number: 12 of 14 with model GLS for Validation 1 with params {} and transformations {"fillna": "KNNImputer", "transformations": {"0": "Slice", "1": "DifferencedTransformer", "2": "Detrend", "3": "Slice"}, "transformation_params": {"0": {"method": 0.5}, "1": {}, "2": {"model": "Linear"}, "3": {"method": 100}}}
12 - GLS with avg smape 63.7:
Model Number: 13 of 14 with model SeasonalNaive for Validation 1 with params {"method": "median", "lag_1": 24, "lag_2": 28} and transformations {"fillna": "ffill_mean_biased", "transformations": {"0": "AlignLastValue"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 10, "threshold_method": "max"}}}
13 - SeasonalNaive with avg smape 60.17:
Model Number: 14 of 14 with model LastValueNaive for Validation 1 with params {} and transformations {"fillna": "rolling_mean_24", "transformations": {"0": "EWMAFilter", "1": "MinMaxScaler", "2": "LevelShiftTransformer", "3": "AlignLastValue"}, "transformation_params": {"0": {"span": 3}, "1": {}, "2": {"window_size": 90, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 30, "alignment": "last_value"}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "max"}}}
14 - LastValueNaive with avg smape 61.53:
Validation Round: 2
Validation train index is DatetimeIndex(['2020-02-23', '2020-02-24', '2020-02-25', '2020-02-26',
'2020-02-27', '2020-02-28', '2020-02-29', '2020-03-01',
'2020-03-02', '2020-03-03',
...
'2023-01-29', '2023-01-30', '2023-01-31', '2023-02-01',
'2023-02-02', '2023-02-03', '2023-02-04', '2023-02-05',
'2023-02-06', '2023-02-07'],
dtype='datetime64[ns]', name='Date', length=1081, freq=None)
Model Number: 1 of 14 with model AverageValueNaive for Validation 2 with params {"method": "Weighted_Mean", "window": 168} and transformations {"fillna": "KNNImputer", "transformations": {"0": "RobustScaler", "1": "AlignLastValue"}, "transformation_params": {"0": {}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}
📈 1 - AverageValueNaive with avg smape 48.06:
Model Number: 2 of 14 with model AverageValueNaive for Validation 2 with params {"method": "Weighted_Mean", "window": 2} and transformations {"fillna": "KNNImputer", "transformations": {"0": "Slice", "1": "AlignLastValue"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}
📈 2 - AverageValueNaive with avg smape 47.97:
Model Number: 3 of 14 with model AverageValueNaive for Validation 2 with params {"method": "Mean", "window": 420} and transformations {"fillna": "KNNImputer", "transformations": {"0": "Slice", "1": "AlignLastValue"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}
3 - AverageValueNaive with avg smape 48.05:
Model Number: 4 of 14 with model LastValueNaive for Validation 2 with params {} and transformations {"fillna": "mean", "transformations": {"0": "ClipOutliers", "1": "QuantileTransformer"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 1, "fillna": null}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}}}
4 - LastValueNaive with avg smape 48.77:
Model Number: 5 of 14 with model LastValueNaive for Validation 2 with params {} and transformations {"fillna": "zero", "transformations": {"0": "PowerTransformer", "1": "AlignLastValue"}, "transformation_params": {"0": {}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "max"}}}
5 - LastValueNaive with avg smape 47.97:
Model Number: 6 of 14 with model ConstantNaive for Validation 2 with params {"constant": 0.1} and transformations {"fillna": "mean", "transformations": {"0": "ClipOutliers", "1": "Detrend", "2": "SeasonalDifference", "3": "AlignLastValue"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 2, "fillna": null}, "1": {"model": "GLS", "phi": 1, "window": null, "transform_dict": null}, "2": {"lag_1": 12, "method": "Median"}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}}}
6 - ConstantNaive with avg smape 54.85:
Model Number: 7 of 14 with model ConstantNaive for Validation 2 with params {"constant": 0} and transformations {"fillna": "KNNImputer", "transformations": {"0": "ClipOutliers", "1": "Detrend", "2": "SeasonalDifference", "3": "AlignLastValue"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 2, "fillna": null}, "1": {"model": "GLS", "phi": 1, "window": null, "transform_dict": null}, "2": {"lag_1": 12, "method": "Median"}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}}}
7 - ConstantNaive with avg smape 54.85:
Model Number: 8 of 14 with model SeasonalityMotif for Validation 2 with params {"window": 5, "point_method": "midhinge", "distance_metric": "mae", "k": 1, "datepart_method": "recurring", "independent": false} and transformations {"fillna": "ffill", "transformations": {"0": "AlignLastValue", "1": "HPFilter"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 10, "threshold_method": "max"}, "1": {"part": "trend", "lamb": 1600}}}
8 - SeasonalityMotif with avg smape 57.31:
Model Number: 9 of 14 with model GLS for Validation 2 with params {} and transformations {"fillna": "KNNImputer", "transformations": {"0": "Slice", "1": "AlignLastValue", "2": "AlignLastValue", "3": "Log"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}, "2": {"rows": 1, "lag": 2, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "mean"}, "3": {}}}
📈 9 - GLS with avg smape 47.94:
Model Number: 10 of 14 with model GLS for Validation 2 with params {} and transformations {"fillna": "akima", "transformations": {"0": "AlignLastValue", "1": "AnomalyRemoval", "2": "Discretize", "3": "Discretize", "4": "DifferencedTransformer"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}, "1": {"method": "IQR", "method_params": {"iqr_threshold": 3.0, "iqr_quantiles": [0.25, 0.75]}, "fillna": "ffill", "transform_dict": null, "isolated_only": false}, "2": {"discretization": "upper", "n_bins": 5}, "3": {"discretization": "center", "n_bins": 5}, "4": {"lag": 1, "fill": "zero"}}}
10 - GLS with avg smape 50.42:
Model Number: 11 of 14 with model SectionalMotif for Validation 2 with params {"window": 15, "point_method": "median", "distance_metric": "rogerstanimoto", "include_differenced": true, "k": 5, "stride_size": 1, "regression_type": null} and transformations {"fillna": "ffill", "transformations": {"0": "MaxAbsScaler"}, "transformation_params": {"0": {}}}
11 - SectionalMotif with avg smape 77.44:
Model Number: 12 of 14 with model GLS for Validation 2 with params {} and transformations {"fillna": "KNNImputer", "transformations": {"0": "Slice", "1": "DifferencedTransformer", "2": "Detrend", "3": "Slice"}, "transformation_params": {"0": {"method": 0.5}, "1": {}, "2": {"model": "Linear"}, "3": {"method": 100}}}
12 - GLS with avg smape 49.7:
Model Number: 13 of 14 with model SeasonalNaive for Validation 2 with params {"method": "median", "lag_1": 24, "lag_2": 28} and transformations {"fillna": "ffill_mean_biased", "transformations": {"0": "AlignLastValue"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 10, "threshold_method": "max"}}}
13 - SeasonalNaive with avg smape 52.53:
Model Number: 14 of 14 with model LastValueNaive for Validation 2 with params {} and transformations {"fillna": "rolling_mean_24", "transformations": {"0": "EWMAFilter", "1": "MinMaxScaler", "2": "LevelShiftTransformer", "3": "AlignLastValue"}, "transformation_params": {"0": {"span": 3}, "1": {}, "2": {"window_size": 90, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 30, "alignment": "last_value"}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "max"}}}
14 - LastValueNaive with avg smape 47.97:
TotalRuntime missing in 3!
Validation Round: 1
Validation train index is DatetimeIndex(['2020-02-23', '2020-02-24', '2020-02-25', '2020-02-26',
'2020-02-27', '2020-02-28', '2020-02-29', '2020-03-01',
'2020-03-02', '2020-03-03',
...
'2023-02-28', '2023-03-01', '2023-03-02', '2023-03-03',
'2023-03-04', '2023-03-05', '2023-03-06', '2023-03-07',
'2023-03-08', '2023-03-09'],
dtype='datetime64[ns]', name='Date', length=1111, freq=None)
TotalRuntime missing in 0!
Validation Round: 2
Validation train index is DatetimeIndex(['2020-02-23', '2020-02-24', '2020-02-25', '2020-02-26',
'2020-02-27', '2020-02-28', '2020-02-29', '2020-03-01',
'2020-03-02', '2020-03-03',
...
'2023-01-29', '2023-01-30', '2023-01-31', '2023-02-01',
'2023-02-02', '2023-02-03', '2023-02-04', '2023-02-05',
'2023-02-06', '2023-02-07'],
dtype='datetime64[ns]', name='Date', length=1081, freq=None)
TotalRuntime missing in 0!
Model Number: 1 with model Ensemble in generation 0 of Horizontal Ensembles with params {"model_name": "Horizontal", "model_count": 3, "model_metric": "Score", "models": {"7a14af550afa2194472cfc2e4e1440fb": {"Model": "LastValueNaive", "ModelParameters": "{}", "TransformationParameters": "{\"fillna\": \"mean\", \"transformations\": {\"0\": \"ClipOutliers\", \"1\": \"QuantileTransformer\"}, \"transformation_params\": {\"0\": {\"method\": \"clip\", \"std_threshold\": 1, \"fillna\": null}, \"1\": {\"output_distribution\": \"uniform\", \"n_quantiles\": 1000}}}"}, "32e35e1e5b4ef8e16aa3292c7d099f1f": {"Model": "GLS", "ModelParameters": "{}", "TransformationParameters": "{\"fillna\": \"KNNImputer\", \"transformations\": {\"0\": \"Slice\", \"1\": \"AlignLastValue\", \"2\": \"AlignLastValue\", \"3\": \"Log\"}, \"transformation_params\": {\"0\": {\"method\": 0.5}, \"1\": {\"rows\": 1, \"lag\": 1, \"method\": \"additive\", \"strength\": 0.9, \"first_value_only\": false, \"threshold\": 3, \"threshold_method\": \"mean\"}, \"2\": {\"rows\": 1, \"lag\": 2, \"method\": \"additive\", \"strength\": 1.0, \"first_value_only\": false, \"threshold\": null, \"threshold_method\": \"mean\"}, \"3\": {}}}"}, "9b3a64ae866754d1d22a6441d35bce73": {"Model": "AverageValueNaive", "ModelParameters": "{\"method\": \"Mean\", \"window\": 420}", "TransformationParameters": "{\"fillna\": \"KNNImputer\", \"transformations\": {\"0\": \"Slice\", \"1\": \"AlignLastValue\"}, \"transformation_params\": {\"0\": {\"method\": 0.5}, \"1\": {\"rows\": 1, \"lag\": 1, \"method\": \"additive\", \"strength\": 0.9, \"first_value_only\": false, \"threshold\": 3, \"threshold_method\": \"mean\"}}}"}}, "series": {"theta": "32e35e1e5b4ef8e16aa3292c7d099f1f", "kappa": "7a14af550afa2194472cfc2e4e1440fb", "rho": "9b3a64ae866754d1d22a6441d35bce73", "sigma": "9b3a64ae866754d1d22a6441d35bce73"}} and transformations {}
Ensemble Horizontal component 1 of 3 LastValueNaive started
Ensemble Horizontal component 2 of 3 GLS started
Ensemble Horizontal component 3 of 3 AverageValueNaive started
Ensemble Horizontal component 1 of 3 LastValueNaive started
Ensemble Horizontal component 2 of 3 GLS started
Ensemble Horizontal component 3 of 3 AverageValueNaive started
2024-10-03 at 13:26:39 | ERROR | validation of end failed
Check the predicted ODE parameter values.
[6]:
df = snr.append().summary()
df.loc[df["Start"] >= future_start_date]
2024-10-03 at 13:26:39 | ERROR | validation of end failed
2024-10-03 at 13:26:39 | ERROR | validation of end failed
[6]:
Start | End | Rt | theta | kappa | rho | sigma | alpha1 [-] | 1/alpha2 [day] | 1/beta [day] | 1/gamma [day] | ODE | tau | ||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Scenario | Phase | |||||||||||||
Predicted | 68th | 2023-05-09 | 2023-06-07 | 1.56 | 0.000773 | 0.000001 | 0.001171 | 0.000747 | 0.001 | 14311 | 14 | 22 | SIR-F Model | 24 |
Check the dynamics.
[7]:
snr.simulate(name="Predicted");
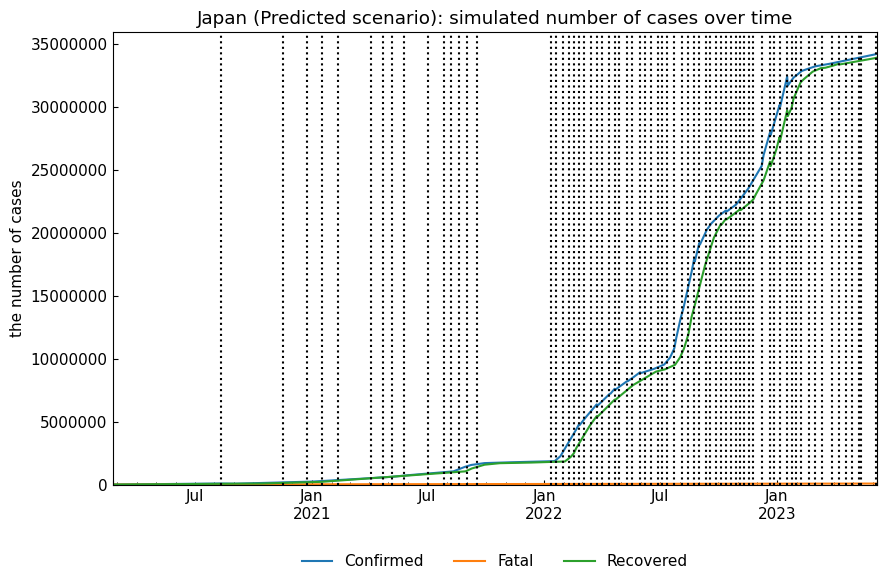
2. Time-series prediction with indicators
using `future_regressor
functionality of AutoTS <https://winedarksea.github.io/AutoTS/build/html/source/tutorial.html#adding-regressors-and-other-information>`__, we will predict ODE parameter values with indicators. We can download/create time-series data of indicators using DataEngineer
class as explained in Tutorial: Data preparation and Tutorial: Data
engineering.
[8]:
data_eng = cs.DataEngineer()
data_eng.download(databases=["japan", "covid19dh", "owid"]).clean().transform()
subset_df, *_ = data_eng.subset(geo="Japan")
indicator_df = subset_df.drop(["Population", "Susceptible", "Confirmed", "Infected", "Fatal", "Recovered"], axis=1)
indicator_df
[8]:
Cancel_events | Contact_tracing | Gatherings_restrictions | Information_campaigns | Internal_movement_restrictions | International_movement_restrictions | School_closing | Stay_home_restrictions | Stringency_index | Testing_policy | ... | Transport_closing | Vaccinated_full | Vaccinated_once | Vaccinations | Vaccinations_boosters | Workplace_closing | Country_0 | Country_Japan | Product_0 | Product_Moderna, Novavax, Oxford/AstraZeneca, Pfizer/BioNTech | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Date | |||||||||||||||||||||
2020-02-05 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | ... | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 1 | 0 | 1 | 0 |
2020-02-06 | 0.0 | 2.0 | 0.0 | 2.0 | 0.0 | 3.0 | 0.0 | 0.0 | 19.44 | 1.0 | ... | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0 | 1 | 1 | 0 |
2020-02-07 | 0.0 | 2.0 | 0.0 | 2.0 | 0.0 | 3.0 | 0.0 | 0.0 | 19.44 | 1.0 | ... | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0 | 1 | 1 | 0 |
2020-02-08 | 0.0 | 2.0 | 0.0 | 2.0 | 0.0 | 3.0 | 0.0 | 0.0 | 19.44 | 1.0 | ... | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0 | 1 | 1 | 0 |
2020-02-09 | 0.0 | 2.0 | 0.0 | 2.0 | 0.0 | 3.0 | 0.0 | 0.0 | 19.44 | 1.0 | ... | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0 | 1 | 1 | 0 |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
2023-05-04 | 1.0 | 1.0 | 1.0 | 1.0 | 1.0 | 1.0 | 1.0 | 1.0 | 33.33 | 1.0 | ... | 0.0 | 63889986991.0 | 67171434888.0 | 181897083005.0 | 50835661126.0 | 1.0 | 0 | 1 | 0 | 1 |
2023-05-05 | 1.0 | 1.0 | 1.0 | 1.0 | 1.0 | 1.0 | 1.0 | 1.0 | 33.33 | 1.0 | ... | 0.0 | 63993367323.0 | 67276140121.0 | 182280782416.0 | 51011274972.0 | 1.0 | 0 | 1 | 0 | 1 |
2023-05-06 | 1.0 | 1.0 | 1.0 | 1.0 | 1.0 | 1.0 | 1.0 | 1.0 | 33.33 | 1.0 | ... | 0.0 | 64096748099.0 | 67380845596.0 | 182664482513.0 | 51186888818.0 | 1.0 | 0 | 1 | 0 | 1 |
2023-05-07 | 1.0 | 1.0 | 1.0 | 1.0 | 1.0 | 1.0 | 1.0 | 1.0 | 33.33 | 1.0 | ... | 0.0 | 64200128969.0 | 67485551123.0 | 183048182756.0 | 51362502664.0 | 1.0 | 0 | 1 | 0 | 1 |
2023-05-08 | 1.0 | 1.0 | 1.0 | 1.0 | 1.0 | 1.0 | 1.0 | 1.0 | 33.33 | 1.0 | ... | 0.0 | 64303509961.0 | 67590256732.0 | 183431912857.0 | 51538146164.0 | 1.0 | 0 | 1 | 0 | 1 |
1189 rows × 21 columns
2.1 Principal Component Analysis
To remove multicollinearity of indicators, we use pca package: a python package to perform Principal Component Analysis and to create insightful plots via our MLEngineer(seed=0).pca(X, n_components)
. Standardization (Z-score normalization) and Principal Component Analysis (PCA) will be performed.
[9]:
ml = cs.MLEngineer()
pca_dict = ml.pca(X=indicator_df, n_components=0.95)
pca_df = pca_dict["PC"].copy()
pca_df.tail()
[9]:
PC1 | PC2 | PC3 | PC4 | PC5 | PC6 | PC7 | PC8 | PC9 | PC10 | |
---|---|---|---|---|---|---|---|---|---|---|
2023-05-04 | 5.673492 | -2.243826 | 0.555292 | -0.134307 | 0.888876 | 0.020971 | -0.364962 | -0.630145 | 0.039768 | -0.324168 |
2023-05-05 | 5.683267 | -2.247735 | 0.557146 | -0.134422 | 0.891652 | 0.019268 | -0.363681 | -0.632074 | 0.041499 | -0.325286 |
2023-05-06 | 5.693041 | -2.251644 | 0.558999 | -0.134538 | 0.894427 | 0.017564 | -0.362401 | -0.634004 | 0.043231 | -0.326404 |
2023-05-07 | 5.702815 | -2.255553 | 0.560853 | -0.134654 | 0.897203 | 0.015860 | -0.361120 | -0.635933 | 0.044962 | -0.327522 |
2023-05-08 | 5.712591 | -2.259462 | 0.562707 | -0.134770 | 0.899979 | 0.014157 | -0.359839 | -0.637862 | 0.046694 | -0.328641 |
The output of MLEngineer().pca()
is the model of pca package, we can show some figures easily as follows.
Explained variance:
[10]:
pca_dict["model"].plot()
plt.close()
Top features:
[11]:
df = pca_dict["topfeat"].copy()
df["PC"] = df["PC"].str.extract(r"(\d+)").astype(np.int64)
df = df.sort_values(["PC", "type"]).reset_index(drop=True)
def highlight(d):
styles = d.copy()
styles.loc[:, :] = ""
styles.loc[d["type"] == "best", :] = "background-color: yellow"
return styles
df.style.apply(highlight, axis=None)
[11]:
PC | feature | loading | type | |
---|---|---|---|---|
0 | 1 | Vaccinated_once | 0.347669 | best |
1 | 1 | Contact_tracing | -0.294071 | weak |
2 | 1 | Information_campaigns | -0.281398 | weak |
3 | 1 | Vaccinated_full | 0.346382 | weak |
4 | 1 | Vaccinations | 0.343559 | weak |
5 | 1 | Vaccinations_boosters | 0.308128 | weak |
6 | 2 | Stringency_index | 0.438532 | best |
7 | 2 | International_movement_restrictions | 0.313789 | weak |
8 | 2 | Product_0 | -0.318462 | weak |
9 | 2 | Product_Moderna, Novavax, Oxford/AstraZeneca, Pfizer/BioNTech | 0.318462 | weak |
10 | 3 | Country_Japan | 0.677881 | best |
11 | 3 | Country_0 | -0.677881 | weak |
12 | 4 | School_closing | 0.558851 | best |
13 | 5 | Transport_closing | 0.554676 | best |
14 | 6 | Workplace_closing | 0.650936 | best |
15 | 7 | Tests | 0.570970 | best |
16 | 8 | Cancel_events | 0.513032 | best |
17 | 9 | Testing_policy | 0.664159 | best |
18 | 10 | Gatherings_restrictions | 0.525608 | best |
19 | 10 | Internal_movement_restrictions | 0.331885 | weak |
20 | 10 | Stay_home_restrictions | -0.421741 | weak |
2-2. Future values of indicators
Before prediction of ODE parameter values, we need to prepare future values of (PCA-performed) indicators. We can add future values to the pandas.DataFrame
manually or forecast them with MLEngineer(seed=0).predict(Y, days=<int>, X=None)
as follows.
[12]:
future_df = ml.forecast(Y=pca_df, days=30, X=None)
future_df.tail()
Using 1 cpus for n_jobs.
Data frequency is: D, used frequency is: D
Model Number: 1 with model AverageValueNaive in generation 0 of 1 with params {"method": "Mean"} and transformations {"fillna": "fake_date", "transformations": {"0": "DifferencedTransformer", "1": "SinTrend"}, "transformation_params": {"0": {}, "1": {}}}
Model Number: 2 with model AverageValueNaive in generation 0 of 1 with params {"method": "Mean"} and transformations {"fillna": "mean", "transformations": {"0": "ClipOutliers", "1": "QuantileTransformer", "2": "DifferencedTransformer"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 3, "fillna": null}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {}}}
Model Number: 3 with model AverageValueNaive in generation 0 of 1 with params {"method": "Mean"} and transformations {"fillna": "rolling_mean_24", "transformations": {"0": "SeasonalDifference", "1": "Round", "2": "Detrend"}, "transformation_params": {"0": {"lag_1": 7, "method": "Mean"}, "1": {"model": "middle", "decimals": 2, "on_transform": true, "on_inverse": false}, "2": {"model": "GLS"}}}
Model Number: 4 with model GLS in generation 0 of 1 with params {} and transformations {"fillna": "rolling_mean", "transformations": {"0": "RollingMeanTransformer", "1": "DifferencedTransformer", "2": "Detrend", "3": "Slice"}, "transformation_params": {"0": {"fixed": true, "window": 3}, "1": {}, "2": {"model": "Linear"}, "3": {"method": 100}}}
Model Number: 5 with model GLS in generation 0 of 1 with params {} and transformations {"fillna": "median", "transformations": {"0": "ClipOutliers", "1": "QuantileTransformer", "2": "RobustScaler", "3": "Round", "4": "MaxAbsScaler"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 3.5, "fillna": null}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {}, "3": {"model": "middle", "decimals": 2, "on_transform": true, "on_inverse": true}, "4": {}}}
Model Number: 6 with model LastValueNaive in generation 0 of 1 with params {} and transformations {"fillna": "mean", "transformations": {"0": "bkfilter", "1": "SinTrend", "2": "Detrend", "3": "PowerTransformer"}, "transformation_params": {"0": {}, "1": {}, "2": {"model": "Linear"}, "3": {}}}
Model Number: 7 with model LastValueNaive in generation 0 of 1 with params {} and transformations {"fillna": "rolling_mean_24", "transformations": {"0": "PositiveShift", "1": "SinTrend", "2": "bkfilter"}, "transformation_params": {"0": {}, "1": {}, "2": {}}}
Model Number: 8 with model LastValueNaive in generation 0 of 1 with params {} and transformations {"fillna": "fake_date", "transformations": {"0": "SeasonalDifference", "1": "SinTrend"}, "transformation_params": {"0": {"lag_1": 7, "method": "LastValue"}, "1": {}}}
Model Number: 9 with model LastValueNaive in generation 0 of 1 with params {} and transformations {"fillna": "mean", "transformations": {"0": "ClipOutliers", "1": "QuantileTransformer"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 1, "fillna": null}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}}}
Model Number: 10 with model SeasonalNaive in generation 0 of 1 with params {"method": "LastValue", "lag_1": 2, "lag_2": 7} and transformations {"fillna": "rolling_mean_24", "transformations": {"0": "SinTrend", "1": "Round", "2": "PowerTransformer"}, "transformation_params": {"0": {}, "1": {"model": "middle", "decimals": 2, "on_transform": false, "on_inverse": true}, "2": {}}}
Model Number: 11 with model SeasonalNaive in generation 0 of 1 with params {"method": "LastValue", "lag_1": 2, "lag_2": 1} and transformations {"fillna": "rolling_mean_24", "transformations": {"0": "SeasonalDifference", "1": "QuantileTransformer", "2": "Detrend"}, "transformation_params": {"0": {"lag_1": 12, "method": "Median"}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {"model": "GLS"}}}
Model Number: 12 with model SeasonalNaive in generation 0 of 1 with params {"method": "LastValue", "lag_1": 7, "lag_2": 2} and transformations {"fillna": "mean", "transformations": {"0": "QuantileTransformer", "1": "ClipOutliers"}, "transformation_params": {"0": {"output_distribution": "uniform", "n_quantiles": 1000}, "1": {"method": "clip", "std_threshold": 2, "fillna": null}}}
Model Number: 13 with model ConstantNaive in generation 0 of 1 with params {} and transformations {"fillna": "ffill", "transformations": {"0": "PowerTransformer", "1": "QuantileTransformer", "2": "SeasonalDifference"}, "transformation_params": {"0": {}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {"lag_1": 7, "method": "LastValue"}}}
Model Number: 14 with model SeasonalNaive in generation 0 of 1 with params {"method": "lastvalue", "lag_1": 364, "lag_2": 28} and transformations {"fillna": "ffill", "transformations": {"0": "SeasonalDifference", "1": "MaxAbsScaler", "2": "Round"}, "transformation_params": {"0": {"lag_1": 7, "method": "LastValue"}, "1": {}, "2": {"decimals": 0, "on_transform": false, "on_inverse": true}}}
Model Number: 15 with model SectionalMotif in generation 0 of 1 with params {"window": 10, "point_method": "weighted_mean", "distance_metric": "sokalmichener", "include_differenced": true, "k": 20, "stride_size": 1, "regression_type": null} and transformations {"fillna": "zero", "transformations": {"0": null}, "transformation_params": {"0": {}}}
Model Number: 16 with model SectionalMotif in generation 0 of 1 with params {"window": 5, "point_method": "midhinge", "distance_metric": "canberra", "include_differenced": false, "k": 10, "stride_size": 1, "regression_type": null} and transformations {"fillna": "rolling_mean_24", "transformations": {"0": "QuantileTransformer", "1": "QuantileTransformer", "2": "RobustScaler"}, "transformation_params": {"0": {"output_distribution": "uniform", "n_quantiles": 1000}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {}}}
Model Number: 17 with model SeasonalNaive in generation 0 of 1 with params {"method": "lastvalue", "lag_1": 364, "lag_2": 30} and transformations {"fillna": "fake_date", "transformations": {"0": "SeasonalDifference", "1": "MaxAbsScaler", "2": "Round"}, "transformation_params": {"0": {"lag_1": 7, "method": "LastValue"}, "1": {}, "2": {"decimals": 0, "on_transform": false, "on_inverse": true}}}
Model Number: 18 with model SeasonalityMotif in generation 0 of 1 with params {"window": 5, "point_method": "weighted_mean", "distance_metric": "mae", "k": 10, "datepart_method": "common_fourier"} and transformations {"fillna": "nearest", "transformations": {"0": "AlignLastValue"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "multiplicative", "strength": 1.0, "first_value_only": false}}}
Model Number: 19 with model SectionalMotif in generation 0 of 1 with params {"window": 7, "point_method": "median", "distance_metric": "canberra", "include_differenced": true, "k": 5, "stride_size": 1, "regression_type": null} and transformations {"fillna": "ffill", "transformations": {"0": "SeasonalDifference", "1": "AlignLastValue", "2": "SeasonalDifference", "3": "LevelShiftTransformer"}, "transformation_params": {"0": {"lag_1": 7, "method": "Median"}, "1": {"rows": 1, "lag": 2, "method": "additive", "strength": 1.0, "first_value_only": false}, "2": {"lag_1": 12, "method": 5}, "3": {"window_size": 30, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 10, "alignment": "average"}}}
Model Number: 20 with model ConstantNaive in generation 0 of 1 with params {"constant": 1} and transformations {"fillna": "zero", "transformations": {"0": "ClipOutliers", "1": "Detrend", "2": "AnomalyRemoval"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 3, "fillna": null}, "1": {"model": "GLS", "phi": 1, "window": 30, "transform_dict": null}, "2": {"method": "zscore", "method_params": {"distribution": "chi2", "alpha": 0.1}, "fillna": "fake_date", "transform_dict": {"fillna": "quadratic", "transformations": {"0": "AlignLastValue"}, "transformation_params": {"0": {"rows": 4, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}, "isolated_only": false}}}
Model Number: 21 with model LastValueNaive in generation 0 of 1 with params {} and transformations {"fillna": "nearest", "transformations": {"0": "LevelShiftTransformer", "1": "MaxAbsScaler", "2": "AnomalyRemoval", "3": "AlignLastValue", "4": "AlignLastValue"}, "transformation_params": {"0": {"window_size": 90, "alpha": 3.0, "grouping_forward_limit": 3, "max_level_shifts": 5, "alignment": "rolling_diff"}, "1": {}, "2": {"method": "IQR", "method_params": {"iqr_threshold": 1.5, "iqr_quantiles": [0.4, 0.6]}, "fillna": "linear", "transform_dict": null, "isolated_only": true}, "3": {"rows": 1, "lag": 1, "method": "multiplicative", "strength": 0.7, "first_value_only": false, "threshold": 1, "threshold_method": "max"}, "4": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.5, "first_value_only": false, "threshold": 10, "threshold_method": "mean"}}}
Model Number: 22 with model AverageValueNaive in generation 0 of 1 with params {"method": "Median", "window": null} and transformations {"fillna": "ffill", "transformations": {"0": "Detrend", "1": "Detrend"}, "transformation_params": {"0": {"model": "Linear", "phi": 1, "window": null, "transform_dict": null}, "1": {"model": "Linear", "phi": 1, "window": null, "transform_dict": {"fillna": null, "transformations": {"0": "ClipOutliers"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 4}}}}}}
Model Number: 23 with model GLS in generation 0 of 1 with params {} and transformations {"fillna": "akima", "transformations": {"0": "AlignLastValue", "1": "AnomalyRemoval", "2": "Discretize", "3": "Discretize", "4": "DifferencedTransformer"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}, "1": {"method": "IQR", "method_params": {"iqr_threshold": 3.0, "iqr_quantiles": [0.25, 0.75]}, "fillna": "ffill", "transform_dict": null, "isolated_only": false}, "2": {"discretization": "upper", "n_bins": 5}, "3": {"discretization": "center", "n_bins": 5}, "4": {"lag": 1, "fill": "zero"}}}
Model Number: 24 with model SeasonalNaive in generation 0 of 1 with params {"method": "lastvalue", "lag_1": 2, "lag_2": 60} and transformations {"fillna": "ffill", "transformations": {"0": "ClipOutliers", "1": "Detrend"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 4, "fillna": null}, "1": {"model": "GLS", "phi": 1, "window": null, "transform_dict": {"fillna": null, "transformations": {"0": "ClipOutliers"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 4}}}}}}
Model Number: 25 with model SeasonalityMotif in generation 0 of 1 with params {"window": 5, "point_method": "midhinge", "distance_metric": "mae", "k": 1, "datepart_method": "recurring", "independent": false} and transformations {"fillna": "ffill", "transformations": {"0": "AlignLastValue", "1": "HPFilter"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 10, "threshold_method": "max"}, "1": {"part": "trend", "lamb": 1600}}}
Model Number: 26 with model SectionalMotif in generation 0 of 1 with params {"window": 10, "point_method": "mean", "distance_metric": "matching", "include_differenced": true, "k": 10, "stride_size": 1, "regression_type": null} and transformations {"fillna": "pchip", "transformations": {"0": "PCA", "1": "AlignLastValue", "2": "DiffSmoother", "3": "QuantileTransformer", "4": "AlignLastValue"}, "transformation_params": {"0": {"whiten": false, "n_components": null}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}, "2": {"method": "rolling_zscore", "method_params": {"distribution": "gamma", "alpha": 0.1, "rolling_periods": 200, "center": true}, "transform_dict": null, "reverse_alignment": true, "isolated_only": true, "fillna": "linear"}, "3": {"output_distribution": "uniform", "n_quantiles": "quarter"}, "4": {"rows": 1, "lag": 1, "method": "multiplicative", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}}}
Model Number: 27 with model SeasonalNaive in generation 0 of 1 with params {"method": "median", "lag_1": 24, "lag_2": 28} and transformations {"fillna": "ffill_mean_biased", "transformations": {"0": "AlignLastValue"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 10, "threshold_method": "max"}}}
Model Number: 28 with model SectionalMotif in generation 0 of 1 with params {"window": 10, "point_method": "weighted_mean", "distance_metric": "chebyshev", "include_differenced": true, "k": 10, "stride_size": 1, "regression_type": null} and transformations {"fillna": "mean", "transformations": {"0": "ClipOutliers", "1": "Detrend", "2": "PowerTransformer", "3": "AlignLastValue"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 4, "fillna": null}, "1": {"model": "Linear", "phi": 0.999, "window": null, "transform_dict": null}, "2": {}, "3": {"rows": 1, "lag": 28, "method": "multiplicative", "strength": 0.9, "first_value_only": false, "threshold": null, "threshold_method": "mean"}}}
Model Number: 29 with model AverageValueNaive in generation 0 of 1 with params {"method": "Mean", "window": null} and transformations {"fillna": "quadratic", "transformations": {"0": "STLFilter"}, "transformation_params": {"0": {"decomp_type": "STL", "part": "trend", "seasonal": 7}}}
Model Number: 30 with model LastValueNaive in generation 0 of 1 with params {} and transformations {"fillna": "rolling_mean_24", "transformations": {"0": "EWMAFilter", "1": "MinMaxScaler", "2": "LevelShiftTransformer", "3": "AlignLastValue"}, "transformation_params": {"0": {"span": 3}, "1": {}, "2": {"window_size": 90, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 30, "alignment": "last_value"}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "max"}}}
Model Number: 31 with model AverageValueNaive in generation 0 of 1 with params {"method": "Weighted_Mean", "window": 168} and transformations {"fillna": "quadratic", "transformations": {"0": "AlignLastValue", "1": "LevelShiftTransformer", "2": "AlignLastValue", "3": "SeasonalDifference"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}, "1": {"window_size": 90, "alpha": 2.5, "grouping_forward_limit": 4, "max_level_shifts": 5, "alignment": "average"}, "2": {"rows": 1, "lag": 28, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "max"}, "3": {"lag_1": 7, "method": "LastValue"}}}
Model Number: 32 with model AverageValueNaive in generation 0 of 1 with params {"method": "Exp_Weighted_Mean", "window": null} and transformations {"fillna": "nearest", "transformations": {"0": null}, "transformation_params": {"0": {}}}
Model Number: 33 with model SectionalMotif in generation 0 of 1 with params {"window": 50, "point_method": "weighted_mean", "distance_metric": "russellrao", "include_differenced": true, "k": 10, "stride_size": 1, "regression_type": null} and transformations {"fillna": "ffill", "transformations": {"0": "AlignLastValue", "1": "RegressionFilter", "2": "LevelShiftTransformer"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "multiplicative", "strength": 1.0, "first_value_only": true, "threshold": null, "threshold_method": "mean"}, "1": {"sigma": 3, "rolling_window": 90, "run_order": "season_first", "regression_params": {"regression_model": {"model": "ElasticNet", "model_params": {"l1_ratio": 0.5, "fit_intercept": true, "selection": "cyclic"}}, "datepart_method": "recurring", "polynomial_degree": null, "transform_dict": {"fillna": null, "transformations": {"0": "EWMAFilter"}, "transformation_params": {"0": {"span": 7}}}, "holiday_countries_used": false, "lags": null, "forward_lags": null}, "holiday_params": null, "trend_method": "rolling_mean"}, "2": {"window_size": 90, "alpha": 2.5, "grouping_forward_limit": 3, "max_level_shifts": 5, "alignment": "average"}}}
Model Number: 34 with model SectionalMotif in generation 0 of 1 with params {"window": 10, "point_method": "median", "distance_metric": "cityblock", "include_differenced": true, "k": 10, "stride_size": 1, "regression_type": "User"} and transformations {"fillna": "ffill_mean_biased", "transformations": {"0": "EWMAFilter", "1": "StandardScaler"}, "transformation_params": {"0": {"span": 12}, "1": {}}}
Template Eval Error: Traceback (most recent call last):
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 1646, in TemplateWizard
df_forecast = model_forecast(
^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 1493, in model_forecast
model = model.fit(df_train_low, future_regressor_train)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 868, in fit
self.model = self.model.fit(
^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/models/basics.py", line 1828, in fit
raise ValueError(
ValueError: regression_type=='User' but no future_regressor supplied
in model 34 in generation 0: SectionalMotif
Model Number: 35 with model AverageValueNaive in generation 0 of 1 with params {"method": "Weighted_Mean", "window": 2} and transformations {"fillna": "KNNImputer", "transformations": {"0": "Slice", "1": "AlignLastValue"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}
Model Number: 36 with model GLS in generation 0 of 1 with params {} and transformations {"fillna": "linear", "transformations": {"0": "ClipOutliers", "1": "Detrend", "2": "PowerTransformer", "3": "AlignLastValue"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 3, "fillna": null}, "1": {"model": "Linear", "phi": 1, "window": null, "transform_dict": {"fillna": null, "transformations": {"0": "EWMAFilter"}, "transformation_params": {"0": {"span": 2}}}}, "2": {}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.7, "first_value_only": true, "threshold": null, "threshold_method": "max"}}}
Model Number: 37 with model ConstantNaive in generation 0 of 1 with params {"constant": 1} and transformations {"fillna": "fake_date", "transformations": {"0": "AlignLastValue", "1": "KalmanSmoothing"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": true, "threshold": null, "threshold_method": "mean"}, "1": {"model_name": "spline", "state_transition": [[2, -1], [1, 0]], "process_noise": [[1, 0], [0, 0]], "observation_model": [[1, 0]], "observation_noise": 0.1, "em_iter": null, "on_transform": true, "on_inverse": false}}}
Model Number: 38 with model GLS in generation 0 of 1 with params {} and transformations {"fillna": "fake_date", "transformations": {"0": "HolidayTransformer", "1": "FFTFilter", "2": "CumSumTransformer", "3": "SeasonalDifference"}, "transformation_params": {"0": {"threshold": 0.8, "splash_threshold": null, "use_dayofmonth_holidays": true, "use_wkdom_holidays": true, "use_wkdeom_holidays": false, "use_lunar_holidays": false, "use_lunar_weekday": false, "use_islamic_holidays": true, "use_hebrew_holidays": false, "anomaly_detector_params": {"method": "mad", "method_params": {"distribution": "uniform", "alpha": 0.05}, "fillna": "ffill", "transform_dict": {"transformations": {"0": "DatepartRegression"}, "transformation_params": {"0": {"datepart_method": "simple_3", "regression_model": {"model": "FastRidge", "model_params": {}}}}}, "isolated_only": false}, "remove_excess_anomalies": true, "impact": "regression", "regression_params": {}}, "1": {"cutoff": 0.1, "reverse": false}, "2": {}, "3": {"lag_1": 7, "method": "Mean"}}}
Model Number: 39 with model ConstantNaive in generation 0 of 1 with params {"constant": 0} and transformations {"fillna": "ffill", "transformations": {"0": "StandardScaler", "1": "Round", "2": "IntermittentOccurrence"}, "transformation_params": {"0": {}, "1": {"decimals": 1, "on_transform": true, "on_inverse": false}, "2": {"center": "mean"}}}
Model Number: 40 with model GLS in generation 0 of 1 with params {} and transformations {"fillna": "cubic", "transformations": {"0": "ClipOutliers", "1": "Detrend", "2": "DiffSmoother", "3": "AlignLastValue"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 3, "fillna": null}, "1": {"model": "Linear", "phi": 1, "window": 900, "transform_dict": null}, "2": {"method": null, "method_params": null, "transform_dict": null, "reverse_alignment": true, "isolated_only": false, "fillna": 1.0}, "3": {"rows": 7, "lag": 28, "method": "additive", "strength": 0.7, "first_value_only": false, "threshold": null, "threshold_method": "max"}}}
/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/sklearn/linear_model/_ridge.py:216: LinAlgWarning: Ill-conditioned matrix (rcond=9.20734e-26): result may not be accurate.
return linalg.solve(A, Xy, assume_a="pos", overwrite_a=True).T
Model Number: 41 with model AverageValueNaive in generation 0 of 1 with params {"method": "Mean", "window": null} and transformations {"fillna": "akima", "transformations": {"0": "LevelShiftTransformer"}, "transformation_params": {"0": {"window_size": 364, "alpha": 3.0, "grouping_forward_limit": 4, "max_level_shifts": 5, "alignment": "average"}}}
Model Number: 42 with model SeasonalityMotif in generation 0 of 1 with params {"window": 10, "point_method": "median", "distance_metric": "minkowski", "k": 20, "datepart_method": "simple_2", "independent": false} and transformations {"fillna": "time", "transformations": {"0": "bkfilter", "1": "MaxAbsScaler", "2": "StandardScaler", "3": "QuantileTransformer", "4": "cffilter"}, "transformation_params": {"0": {}, "1": {}, "2": {}, "3": {"output_distribution": "uniform", "n_quantiles": 20}, "4": {}}}
Model Number: 43 with model SeasonalityMotif in generation 0 of 1 with params {"window": 10, "point_method": "median", "distance_metric": "mse", "k": 10, "datepart_method": "expanded_binarized", "independent": false} and transformations {"fillna": "fake_date", "transformations": {"0": "MinMaxScaler"}, "transformation_params": {"0": {}}}
Model Number: 44 with model AverageValueNaive in generation 0 of 1 with params {"method": "Mean", "window": 420} and transformations {"fillna": "ffill", "transformations": {"0": "ClipOutliers", "1": "Detrend"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 1, "fillna": null}, "1": {"model": "GLS", "phi": 1, "window": null, "transform_dict": {"fillna": null, "transformations": {"0": "ClipOutliers"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 4}}}}}}
Model Number: 45 with model LastValueNaive in generation 0 of 1 with params {} and transformations {"fillna": "ffill", "transformations": {"0": "AlignLastValue", "1": "DifferencedTransformer", "2": "MinMaxScaler"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "multiplicative", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "max"}, "1": {"lag": 1, "fill": "bfill"}, "2": {}}}
Model Number: 46 with model GLS in generation 0 of 1 with params {} and transformations {"fillna": "fake_date", "transformations": {"0": "LevelShiftTransformer", "1": "FFTDecomposition", "2": "AlignLastValue", "3": "PositiveShift"}, "transformation_params": {"0": {"window_size": 30, "alpha": 3.5, "grouping_forward_limit": 3, "max_level_shifts": 10, "alignment": "average"}, "1": {"n_harmonics": null, "detrend": null}, "2": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 10, "threshold_method": "max"}, "3": {}}}
Model Number: 47 with model AverageValueNaive in generation 0 of 1 with params {"method": "Weighted_Mean", "window": null} and transformations {"fillna": "ffill_mean_biased", "transformations": {"0": "QuantileTransformer", "1": "SeasonalDifference", "2": "cffilter", "3": "AlignLastValue", "4": "PositiveShift"}, "transformation_params": {"0": {"output_distribution": "uniform", "n_quantiles": 1000}, "1": {"lag_1": 7, "method": "Mean"}, "2": {}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "max"}, "4": {}}}
Model Number: 48 with model SeasonalityMotif in generation 0 of 1 with params {"window": 7, "point_method": "median", "distance_metric": "minkowski", "k": 10, "datepart_method": "recurring", "independent": false} and transformations {"fillna": "ffill_mean_biased", "transformations": {"0": "ClipOutliers"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 3, "fillna": null}}}
Model Number: 49 with model SeasonalityMotif in generation 0 of 1 with params {"window": 10, "point_method": "weighted_mean", "distance_metric": "chebyshev", "k": 10, "datepart_method": "expanded_binarized", "independent": false} and transformations {"fillna": "median", "transformations": {"0": "Round", "1": "SeasonalDifference"}, "transformation_params": {"0": {"decimals": -2, "on_transform": true, "on_inverse": true}, "1": {"lag_1": 7, "method": "LastValue"}}}
Model Number: 50 with model ConstantNaive in generation 0 of 1 with params {"constant": 0} and transformations {"fillna": "cubic", "transformations": {"0": "StandardScaler", "1": "DifferencedTransformer", "2": "SinTrend", "3": "AlignLastValue"}, "transformation_params": {"0": {}, "1": {"lag": 1, "fill": "zero"}, "2": {}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "mean"}}}
Model Number: 51 with model LastValueNaive in generation 0 of 1 with params {} and transformations {"fillna": "rolling_mean", "transformations": {"0": "SinTrend", "1": "StandardScaler", "2": "Discretize"}, "transformation_params": {"0": {}, "1": {}, "2": {"discretization": "center", "n_bins": 20}}}
Model Number: 52 with model ConstantNaive in generation 0 of 1 with params {"constant": 0.1} and transformations {"fillna": "mean", "transformations": {"0": "ClipOutliers", "1": "Detrend", "2": "SeasonalDifference", "3": "AlignLastValue"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 2, "fillna": null}, "1": {"model": "GLS", "phi": 1, "window": null, "transform_dict": null}, "2": {"lag_1": 12, "method": "Median"}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}}}
Model Number: 53 with model GLS in generation 0 of 1 with params {} and transformations {"fillna": "ffill", "transformations": {"0": "QuantileTransformer", "1": "EWMAFilter", "2": "MinMaxScaler", "3": "convolution_filter"}, "transformation_params": {"0": {"output_distribution": "normal", "n_quantiles": "quarter"}, "1": {"span": 3}, "2": {}, "3": {}}}
Model Number: 54 with model SeasonalNaive in generation 0 of 1 with params {"method": "mean", "lag_1": 96, "lag_2": 364} and transformations {"fillna": "ffill", "transformations": {"0": "KalmanSmoothing"}, "transformation_params": {"0": {"model_name": "factor", "state_transition": [[1, 1, 0, 0, 0, 0], [0, 1, 0, 0, 0, 0], [0, 0, 1, 0, 0, 0], [0, 0, 0, 1, 1, 0], [0, 0, 0, 0, 1, 0], [0, 0, 0, 0, 0, 1]], "process_noise": [[1, 0, 0, 0, 0, 0], [0, 1, 0, 0, 0, 0], [0, 0, 0, 0, 0, 0], [0, 0, 1, 0, 0, 0], [0, 0, 0, 1, 0, 0], [0, 0, 0, 0, 0, 0]], "observation_model": [[1, 0, 0, 0, 0, 0]], "observation_noise": 0.04, "em_iter": null, "on_transform": false, "on_inverse": true}}}
New Generation: 1 of 1
Model Number: 55 with model SeasonalNaive in generation 1 of 1 with params {"method": "median", "lag_1": 24, "lag_2": 28} and transformations {"fillna": "ffill_mean_biased", "transformations": {"0": "RobustScaler", "1": "AlignLastValue", "2": "Round", "3": "DifferencedTransformer", "4": "LevelShiftTransformer"}, "transformation_params": {"0": {}, "1": {"rows": 7, "lag": 1, "method": "multiplicative", "strength": 0.2, "first_value_only": false, "threshold": 10, "threshold_method": "max"}, "2": {"decimals": 0, "on_transform": true, "on_inverse": false}, "3": {"lag": 7, "fill": "zero"}, "4": {"window_size": 90, "alpha": 3.0, "grouping_forward_limit": 4, "max_level_shifts": 30, "alignment": "average"}}}
Template Eval Error: Traceback (most recent call last):
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 1646, in TemplateWizard
df_forecast = model_forecast(
^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 1494, in model_forecast
return model.predict(
^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 989, in predict
raise ValueError(
ValueError: Model returned NaN due to a preprocessing transformer {'fillna': 'ffill_mean_biased', 'transformations': {'0': 'RobustScaler', '1': 'AlignLastValue', '2': 'Round', '3': 'DifferencedTransformer', '4': 'LevelShiftTransformer'}, 'transformation_params': {'0': {}, '1': {'rows': 7, 'lag': 1, 'method': 'multiplicative', 'strength': 0.2, 'first_value_only': False, 'threshold': 10, 'threshold_method': 'max'}, '2': {'decimals': 0, 'on_transform': True, 'on_inverse': False}, '3': {'lag': 7, 'fill': 'zero'}, '4': {'window_size': 90, 'alpha': 3.0, 'grouping_forward_limit': 4, 'max_level_shifts': 30, 'alignment': 'average'}}}. fail_on_forecast_nan=True
in model 55 in generation 1: SeasonalNaive
Model Number: 56 with model GLS in generation 1 of 1 with params {} and transformations {"fillna": "median", "transformations": {"0": "KalmanSmoothing", "1": "DifferencedTransformer", "2": "Slice"}, "transformation_params": {"0": {"model_name": "randomly generated", "state_transition": [[0.0, 0.0, 0.0, 0.0], [-0.43251989572619787, 0.9474468384977176, 0.7862744604186882, -0.06785728666895087], [1.219121062175026, -0.9284460183917971, 0.29169810653560596, -0.017704099223315478], [5.582143100899549, -0.07465026707815797, -0.06818019429651916, 0.9994437258224352]], "process_noise": [[0.0, 0.0, 0.0, 0.0], [0.0, 0.01, 0.0, 0.0], [0.0, 0.0, 0.032, 0.0], [0.0, 0.0, 0.0, 0.011]], "observation_model": [[0.8152699081292198, 0.22909794833826744, -1.0261787778523443, 0.47752546922509714]], "observation_noise": 1.0080192359535038, "em_iter": 10, "on_transform": true, "on_inverse": false}, "1": {}, "2": {"method": 100}}}
Model Number: 57 with model ConstantNaive in generation 1 of 1 with params {"constant": 0} and transformations {"fillna": "linear", "transformations": {"0": "EWMAFilter", "1": "QuantileTransformer", "2": "StandardScaler", "3": "IntermittentOccurrence"}, "transformation_params": {"0": {"span": 12}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {}, "3": {"center": "mean"}}}
Model Number: 58 with model SectionalMotif in generation 1 of 1 with params {"window": 7, "point_method": "midhinge", "distance_metric": "cosine", "include_differenced": true, "k": 10, "stride_size": 1, "regression_type": null} and transformations {"fillna": "ffill", "transformations": {"0": "SeasonalDifference", "1": "AlignLastValue", "2": "Slice", "3": "LevelShiftTransformer"}, "transformation_params": {"0": {"lag_1": 7, "method": "Median"}, "1": {"rows": 1, "lag": 2, "method": "additive", "strength": 1.0, "first_value_only": false}, "2": {"method": 0.5}, "3": {"window_size": 30, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 10, "alignment": "average"}}}
Model Number: 59 with model SectionalMotif in generation 1 of 1 with params {"window": 7, "point_method": "median", "distance_metric": "sokalsneath", "include_differenced": true, "k": 5, "stride_size": 1, "regression_type": null} and transformations {"fillna": "ffill", "transformations": {"0": "SeasonalDifference", "1": "AlignLastValue", "2": "PowerTransformer", "3": "LevelShiftTransformer"}, "transformation_params": {"0": {"lag_1": 7, "method": "Median"}, "1": {"rows": 1, "lag": 2, "method": "additive", "strength": 1.0, "first_value_only": false}, "2": {}, "3": {"window_size": 30, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 10, "alignment": "average"}}}
Model Number: 60 with model GLS in generation 1 of 1 with params {} and transformations {"fillna": "ffill", "transformations": {"0": "MaxAbsScaler", "1": "cffilter", "2": "QuantileTransformer", "3": "DifferencedTransformer", "4": "KalmanSmoothing"}, "transformation_params": {"0": {}, "1": {}, "2": {"output_distribution": "uniform", "n_quantiles": 1000}, "3": {"lag": 1, "fill": "bfill"}, "4": {"model_name": "randomly generated_original", "state_transition": [[1, 1, 0, 0], [0, 1, 0, 0], [0, -1, 0, 0], [0, 0, 0, 0]], "process_noise": [[0.04, 0.0, 0.0, 0.0], [0.0, 0.0, 0.0, 0.0], [0.0, 0.0, 0.0, 0.0], [0.0, 0.0, 0.0, 0.0]], "observation_model": [[1, 1, 0, 0]], "observation_noise": 0.2, "em_iter": null, "on_transform": true, "on_inverse": false}}}
Model Number: 61 with model SeasonalityMotif in generation 1 of 1 with params {"window": 5, "point_method": "mean", "distance_metric": "mae", "k": 10, "datepart_method": "expanded_binarized", "independent": false} and transformations {"fillna": "mean", "transformations": {"0": "AlignLastValue", "1": "SeasonalDifference", "2": "LevelShiftTransformer"}, "transformation_params": {"0": {"rows": 1, "lag": 2, "method": "additive", "strength": 1.0, "first_value_only": false}, "1": {"lag_1": 12, "method": 5}, "2": {"window_size": 30, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 10, "alignment": "average"}}}
Model Number: 62 with model LastValueNaive in generation 1 of 1 with params {} and transformations {"fillna": "fake_date", "transformations": {"0": "BKBandpassFilter"}, "transformation_params": {"0": {"low": 6, "high": 364, "K": 6, "lanczos_factor": false, "return_diff": false, "on_transform": false, "on_inverse": true}}}
Template Eval Error: Traceback (most recent call last):
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 1646, in TemplateWizard
df_forecast = model_forecast(
^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 1494, in model_forecast
return model.predict(
^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 911, in predict
raise ValueError(
ValueError: Model LastValueNaive returned improper forecast_length. Returned: 18 and requested: 30
in model 62 in generation 1: LastValueNaive
Model Number: 63 with model AverageValueNaive in generation 1 of 1 with params {"method": "Mean", "window": 96} and transformations {"fillna": "quadratic", "transformations": {"0": "AlignLastValue", "1": "LevelShiftTransformer", "2": "AlignLastValue", "3": "SeasonalDifference"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}, "1": {"window_size": 90, "alpha": 2.5, "grouping_forward_limit": 4, "max_level_shifts": 5, "alignment": "average"}, "2": {"rows": 1, "lag": 28, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "max"}, "3": {"lag_1": 7, "method": "LastValue"}}}
Model Number: 64 with model SeasonalityMotif in generation 1 of 1 with params {"window": 7, "point_method": "weighted_mean", "distance_metric": "mae", "k": 10, "datepart_method": "expanded_binarized", "independent": false} and transformations {"fillna": "median", "transformations": {"0": "DifferencedTransformer", "1": "StandardScaler"}, "transformation_params": {"0": {"lag": 1, "fill": "bfill"}, "1": {}}}
Model Number: 65 with model AverageValueNaive in generation 1 of 1 with params {"method": "Mean", "window": null} and transformations {"fillna": "rolling_mean", "transformations": {"0": "ClipOutliers", "1": "AlignLastValue", "2": "LevelShiftTransformer"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 3.5, "fillna": null}, "1": {"rows": 1, "lag": 2, "method": "additive", "strength": 1.0, "first_value_only": false}, "2": {"window_size": 30, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 10, "alignment": "average"}}}
Model Number: 66 with model SectionalMotif in generation 1 of 1 with params {"window": 5, "point_method": "midhinge", "distance_metric": "canberra", "include_differenced": false, "k": 10, "stride_size": 1, "regression_type": null} and transformations {"fillna": "ffill", "transformations": {"0": "AlignLastValue", "1": "RegressionFilter", "2": "SeasonalDifference"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "multiplicative", "strength": 1.0, "first_value_only": true, "threshold": null, "threshold_method": "mean"}, "1": {"sigma": 3, "rolling_window": 90, "run_order": "season_first", "regression_params": {"regression_model": {"model": "ElasticNet", "model_params": {"l1_ratio": 0.5, "fit_intercept": true, "selection": "cyclic"}}, "datepart_method": "recurring", "polynomial_degree": null, "transform_dict": {"fillna": null, "transformations": {"0": "EWMAFilter"}, "transformation_params": {"0": {"span": 7}}}, "holiday_countries_used": false, "lags": null, "forward_lags": null}, "holiday_params": null, "trend_method": "rolling_mean"}, "2": {"lag_1": 12, "method": 5}}}
Model Number: 67 with model ConstantNaive in generation 1 of 1 with params {"constant": 0.1} and transformations {"fillna": "ffill", "transformations": {"0": "DifferencedTransformer", "1": "Detrend", "2": "LevelShiftTransformer"}, "transformation_params": {"0": {"lag": 1, "fill": "bfill"}, "1": {"model": "Linear", "phi": 1, "window": 900, "transform_dict": {"fillna": null, "transformations": {"0": "EWMAFilter"}, "transformation_params": {"0": {"span": 2}}}}, "2": {"window_size": 30, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 10, "alignment": "average"}}}
Model Number: 68 with model ConstantNaive in generation 1 of 1 with params {"constant": 0.1} and transformations {"fillna": "nearest", "transformations": {"0": "ClipOutliers", "1": "Detrend"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 2, "fillna": null}, "1": {"model": "GLS", "phi": 1, "window": null, "transform_dict": null}}}
Model Number: 69 with model GLS in generation 1 of 1 with params {} and transformations {"fillna": "akima", "transformations": {"0": "AlignLastValue", "1": "AnomalyRemoval", "2": "bkfilter", "3": "bkfilter", "4": "convolution_filter"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 10, "threshold_method": "mean"}, "1": {"method": "IQR", "method_params": {"iqr_threshold": 3.0, "iqr_quantiles": [0.25, 0.75]}, "fillna": "ffill", "transform_dict": null, "isolated_only": false}, "2": {}, "3": {}, "4": {}}}
Model Number: 70 with model SeasonalNaive in generation 1 of 1 with params {"method": "lastvalue", "lag_1": 28, "lag_2": 60} and transformations {"fillna": "time", "transformations": {"0": "StandardScaler", "1": "Detrend"}, "transformation_params": {"0": {}, "1": {"model": "GLS", "phi": 1, "window": null, "transform_dict": {"fillna": null, "transformations": {"0": "ClipOutliers"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 4}}}}}}
Model Number: 71 with model SectionalMotif in generation 1 of 1 with params {"window": 5, "point_method": "midhinge", "distance_metric": "canberra", "include_differenced": true, "k": 5, "stride_size": 1, "regression_type": null} and transformations {"fillna": "ffill", "transformations": {"0": "SeasonalDifference", "1": "AlignLastValue", "2": "SeasonalDifference", "3": "LevelShiftTransformer"}, "transformation_params": {"0": {"lag_1": 7, "method": "Median"}, "1": {"rows": 1, "lag": 2, "method": "additive", "strength": 1.0, "first_value_only": false}, "2": {"lag_1": 12, "method": 5}, "3": {"window_size": 30, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 10, "alignment": "average"}}}
Model Number: 72 with model AverageValueNaive in generation 1 of 1 with params {"method": "Median", "window": null} and transformations {"fillna": "ffill", "transformations": {"0": "bkfilter", "1": "QuantileTransformer", "2": "DifferencedTransformer", "3": "HistoricValues", "4": "CumSumTransformer"}, "transformation_params": {"0": {}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {}, "3": {"window": 10}, "4": {}}}
Model Number: 73 with model LastValueNaive in generation 1 of 1 with params {} and transformations {"fillna": "ffill", "transformations": {"0": "HistoricValues", "1": "AlignLastValue", "2": "SeasonalDifference", "3": "LevelShiftTransformer", "4": "SinTrend"}, "transformation_params": {"0": {"window": null}, "1": {"rows": 1, "lag": 2, "method": "additive", "strength": 1.0, "first_value_only": false}, "2": {"lag_1": 12, "method": 5}, "3": {"window_size": 30, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 10, "alignment": "average"}, "4": {}}}
Model Number: 74 with model LastValueNaive in generation 1 of 1 with params {} and transformations {"fillna": "time", "transformations": {"0": "LevelShiftTransformer", "1": "MaxAbsScaler", "2": "ClipOutliers", "3": "AlignLastValue", "4": "AlignLastValue"}, "transformation_params": {"0": {"window_size": 90, "alpha": 3.0, "grouping_forward_limit": 3, "max_level_shifts": 5, "alignment": "rolling_diff"}, "1": {}, "2": {"method": "clip", "std_threshold": 3, "fillna": null}, "3": {"rows": 1, "lag": 1, "method": "multiplicative", "strength": 0.7, "first_value_only": false, "threshold": 1, "threshold_method": "max"}, "4": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 10, "threshold_method": "max"}}}
Model Number: 75 with model SectionalMotif in generation 1 of 1 with params {"window": 5, "point_method": "weighted_mean", "distance_metric": "canberra", "include_differenced": true, "k": 10, "stride_size": 1, "regression_type": null} and transformations {"fillna": "ffill", "transformations": {"0": "SeasonalDifference", "1": "AlignLastValue", "2": "SeasonalDifference", "3": "LevelShiftTransformer"}, "transformation_params": {"0": {"lag_1": 7, "method": "Median"}, "1": {"rows": 1, "lag": 2, "method": "additive", "strength": 1.0, "first_value_only": false}, "2": {"lag_1": 12, "method": 5}, "3": {"window_size": 30, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 10, "alignment": "average"}}}
Model Number: 76 with model SeasonalityMotif in generation 1 of 1 with params {"window": 5, "point_method": "weighted_mean", "distance_metric": "canberra", "k": 1, "datepart_method": "common_fourier_rw", "independent": false} and transformations {"fillna": "median", "transformations": {"0": "bkfilter", "1": "MaxAbsScaler"}, "transformation_params": {"0": {}, "1": {}}}
Model Number: 77 with model ConstantNaive in generation 1 of 1 with params {"constant": 0} and transformations {"fillna": "nearest", "transformations": {"0": "ClipOutliers", "1": "Detrend", "2": "SeasonalDifference"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 2, "fillna": null}, "1": {"model": "GLS", "phi": 1, "window": null, "transform_dict": null}, "2": {"lag_1": 12, "method": "Median"}}}
Model Number: 78 with model ConstantNaive in generation 1 of 1 with params {"constant": 0} and transformations {"fillna": "ffill", "transformations": {"0": "SeasonalDifference", "1": "QuantileTransformer", "2": "SeasonalDifference"}, "transformation_params": {"0": {"lag_1": 7, "method": "Median"}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {"lag_1": 7, "method": "LastValue"}}}
Model Number: 79 with model SectionalMotif in generation 1 of 1 with params {"window": 7, "point_method": "median", "distance_metric": "canberra", "include_differenced": true, "k": 10, "stride_size": 1, "regression_type": "User"} and transformations {"fillna": "ffill", "transformations": {"0": "AlignLastDiff", "1": "DatepartRegression", "2": "SeasonalDifference"}, "transformation_params": {"0": {"rows": 364, "displacement_rows": 21, "quantile": 1.0, "decay_span": 90}, "1": {"regression_model": {"model": "ElasticNet", "model_params": {"l1_ratio": 0.5, "fit_intercept": true, "selection": "cyclic"}}, "datepart_method": "expanded", "polynomial_degree": null, "transform_dict": null, "holiday_countries_used": false, "lags": null, "forward_lags": null}, "2": {"lag_1": 12, "method": 5}}}
Template Eval Error: Traceback (most recent call last):
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 1646, in TemplateWizard
df_forecast = model_forecast(
^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 1493, in model_forecast
model = model.fit(df_train_low, future_regressor_train)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 868, in fit
self.model = self.model.fit(
^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/models/basics.py", line 1828, in fit
raise ValueError(
ValueError: regression_type=='User' but no future_regressor supplied
in model 79 in generation 1: SectionalMotif
Model Number: 80 with model LastValueNaive in generation 1 of 1 with params {} and transformations {"fillna": "KNNImputer", "transformations": {"0": "EWMAFilter", "1": "BKBandpassFilter", "2": "StandardScaler", "3": "AlignLastValue"}, "transformation_params": {"0": {"span": 3}, "1": {"low": 6, "high": 364, "K": 6, "lanczos_factor": false, "return_diff": false, "on_transform": false, "on_inverse": true}, "2": {}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "max"}}}
Template Eval Error: Traceback (most recent call last):
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 1646, in TemplateWizard
df_forecast = model_forecast(
^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 1494, in model_forecast
return model.predict(
^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 911, in predict
raise ValueError(
ValueError: Model LastValueNaive returned improper forecast_length. Returned: 18 and requested: 30
in model 80 in generation 1: LastValueNaive
Model Number: 81 with model LastValueNaive in generation 1 of 1 with params {} and transformations {"fillna": "ffill", "transformations": {"0": "SeasonalDifference", "1": "SinTrend", "2": "SeasonalDifference", "3": "LevelShiftTransformer"}, "transformation_params": {"0": {"lag_1": 7, "method": "Median"}, "1": {}, "2": {"lag_1": 12, "method": 5}, "3": {"window_size": 30, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 10, "alignment": "average"}}}
Model Number: 82 with model ConstantNaive in generation 1 of 1 with params {"constant": 0} and transformations {"fillna": "cubic", "transformations": {"0": "StandardScaler", "1": "DifferencedTransformer", "2": "DifferencedTransformer", "3": "AlignLastValue"}, "transformation_params": {"0": {}, "1": {"lag": 1, "fill": "zero"}, "2": {"lag": 1, "fill": "bfill"}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "mean"}}}
Model Number: 83 with model AverageValueNaive in generation 1 of 1 with params {"method": "Weighted_Mean", "window": null} and transformations {"fillna": "ffill", "transformations": {"0": "MaxAbsScaler", "1": "ClipOutliers", "2": "AlignLastDiff", "3": "StandardScaler", "4": "HistoricValues", "5": "CumSumTransformer"}, "transformation_params": {"0": {}, "1": {"method": "clip", "std_threshold": 4, "fillna": null}, "2": {"rows": 1, "displacement_rows": 1, "quantile": 1.0, "decay_span": null}, "3": {}, "4": {"window": 10}, "5": {}}}
Model Number: 84 with model SeasonalNaive in generation 1 of 1 with params {"method": "lastvalue", "lag_1": 2, "lag_2": 1} and transformations {"fillna": "ffill", "transformations": {"0": "ClipOutliers", "1": "Detrend", "2": "AlignLastValue"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 4, "fillna": null}, "1": {"model": "GLS", "phi": 1, "window": null, "transform_dict": {"fillna": null, "transformations": {"0": "ClipOutliers"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 4}}}}, "2": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 10, "threshold_method": "mean"}}}
Model Number: 85 with model AverageValueNaive in generation 1 of 1 with params {"method": "Weighted_Mean", "window": null} and transformations {"fillna": "quadratic", "transformations": {"0": "AlignLastValue", "1": "LevelShiftTransformer", "2": "AlignLastValue"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}, "1": {"window_size": 90, "alpha": 2.5, "grouping_forward_limit": 4, "max_level_shifts": 5, "alignment": "average"}, "2": {"rows": 1, "lag": 28, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "max"}}}
Model Number: 86 with model SeasonalNaive in generation 1 of 1 with params {"method": "mean", "lag_1": 2, "lag_2": 60} and transformations {"fillna": "ffill_mean_biased", "transformations": {"0": "AlignLastValue", "1": "DifferencedTransformer", "2": "SeasonalDifference", "3": "LevelShiftTransformer", "4": "AlignLastValue"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.7, "first_value_only": false, "threshold": null, "threshold_method": "mean"}, "1": {"lag": 1, "fill": "bfill"}, "2": {"lag_1": 12, "method": 5}, "3": {"window_size": 30, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 10, "alignment": "average"}, "4": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 10, "threshold_method": "mean"}}}
Model Number: 87 with model SeasonalNaive in generation 1 of 1 with params {"method": "lastvalue", "lag_1": 7, "lag_2": null} and transformations {"fillna": "cubic", "transformations": {"0": "SeasonalDifference", "1": "Log", "2": "StandardScaler"}, "transformation_params": {"0": {"lag_1": 7, "method": "Median"}, "1": {}, "2": {}}}
Model Number: 88 with model GLS in generation 1 of 1 with params {} and transformations {"fillna": "ffill", "transformations": {"0": "RollingMean100thN", "1": "Detrend", "2": "HPFilter", "3": "AlignLastValue", "4": "StandardScaler"}, "transformation_params": {"0": {}, "1": {"model": "GLS", "phi": 1, "window": null, "transform_dict": null}, "2": {"part": "trend", "lamb": 104976000000}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}, "4": {}}}
Model Number: 89 with model SeasonalNaive in generation 1 of 1 with params {"method": "lastvalue", "lag_1": 364, "lag_2": 28} and transformations {"fillna": "KNNImputer", "transformations": {"0": "RollingMeanTransformer", "1": "Slice", "2": "PositiveShift"}, "transformation_params": {"0": {"fixed": false, "window": 90, "macro_micro": false, "center": false}, "1": {"method": 0.5}, "2": {}}}
TotalRuntime missing in 2!
Validation Round: 1
Validation train index is DatetimeIndex(['2020-02-05', '2020-02-06', '2020-02-07', '2020-02-08',
'2020-02-09', '2020-02-10', '2020-02-11', '2020-02-12',
'2020-02-13', '2020-02-14',
...
'2023-02-28', '2023-03-01', '2023-03-02', '2023-03-03',
'2023-03-04', '2023-03-05', '2023-03-06', '2023-03-07',
'2023-03-08', '2023-03-09'],
dtype='datetime64[ns]', length=1129, freq=None)
Model Number: 1 of 16 with model ConstantNaive for Validation 1 with params {"constant": 0} and transformations {"fillna": "cubic", "transformations": {"0": "StandardScaler", "1": "DifferencedTransformer", "2": "DifferencedTransformer", "3": "AlignLastValue"}, "transformation_params": {"0": {}, "1": {"lag": 1, "fill": "zero"}, "2": {"lag": 1, "fill": "bfill"}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "mean"}}}
📈 1 - ConstantNaive with avg smape 120.0:
Model Number: 2 of 16 with model SeasonalityMotif for Validation 1 with params {"window": 7, "point_method": "weighted_mean", "distance_metric": "mae", "k": 10, "datepart_method": "expanded_binarized", "independent": false} and transformations {"fillna": "median", "transformations": {"0": "DifferencedTransformer", "1": "StandardScaler"}, "transformation_params": {"0": {"lag": 1, "fill": "bfill"}, "1": {}}}
2 - SeasonalityMotif with avg smape 120.01:
Model Number: 3 of 16 with model GLS for Validation 1 with params {} and transformations {"fillna": "median", "transformations": {"0": "KalmanSmoothing", "1": "DifferencedTransformer", "2": "Slice"}, "transformation_params": {"0": {"model_name": "randomly generated", "state_transition": [[0.0, 0.0, 0.0, 0.0], [-0.43251989572619787, 0.9474468384977176, 0.7862744604186882, -0.06785728666895087], [1.219121062175026, -0.9284460183917971, 0.29169810653560596, -0.017704099223315478], [5.582143100899549, -0.07465026707815797, -0.06818019429651916, 0.9994437258224352]], "process_noise": [[0.0, 0.0, 0.0, 0.0], [0.0, 0.01, 0.0, 0.0], [0.0, 0.0, 0.032, 0.0], [0.0, 0.0, 0.0, 0.011]], "observation_model": [[0.8152699081292198, 0.22909794833826744, -1.0261787778523443, 0.47752546922509714]], "observation_noise": 1.0080192359535038, "em_iter": 10, "on_transform": true, "on_inverse": false}, "1": {}, "2": {"method": 100}}}
3 - GLS with avg smape 130.47:
Model Number: 4 of 16 with model SectionalMotif for Validation 1 with params {"window": 7, "point_method": "midhinge", "distance_metric": "cosine", "include_differenced": true, "k": 10, "stride_size": 1, "regression_type": null} and transformations {"fillna": "ffill", "transformations": {"0": "SeasonalDifference", "1": "AlignLastValue", "2": "Slice", "3": "LevelShiftTransformer"}, "transformation_params": {"0": {"lag_1": 7, "method": "Median"}, "1": {"rows": 1, "lag": 2, "method": "additive", "strength": 1.0, "first_value_only": false}, "2": {"method": 0.5}, "3": {"window_size": 30, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 10, "alignment": "average"}}}
4 - SectionalMotif with avg smape 127.82:
Model Number: 5 of 16 with model SectionalMotif for Validation 1 with params {"window": 5, "point_method": "midhinge", "distance_metric": "canberra", "include_differenced": true, "k": 5, "stride_size": 1, "regression_type": null} and transformations {"fillna": "ffill", "transformations": {"0": "SeasonalDifference", "1": "AlignLastValue", "2": "SeasonalDifference", "3": "LevelShiftTransformer"}, "transformation_params": {"0": {"lag_1": 7, "method": "Median"}, "1": {"rows": 1, "lag": 2, "method": "additive", "strength": 1.0, "first_value_only": false}, "2": {"lag_1": 12, "method": 5}, "3": {"window_size": 30, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 10, "alignment": "average"}}}
5 - SectionalMotif with avg smape 143.5:
Model Number: 6 of 16 with model SeasonalityMotif for Validation 1 with params {"window": 5, "point_method": "mean", "distance_metric": "mae", "k": 10, "datepart_method": "expanded_binarized", "independent": false} and transformations {"fillna": "mean", "transformations": {"0": "AlignLastValue", "1": "SeasonalDifference", "2": "LevelShiftTransformer"}, "transformation_params": {"0": {"rows": 1, "lag": 2, "method": "additive", "strength": 1.0, "first_value_only": false}, "1": {"lag_1": 12, "method": 5}, "2": {"window_size": 30, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 10, "alignment": "average"}}}
6 - SeasonalityMotif with avg smape 122.91:
Model Number: 7 of 16 with model SectionalMotif for Validation 1 with params {"window": 7, "point_method": "median", "distance_metric": "canberra", "include_differenced": true, "k": 5, "stride_size": 1, "regression_type": null} and transformations {"fillna": "ffill", "transformations": {"0": "SeasonalDifference", "1": "AlignLastValue", "2": "SeasonalDifference", "3": "LevelShiftTransformer"}, "transformation_params": {"0": {"lag_1": 7, "method": "Median"}, "1": {"rows": 1, "lag": 2, "method": "additive", "strength": 1.0, "first_value_only": false}, "2": {"lag_1": 12, "method": 5}, "3": {"window_size": 30, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 10, "alignment": "average"}}}
7 - SectionalMotif with avg smape 143.43:
Model Number: 8 of 16 with model AverageValueNaive for Validation 1 with params {"method": "Median", "window": null} and transformations {"fillna": "ffill", "transformations": {"0": "bkfilter", "1": "QuantileTransformer", "2": "DifferencedTransformer", "3": "HistoricValues", "4": "CumSumTransformer"}, "transformation_params": {"0": {}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {}, "3": {"window": 10}, "4": {}}}
8 - AverageValueNaive with avg smape 124.15:
Model Number: 9 of 16 with model AverageValueNaive for Validation 1 with params {"method": "Weighted_Mean", "window": null} and transformations {"fillna": "ffill", "transformations": {"0": "MaxAbsScaler", "1": "ClipOutliers", "2": "AlignLastDiff", "3": "StandardScaler", "4": "HistoricValues", "5": "CumSumTransformer"}, "transformation_params": {"0": {}, "1": {"method": "clip", "std_threshold": 4, "fillna": null}, "2": {"rows": 1, "displacement_rows": 1, "quantile": 1.0, "decay_span": null}, "3": {}, "4": {"window": 10}, "5": {}}}
9 - AverageValueNaive with avg smape 123.89:
Model Number: 10 of 16 with model GLS for Validation 1 with params {} and transformations {"fillna": "rolling_mean", "transformations": {"0": "RollingMeanTransformer", "1": "DifferencedTransformer", "2": "Detrend", "3": "Slice"}, "transformation_params": {"0": {"fixed": true, "window": 3}, "1": {}, "2": {"model": "Linear"}, "3": {"method": 100}}}
10 - GLS with avg smape 139.78:
Model Number: 11 of 16 with model AverageValueNaive for Validation 1 with params {"method": "Mean", "window": 96} and transformations {"fillna": "quadratic", "transformations": {"0": "AlignLastValue", "1": "LevelShiftTransformer", "2": "AlignLastValue", "3": "SeasonalDifference"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}, "1": {"window_size": 90, "alpha": 2.5, "grouping_forward_limit": 4, "max_level_shifts": 5, "alignment": "average"}, "2": {"rows": 1, "lag": 28, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "max"}, "3": {"lag_1": 7, "method": "LastValue"}}}
11 - AverageValueNaive with avg smape 123.44:
Model Number: 12 of 16 with model LastValueNaive for Validation 1 with params {} and transformations {"fillna": "ffill", "transformations": {"0": "AlignLastValue", "1": "DifferencedTransformer", "2": "MinMaxScaler"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "multiplicative", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "max"}, "1": {"lag": 1, "fill": "bfill"}, "2": {}}}
12 - LastValueNaive with avg smape 120.25:
Model Number: 13 of 16 with model LastValueNaive for Validation 1 with params {} and transformations {"fillna": "rolling_mean_24", "transformations": {"0": "EWMAFilter", "1": "MinMaxScaler", "2": "LevelShiftTransformer", "3": "AlignLastValue"}, "transformation_params": {"0": {"span": 3}, "1": {}, "2": {"window_size": 90, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 30, "alignment": "last_value"}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "max"}}}
13 - LastValueNaive with avg smape 124.37:
Model Number: 14 of 16 with model SeasonalityMotif for Validation 1 with params {"window": 10, "point_method": "median", "distance_metric": "mse", "k": 10, "datepart_method": "expanded_binarized", "independent": false} and transformations {"fillna": "fake_date", "transformations": {"0": "MinMaxScaler"}, "transformation_params": {"0": {}}}
14 - SeasonalityMotif with avg smape 124.9:
Model Number: 15 of 16 with model SectionalMotif for Validation 1 with params {"window": 10, "point_method": "mean", "distance_metric": "matching", "include_differenced": true, "k": 10, "stride_size": 1, "regression_type": null} and transformations {"fillna": "pchip", "transformations": {"0": "PCA", "1": "AlignLastValue", "2": "DiffSmoother", "3": "QuantileTransformer", "4": "AlignLastValue"}, "transformation_params": {"0": {"whiten": false, "n_components": null}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}, "2": {"method": "rolling_zscore", "method_params": {"distribution": "gamma", "alpha": 0.1, "rolling_periods": 200, "center": true}, "transform_dict": null, "reverse_alignment": true, "isolated_only": true, "fillna": "linear"}, "3": {"output_distribution": "uniform", "n_quantiles": 289}, "4": {"rows": 1, "lag": 1, "method": "multiplicative", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}}}
15 - SectionalMotif with avg smape 141.72:
Model Number: 16 of 16 with model LastValueNaive for Validation 1 with params {} and transformations {"fillna": "ffill", "transformations": {"0": "HistoricValues", "1": "AlignLastValue", "2": "SeasonalDifference", "3": "LevelShiftTransformer", "4": "SinTrend"}, "transformation_params": {"0": {"window": null}, "1": {"rows": 1, "lag": 2, "method": "additive", "strength": 1.0, "first_value_only": false}, "2": {"lag_1": 12, "method": 5}, "3": {"window_size": 30, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 10, "alignment": "average"}, "4": {}}}
16 - LastValueNaive with avg smape 132.87:
Validation Round: 2
Validation train index is DatetimeIndex(['2020-02-05', '2020-02-06', '2020-02-07', '2020-02-08',
'2020-02-09', '2020-02-10', '2020-02-11', '2020-02-12',
'2020-02-13', '2020-02-14',
...
'2023-01-29', '2023-01-30', '2023-01-31', '2023-02-01',
'2023-02-02', '2023-02-03', '2023-02-04', '2023-02-05',
'2023-02-06', '2023-02-07'],
dtype='datetime64[ns]', length=1099, freq=None)
Model Number: 1 of 16 with model ConstantNaive for Validation 2 with params {"constant": 0} and transformations {"fillna": "cubic", "transformations": {"0": "StandardScaler", "1": "DifferencedTransformer", "2": "DifferencedTransformer", "3": "AlignLastValue"}, "transformation_params": {"0": {}, "1": {"lag": 1, "fill": "zero"}, "2": {"lag": 1, "fill": "bfill"}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "mean"}}}
📈 1 - ConstantNaive with avg smape 120.01:
Model Number: 2 of 16 with model SeasonalityMotif for Validation 2 with params {"window": 7, "point_method": "weighted_mean", "distance_metric": "mae", "k": 10, "datepart_method": "expanded_binarized", "independent": false} and transformations {"fillna": "median", "transformations": {"0": "DifferencedTransformer", "1": "StandardScaler"}, "transformation_params": {"0": {"lag": 1, "fill": "bfill"}, "1": {}}}
2 - SeasonalityMotif with avg smape 120.02:
Model Number: 3 of 16 with model GLS for Validation 2 with params {} and transformations {"fillna": "median", "transformations": {"0": "KalmanSmoothing", "1": "DifferencedTransformer", "2": "Slice"}, "transformation_params": {"0": {"model_name": "randomly generated", "state_transition": [[0.0, 0.0, 0.0, 0.0], [-0.43251989572619787, 0.9474468384977176, 0.7862744604186882, -0.06785728666895087], [1.219121062175026, -0.9284460183917971, 0.29169810653560596, -0.017704099223315478], [5.582143100899549, -0.07465026707815797, -0.06818019429651916, 0.9994437258224352]], "process_noise": [[0.0, 0.0, 0.0, 0.0], [0.0, 0.01, 0.0, 0.0], [0.0, 0.0, 0.032, 0.0], [0.0, 0.0, 0.0, 0.011]], "observation_model": [[0.8152699081292198, 0.22909794833826744, -1.0261787778523443, 0.47752546922509714]], "observation_noise": 1.0080192359535038, "em_iter": 10, "on_transform": true, "on_inverse": false}, "1": {}, "2": {"method": 100}}}
3 - GLS with avg smape 128.66:
Model Number: 4 of 16 with model SectionalMotif for Validation 2 with params {"window": 7, "point_method": "midhinge", "distance_metric": "cosine", "include_differenced": true, "k": 10, "stride_size": 1, "regression_type": null} and transformations {"fillna": "ffill", "transformations": {"0": "SeasonalDifference", "1": "AlignLastValue", "2": "Slice", "3": "LevelShiftTransformer"}, "transformation_params": {"0": {"lag_1": 7, "method": "Median"}, "1": {"rows": 1, "lag": 2, "method": "additive", "strength": 1.0, "first_value_only": false}, "2": {"method": 0.5}, "3": {"window_size": 30, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 10, "alignment": "average"}}}
4 - SectionalMotif with avg smape 125.02:
Model Number: 5 of 16 with model SectionalMotif for Validation 2 with params {"window": 5, "point_method": "midhinge", "distance_metric": "canberra", "include_differenced": true, "k": 5, "stride_size": 1, "regression_type": null} and transformations {"fillna": "ffill", "transformations": {"0": "SeasonalDifference", "1": "AlignLastValue", "2": "SeasonalDifference", "3": "LevelShiftTransformer"}, "transformation_params": {"0": {"lag_1": 7, "method": "Median"}, "1": {"rows": 1, "lag": 2, "method": "additive", "strength": 1.0, "first_value_only": false}, "2": {"lag_1": 12, "method": 5}, "3": {"window_size": 30, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 10, "alignment": "average"}}}
5 - SectionalMotif with avg smape 126.97:
Model Number: 6 of 16 with model SeasonalityMotif for Validation 2 with params {"window": 5, "point_method": "mean", "distance_metric": "mae", "k": 10, "datepart_method": "expanded_binarized", "independent": false} and transformations {"fillna": "mean", "transformations": {"0": "AlignLastValue", "1": "SeasonalDifference", "2": "LevelShiftTransformer"}, "transformation_params": {"0": {"rows": 1, "lag": 2, "method": "additive", "strength": 1.0, "first_value_only": false}, "1": {"lag_1": 12, "method": 5}, "2": {"window_size": 30, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 10, "alignment": "average"}}}
6 - SeasonalityMotif with avg smape 124.0:
Model Number: 7 of 16 with model SectionalMotif for Validation 2 with params {"window": 7, "point_method": "median", "distance_metric": "canberra", "include_differenced": true, "k": 5, "stride_size": 1, "regression_type": null} and transformations {"fillna": "ffill", "transformations": {"0": "SeasonalDifference", "1": "AlignLastValue", "2": "SeasonalDifference", "3": "LevelShiftTransformer"}, "transformation_params": {"0": {"lag_1": 7, "method": "Median"}, "1": {"rows": 1, "lag": 2, "method": "additive", "strength": 1.0, "first_value_only": false}, "2": {"lag_1": 12, "method": 5}, "3": {"window_size": 30, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 10, "alignment": "average"}}}
7 - SectionalMotif with avg smape 121.79:
Model Number: 8 of 16 with model AverageValueNaive for Validation 2 with params {"method": "Median", "window": null} and transformations {"fillna": "ffill", "transformations": {"0": "bkfilter", "1": "QuantileTransformer", "2": "DifferencedTransformer", "3": "HistoricValues", "4": "CumSumTransformer"}, "transformation_params": {"0": {}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {}, "3": {"window": 10}, "4": {}}}
8 - AverageValueNaive with avg smape 122.86:
Model Number: 9 of 16 with model AverageValueNaive for Validation 2 with params {"method": "Weighted_Mean", "window": null} and transformations {"fillna": "ffill", "transformations": {"0": "MaxAbsScaler", "1": "ClipOutliers", "2": "AlignLastDiff", "3": "StandardScaler", "4": "HistoricValues", "5": "CumSumTransformer"}, "transformation_params": {"0": {}, "1": {"method": "clip", "std_threshold": 4, "fillna": null}, "2": {"rows": 1, "displacement_rows": 1, "quantile": 1.0, "decay_span": null}, "3": {}, "4": {"window": 10}, "5": {}}}
9 - AverageValueNaive with avg smape 123.51:
Model Number: 10 of 16 with model GLS for Validation 2 with params {} and transformations {"fillna": "rolling_mean", "transformations": {"0": "RollingMeanTransformer", "1": "DifferencedTransformer", "2": "Detrend", "3": "Slice"}, "transformation_params": {"0": {"fixed": true, "window": 3}, "1": {}, "2": {"model": "Linear"}, "3": {"method": 100}}}
10 - GLS with avg smape 139.65:
Model Number: 11 of 16 with model AverageValueNaive for Validation 2 with params {"method": "Mean", "window": 96} and transformations {"fillna": "quadratic", "transformations": {"0": "AlignLastValue", "1": "LevelShiftTransformer", "2": "AlignLastValue", "3": "SeasonalDifference"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}, "1": {"window_size": 90, "alpha": 2.5, "grouping_forward_limit": 4, "max_level_shifts": 5, "alignment": "average"}, "2": {"rows": 1, "lag": 28, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "max"}, "3": {"lag_1": 7, "method": "LastValue"}}}
11 - AverageValueNaive with avg smape 122.77:
Model Number: 12 of 16 with model LastValueNaive for Validation 2 with params {} and transformations {"fillna": "ffill", "transformations": {"0": "AlignLastValue", "1": "DifferencedTransformer", "2": "MinMaxScaler"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "multiplicative", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "max"}, "1": {"lag": 1, "fill": "bfill"}, "2": {}}}
12 - LastValueNaive with avg smape 120.22:
Model Number: 13 of 16 with model LastValueNaive for Validation 2 with params {} and transformations {"fillna": "rolling_mean_24", "transformations": {"0": "EWMAFilter", "1": "MinMaxScaler", "2": "LevelShiftTransformer", "3": "AlignLastValue"}, "transformation_params": {"0": {"span": 3}, "1": {}, "2": {"window_size": 90, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 30, "alignment": "last_value"}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "max"}}}
13 - LastValueNaive with avg smape 123.57:
Model Number: 14 of 16 with model SeasonalityMotif for Validation 2 with params {"window": 10, "point_method": "median", "distance_metric": "mse", "k": 10, "datepart_method": "expanded_binarized", "independent": false} and transformations {"fillna": "fake_date", "transformations": {"0": "MinMaxScaler"}, "transformation_params": {"0": {}}}
14 - SeasonalityMotif with avg smape 124.04:
Model Number: 15 of 16 with model SectionalMotif for Validation 2 with params {"window": 10, "point_method": "mean", "distance_metric": "matching", "include_differenced": true, "k": 10, "stride_size": 1, "regression_type": null} and transformations {"fillna": "pchip", "transformations": {"0": "PCA", "1": "AlignLastValue", "2": "DiffSmoother", "3": "QuantileTransformer", "4": "AlignLastValue"}, "transformation_params": {"0": {"whiten": false, "n_components": null}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}, "2": {"method": "rolling_zscore", "method_params": {"distribution": "gamma", "alpha": 0.1, "rolling_periods": 200, "center": true}, "transform_dict": null, "reverse_alignment": true, "isolated_only": true, "fillna": "linear"}, "3": {"output_distribution": "uniform", "n_quantiles": 289}, "4": {"rows": 1, "lag": 1, "method": "multiplicative", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}}}
15 - SectionalMotif with avg smape 124.39:
Model Number: 16 of 16 with model LastValueNaive for Validation 2 with params {} and transformations {"fillna": "ffill", "transformations": {"0": "HistoricValues", "1": "AlignLastValue", "2": "SeasonalDifference", "3": "LevelShiftTransformer", "4": "SinTrend"}, "transformation_params": {"0": {"window": null}, "1": {"rows": 1, "lag": 2, "method": "additive", "strength": 1.0, "first_value_only": false}, "2": {"lag_1": 12, "method": 5}, "3": {"window_size": 30, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 10, "alignment": "average"}, "4": {}}}
16 - LastValueNaive with avg smape 129.11:
TotalRuntime missing in 3!
Validation Round: 1
Validation train index is DatetimeIndex(['2020-02-05', '2020-02-06', '2020-02-07', '2020-02-08',
'2020-02-09', '2020-02-10', '2020-02-11', '2020-02-12',
'2020-02-13', '2020-02-14',
...
'2023-02-28', '2023-03-01', '2023-03-02', '2023-03-03',
'2023-03-04', '2023-03-05', '2023-03-06', '2023-03-07',
'2023-03-08', '2023-03-09'],
dtype='datetime64[ns]', length=1129, freq=None)
TotalRuntime missing in 0!
Validation Round: 2
Validation train index is DatetimeIndex(['2020-02-05', '2020-02-06', '2020-02-07', '2020-02-08',
'2020-02-09', '2020-02-10', '2020-02-11', '2020-02-12',
'2020-02-13', '2020-02-14',
...
'2023-01-29', '2023-01-30', '2023-01-31', '2023-02-01',
'2023-02-02', '2023-02-03', '2023-02-04', '2023-02-05',
'2023-02-06', '2023-02-07'],
dtype='datetime64[ns]', length=1099, freq=None)
TotalRuntime missing in 0!
Model Number: 1 with model Ensemble in generation 0 of Horizontal Ensembles with params {"model_name": "Horizontal", "model_count": 2, "model_metric": "Score", "models": {"1ce393f73057c5cc2f28f904c54167b1": {"Model": "SeasonalityMotif", "ModelParameters": "{\"window\": 10, \"point_method\": \"median\", \"distance_metric\": \"mse\", \"k\": 10, \"datepart_method\": \"expanded_binarized\", \"independent\": false}", "TransformationParameters": "{\"fillna\": \"fake_date\", \"transformations\": {\"0\": \"MinMaxScaler\"}, \"transformation_params\": {\"0\": {}}}"}, "7c534919204ff0b8c75eeaaacb4212c2": {"Model": "ConstantNaive", "ModelParameters": "{\"constant\": 0}", "TransformationParameters": "{\"fillna\": \"cubic\", \"transformations\": {\"0\": \"StandardScaler\", \"1\": \"DifferencedTransformer\", \"2\": \"DifferencedTransformer\", \"3\": \"AlignLastValue\"}, \"transformation_params\": {\"0\": {}, \"1\": {\"lag\": 1, \"fill\": \"zero\"}, \"2\": {\"lag\": 1, \"fill\": \"bfill\"}, \"3\": {\"rows\": 1, \"lag\": 1, \"method\": \"additive\", \"strength\": 1.0, \"first_value_only\": false, \"threshold\": null, \"threshold_method\": \"mean\"}}}"}}, "series": {"PC1": "7c534919204ff0b8c75eeaaacb4212c2", "PC2": "1ce393f73057c5cc2f28f904c54167b1", "PC3": "7c534919204ff0b8c75eeaaacb4212c2", "PC4": "1ce393f73057c5cc2f28f904c54167b1", "PC5": "7c534919204ff0b8c75eeaaacb4212c2", "PC6": "7c534919204ff0b8c75eeaaacb4212c2", "PC7": "1ce393f73057c5cc2f28f904c54167b1", "PC8": "1ce393f73057c5cc2f28f904c54167b1", "PC9": "7c534919204ff0b8c75eeaaacb4212c2", "PC10": "1ce393f73057c5cc2f28f904c54167b1"}} and transformations {}
Ensemble Horizontal component 1 of 2 SeasonalityMotif started
Ensemble Horizontal component 2 of 2 ConstantNaive started
Ensemble Horizontal component 1 of 2 SeasonalityMotif started
Ensemble Horizontal component 2 of 2 ConstantNaive started
[12]:
PC1 | PC2 | PC3 | PC4 | PC5 | PC6 | PC7 | PC8 | PC9 | PC10 | |
---|---|---|---|---|---|---|---|---|---|---|
Date | ||||||||||
2023-06-03 | 5.967052 | 0.0 | 0.610980 | 0.0 | 0.972293 | 0.0 | 0.0 | 0.0 | 0.091757 | 0.0 |
2023-06-04 | 5.976850 | 0.0 | 0.612840 | 0.0 | 0.975080 | 0.0 | 0.0 | 0.0 | 0.093491 | 0.0 |
2023-06-05 | 5.986650 | 0.0 | 0.614699 | 0.0 | 0.977867 | 0.0 | 0.0 | 0.0 | 0.095226 | 0.0 |
2023-06-06 | 5.996450 | 0.0 | 0.616559 | 0.0 | 0.980655 | 0.0 | 0.0 | 0.0 | 0.096961 | 0.0 |
2023-06-07 | 6.006251 | 0.0 | 0.618419 | 0.0 | 0.983442 | 0.0 | 0.0 | 0.0 | 0.098697 | 0.0 |
2-3. Prediction of ODE parameter values
Now we have Y (estimated ODE parameter values) and X (estimated/forecasted indicator values without multicollinearity), we can predict ODE parameter values of future phases using ODEScenario().predict(days=<int>, name=<str>, seed=0, X=<pandas.DataFrame>)
. The new scenario is named “Predicted_with_X” here.
[13]:
snr.build_with_template(name="Predicted_with_X", template="Baseline")
snr.predict(days=30, name="Predicted_with_X", seed=0, X=future_df);
Using 1 cpus for n_jobs.
Data frequency is: D, used frequency is: D
Model Number: 1 with model AverageValueNaive in generation 0 of 1 with params {"method": "Mean"} and transformations {"fillna": "fake_date", "transformations": {"0": "DifferencedTransformer", "1": "SinTrend"}, "transformation_params": {"0": {}, "1": {}}}
Model Number: 2 with model AverageValueNaive in generation 0 of 1 with params {"method": "Mean"} and transformations {"fillna": "mean", "transformations": {"0": "ClipOutliers", "1": "QuantileTransformer", "2": "DifferencedTransformer"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 3, "fillna": null}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {}}}
Model Number: 3 with model AverageValueNaive in generation 0 of 1 with params {"method": "Mean"} and transformations {"fillna": "rolling_mean_24", "transformations": {"0": "SeasonalDifference", "1": "Round", "2": "Detrend"}, "transformation_params": {"0": {"lag_1": 7, "method": "Mean"}, "1": {"model": "middle", "decimals": 2, "on_transform": true, "on_inverse": false}, "2": {"model": "GLS"}}}
Model Number: 4 with model GLS in generation 0 of 1 with params {} and transformations {"fillna": "rolling_mean", "transformations": {"0": "RollingMeanTransformer", "1": "DifferencedTransformer", "2": "Detrend", "3": "Slice"}, "transformation_params": {"0": {"fixed": true, "window": 3}, "1": {}, "2": {"model": "Linear"}, "3": {"method": 100}}}
Model Number: 5 with model GLS in generation 0 of 1 with params {} and transformations {"fillna": "median", "transformations": {"0": "ClipOutliers", "1": "QuantileTransformer", "2": "RobustScaler", "3": "Round", "4": "MaxAbsScaler"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 3.5, "fillna": null}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {}, "3": {"model": "middle", "decimals": 2, "on_transform": true, "on_inverse": true}, "4": {}}}
Model Number: 6 with model LastValueNaive in generation 0 of 1 with params {} and transformations {"fillna": "mean", "transformations": {"0": "bkfilter", "1": "SinTrend", "2": "Detrend", "3": "PowerTransformer"}, "transformation_params": {"0": {}, "1": {}, "2": {"model": "Linear"}, "3": {}}}
Model Number: 7 with model LastValueNaive in generation 0 of 1 with params {} and transformations {"fillna": "rolling_mean_24", "transformations": {"0": "PositiveShift", "1": "SinTrend", "2": "bkfilter"}, "transformation_params": {"0": {}, "1": {}, "2": {}}}
Model Number: 8 with model LastValueNaive in generation 0 of 1 with params {} and transformations {"fillna": "fake_date", "transformations": {"0": "SeasonalDifference", "1": "SinTrend"}, "transformation_params": {"0": {"lag_1": 7, "method": "LastValue"}, "1": {}}}
Model Number: 9 with model LastValueNaive in generation 0 of 1 with params {} and transformations {"fillna": "mean", "transformations": {"0": "ClipOutliers", "1": "QuantileTransformer"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 1, "fillna": null}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}}}
Model Number: 10 with model SeasonalNaive in generation 0 of 1 with params {"method": "LastValue", "lag_1": 2, "lag_2": 7} and transformations {"fillna": "rolling_mean_24", "transformations": {"0": "SinTrend", "1": "Round", "2": "PowerTransformer"}, "transformation_params": {"0": {}, "1": {"model": "middle", "decimals": 2, "on_transform": false, "on_inverse": true}, "2": {}}}
Template Eval Error: Traceback (most recent call last):
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/tools/transform.py", line 5489, in _fit
df = self._fit_one(df, i)
^^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/tools/transform.py", line 5466, in _fit_one
df = self.transformers[i].fit_transform(df)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/sklearn/utils/_set_output.py", line 313, in wrapped
data_to_wrap = f(self, X, *args, **kwargs)
^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/sklearn/base.py", line 1473, in wrapper
return fit_method(estimator, *args, **kwargs)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/sklearn/preprocessing/_data.py", line 3272, in fit_transform
return self._fit(X, y, force_transform=True)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/sklearn/preprocessing/_data.py", line 3304, in _fit
self.lambdas_[i] = optim_function(col)
^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/sklearn/preprocessing/_data.py", line 3493, in _yeo_johnson_optimize
return optimize.brent(_neg_log_likelihood, brack=(-2, 2))
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/scipy/optimize/_optimize.py", line 2626, in brent
res = _minimize_scalar_brent(func, brack, args, **options)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/scipy/optimize/_optimize.py", line 2663, in _minimize_scalar_brent
brent.optimize()
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/scipy/optimize/_optimize.py", line 2433, in optimize
xa, xb, xc, fa, fb, fc, funcalls = self.get_bracket_info()
^^^^^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/scipy/optimize/_optimize.py", line 2402, in get_bracket_info
xa, xb, xc, fa, fb, fc, funcalls = bracket(func, xa=brack[0],
^^^^^^^^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/scipy/optimize/_optimize.py", line 3032, in bracket
raise e
scipy.optimize._optimize.BracketError: The algorithm terminated without finding a valid bracket. Consider trying different initial points.
The above exception was the direct cause of the following exception:
Traceback (most recent call last):
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 1646, in TemplateWizard
df_forecast = model_forecast(
^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 1493, in model_forecast
model = model.fit(df_train_low, future_regressor_train)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 860, in fit
df_train_transformed = self.transformer_object._fit(df)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/tools/transform.py", line 5494, in _fit
raise Exception(err_str) from e
Exception: Transformer PowerTransformer failed on fit from params rolling_mean_24 {'0': {}, '1': {'model': 'middle', 'decimals': 2, 'on_transform': False, 'on_inverse': True}, '2': {}}
in model 10 in generation 0: SeasonalNaive
Model Number: 11 with model SeasonalNaive in generation 0 of 1 with params {"method": "LastValue", "lag_1": 2, "lag_2": 1} and transformations {"fillna": "rolling_mean_24", "transformations": {"0": "SeasonalDifference", "1": "QuantileTransformer", "2": "Detrend"}, "transformation_params": {"0": {"lag_1": 12, "method": "Median"}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {"model": "GLS"}}}
Model Number: 12 with model SeasonalNaive in generation 0 of 1 with params {"method": "LastValue", "lag_1": 7, "lag_2": 2} and transformations {"fillna": "mean", "transformations": {"0": "QuantileTransformer", "1": "ClipOutliers"}, "transformation_params": {"0": {"output_distribution": "uniform", "n_quantiles": 1000}, "1": {"method": "clip", "std_threshold": 2, "fillna": null}}}
Model Number: 13 with model ConstantNaive in generation 0 of 1 with params {} and transformations {"fillna": "ffill", "transformations": {"0": "PowerTransformer", "1": "QuantileTransformer", "2": "SeasonalDifference"}, "transformation_params": {"0": {}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {"lag_1": 7, "method": "LastValue"}}}
/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/sklearn/preprocessing/_data.py:3438: RuntimeWarning: overflow encountered in power
out[pos] = (np.power(x[pos] + 1, lmbda) - 1) / lmbda
Model Number: 14 with model SeasonalNaive in generation 0 of 1 with params {"method": "lastvalue", "lag_1": 364, "lag_2": 28} and transformations {"fillna": "ffill", "transformations": {"0": "SeasonalDifference", "1": "MaxAbsScaler", "2": "Round"}, "transformation_params": {"0": {"lag_1": 7, "method": "LastValue"}, "1": {}, "2": {"decimals": 0, "on_transform": false, "on_inverse": true}}}
Model Number: 15 with model SectionalMotif in generation 0 of 1 with params {"window": 10, "point_method": "weighted_mean", "distance_metric": "sokalmichener", "include_differenced": true, "k": 20, "stride_size": 1, "regression_type": null} and transformations {"fillna": "zero", "transformations": {"0": null}, "transformation_params": {"0": {}}}
Model Number: 16 with model SectionalMotif in generation 0 of 1 with params {"window": 5, "point_method": "midhinge", "distance_metric": "canberra", "include_differenced": false, "k": 10, "stride_size": 1, "regression_type": null} and transformations {"fillna": "rolling_mean_24", "transformations": {"0": "QuantileTransformer", "1": "QuantileTransformer", "2": "RobustScaler"}, "transformation_params": {"0": {"output_distribution": "uniform", "n_quantiles": 1000}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {}}}
Model Number: 17 with model SeasonalNaive in generation 0 of 1 with params {"method": "lastvalue", "lag_1": 364, "lag_2": 30} and transformations {"fillna": "fake_date", "transformations": {"0": "SeasonalDifference", "1": "MaxAbsScaler", "2": "Round"}, "transformation_params": {"0": {"lag_1": 7, "method": "LastValue"}, "1": {}, "2": {"decimals": 0, "on_transform": false, "on_inverse": true}}}
Model Number: 18 with model SeasonalityMotif in generation 0 of 1 with params {"window": 5, "point_method": "weighted_mean", "distance_metric": "mae", "k": 10, "datepart_method": "common_fourier"} and transformations {"fillna": "nearest", "transformations": {"0": "AlignLastValue"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "multiplicative", "strength": 1.0, "first_value_only": false}}}
Model Number: 19 with model SectionalMotif in generation 0 of 1 with params {"window": 7, "point_method": "median", "distance_metric": "canberra", "include_differenced": true, "k": 5, "stride_size": 1, "regression_type": null} and transformations {"fillna": "ffill", "transformations": {"0": "SeasonalDifference", "1": "AlignLastValue", "2": "SeasonalDifference", "3": "LevelShiftTransformer"}, "transformation_params": {"0": {"lag_1": 7, "method": "Median"}, "1": {"rows": 1, "lag": 2, "method": "additive", "strength": 1.0, "first_value_only": false}, "2": {"lag_1": 12, "method": 5}, "3": {"window_size": 30, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 10, "alignment": "average"}}}
Model Number: 20 with model ConstantNaive in generation 0 of 1 with params {"constant": 1} and transformations {"fillna": "zero", "transformations": {"0": "ClipOutliers", "1": "Detrend", "2": "AnomalyRemoval"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 3, "fillna": null}, "1": {"model": "GLS", "phi": 1, "window": 30, "transform_dict": null}, "2": {"method": "zscore", "method_params": {"distribution": "chi2", "alpha": 0.1}, "fillna": "fake_date", "transform_dict": {"fillna": "quadratic", "transformations": {"0": "AlignLastValue"}, "transformation_params": {"0": {"rows": 4, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}, "isolated_only": false}}}
Model Number: 21 with model LastValueNaive in generation 0 of 1 with params {} and transformations {"fillna": "nearest", "transformations": {"0": "LevelShiftTransformer", "1": "MaxAbsScaler", "2": "AnomalyRemoval", "3": "AlignLastValue", "4": "AlignLastValue"}, "transformation_params": {"0": {"window_size": 90, "alpha": 3.0, "grouping_forward_limit": 3, "max_level_shifts": 5, "alignment": "rolling_diff"}, "1": {}, "2": {"method": "IQR", "method_params": {"iqr_threshold": 1.5, "iqr_quantiles": [0.4, 0.6]}, "fillna": "linear", "transform_dict": null, "isolated_only": true}, "3": {"rows": 1, "lag": 1, "method": "multiplicative", "strength": 0.7, "first_value_only": false, "threshold": 1, "threshold_method": "max"}, "4": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.5, "first_value_only": false, "threshold": 10, "threshold_method": "mean"}}}
Model Number: 22 with model AverageValueNaive in generation 0 of 1 with params {"method": "Median", "window": null} and transformations {"fillna": "ffill", "transformations": {"0": "Detrend", "1": "Detrend"}, "transformation_params": {"0": {"model": "Linear", "phi": 1, "window": null, "transform_dict": null}, "1": {"model": "Linear", "phi": 1, "window": null, "transform_dict": {"fillna": null, "transformations": {"0": "ClipOutliers"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 4}}}}}}
Model Number: 23 with model GLS in generation 0 of 1 with params {} and transformations {"fillna": "akima", "transformations": {"0": "AlignLastValue", "1": "AnomalyRemoval", "2": "Discretize", "3": "Discretize", "4": "DifferencedTransformer"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}, "1": {"method": "IQR", "method_params": {"iqr_threshold": 3.0, "iqr_quantiles": [0.25, 0.75]}, "fillna": "ffill", "transform_dict": null, "isolated_only": false}, "2": {"discretization": "upper", "n_bins": 5}, "3": {"discretization": "center", "n_bins": 5}, "4": {"lag": 1, "fill": "zero"}}}
Model Number: 24 with model SeasonalNaive in generation 0 of 1 with params {"method": "lastvalue", "lag_1": 2, "lag_2": 60} and transformations {"fillna": "ffill", "transformations": {"0": "ClipOutliers", "1": "Detrend"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 4, "fillna": null}, "1": {"model": "GLS", "phi": 1, "window": null, "transform_dict": {"fillna": null, "transformations": {"0": "ClipOutliers"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 4}}}}}}
Model Number: 25 with model SeasonalityMotif in generation 0 of 1 with params {"window": 5, "point_method": "midhinge", "distance_metric": "mae", "k": 1, "datepart_method": "recurring", "independent": false} and transformations {"fillna": "ffill", "transformations": {"0": "AlignLastValue", "1": "HPFilter"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 10, "threshold_method": "max"}, "1": {"part": "trend", "lamb": 1600}}}
Model Number: 26 with model SectionalMotif in generation 0 of 1 with params {"window": 10, "point_method": "mean", "distance_metric": "matching", "include_differenced": true, "k": 10, "stride_size": 1, "regression_type": null} and transformations {"fillna": "pchip", "transformations": {"0": "PCA", "1": "AlignLastValue", "2": "DiffSmoother", "3": "QuantileTransformer", "4": "AlignLastValue"}, "transformation_params": {"0": {"whiten": false, "n_components": null}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}, "2": {"method": "rolling_zscore", "method_params": {"distribution": "gamma", "alpha": 0.1, "rolling_periods": 200, "center": true}, "transform_dict": null, "reverse_alignment": true, "isolated_only": true, "fillna": "linear"}, "3": {"output_distribution": "uniform", "n_quantiles": "quarter"}, "4": {"rows": 1, "lag": 1, "method": "multiplicative", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}}}
Model Number: 27 with model SeasonalNaive in generation 0 of 1 with params {"method": "median", "lag_1": 24, "lag_2": 28} and transformations {"fillna": "ffill_mean_biased", "transformations": {"0": "AlignLastValue"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 10, "threshold_method": "max"}}}
Model Number: 28 with model SectionalMotif in generation 0 of 1 with params {"window": 10, "point_method": "weighted_mean", "distance_metric": "chebyshev", "include_differenced": true, "k": 10, "stride_size": 1, "regression_type": null} and transformations {"fillna": "mean", "transformations": {"0": "ClipOutliers", "1": "Detrend", "2": "PowerTransformer", "3": "AlignLastValue"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 4, "fillna": null}, "1": {"model": "Linear", "phi": 0.999, "window": null, "transform_dict": null}, "2": {}, "3": {"rows": 1, "lag": 28, "method": "multiplicative", "strength": 0.9, "first_value_only": false, "threshold": null, "threshold_method": "mean"}}}
Template Eval Error: Traceback (most recent call last):
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 1646, in TemplateWizard
df_forecast = model_forecast(
^^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 1494, in model_forecast
return model.predict(
^^^^^^^^^^^^^^
File "/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/autots/evaluator/auto_model.py", line 989, in predict
raise ValueError(
ValueError: Model returned NaN due to a preprocessing transformer {'fillna': 'mean', 'transformations': {'0': 'ClipOutliers', '1': 'Detrend', '2': 'PowerTransformer', '3': 'AlignLastValue'}, 'transformation_params': {'0': {'method': 'clip', 'std_threshold': 4, 'fillna': None}, '1': {'model': 'Linear', 'phi': 0.999, 'window': None, 'transform_dict': None}, '2': {}, '3': {'rows': 1, 'lag': 28, 'method': 'multiplicative', 'strength': 0.9, 'first_value_only': False, 'threshold': None, 'threshold_method': 'mean'}}}. fail_on_forecast_nan=True
in model 28 in generation 0: SectionalMotif
Model Number: 29 with model AverageValueNaive in generation 0 of 1 with params {"method": "Mean", "window": null} and transformations {"fillna": "quadratic", "transformations": {"0": "STLFilter"}, "transformation_params": {"0": {"decomp_type": "STL", "part": "trend", "seasonal": 7}}}
Model Number: 30 with model LastValueNaive in generation 0 of 1 with params {} and transformations {"fillna": "rolling_mean_24", "transformations": {"0": "EWMAFilter", "1": "MinMaxScaler", "2": "LevelShiftTransformer", "3": "AlignLastValue"}, "transformation_params": {"0": {"span": 3}, "1": {}, "2": {"window_size": 90, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 30, "alignment": "last_value"}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "max"}}}
Model Number: 31 with model AverageValueNaive in generation 0 of 1 with params {"method": "Weighted_Mean", "window": 168} and transformations {"fillna": "quadratic", "transformations": {"0": "AlignLastValue", "1": "LevelShiftTransformer", "2": "AlignLastValue", "3": "SeasonalDifference"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}, "1": {"window_size": 90, "alpha": 2.5, "grouping_forward_limit": 4, "max_level_shifts": 5, "alignment": "average"}, "2": {"rows": 1, "lag": 28, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "max"}, "3": {"lag_1": 7, "method": "LastValue"}}}
Model Number: 32 with model AverageValueNaive in generation 0 of 1 with params {"method": "Exp_Weighted_Mean", "window": null} and transformations {"fillna": "nearest", "transformations": {"0": null}, "transformation_params": {"0": {}}}
Model Number: 33 with model SectionalMotif in generation 0 of 1 with params {"window": 50, "point_method": "weighted_mean", "distance_metric": "russellrao", "include_differenced": true, "k": 10, "stride_size": 1, "regression_type": null} and transformations {"fillna": "ffill", "transformations": {"0": "AlignLastValue", "1": "RegressionFilter", "2": "LevelShiftTransformer"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "multiplicative", "strength": 1.0, "first_value_only": true, "threshold": null, "threshold_method": "mean"}, "1": {"sigma": 3, "rolling_window": 90, "run_order": "season_first", "regression_params": {"regression_model": {"model": "ElasticNet", "model_params": {"l1_ratio": 0.5, "fit_intercept": true, "selection": "cyclic"}}, "datepart_method": "recurring", "polynomial_degree": null, "transform_dict": {"fillna": null, "transformations": {"0": "EWMAFilter"}, "transformation_params": {"0": {"span": 7}}}, "holiday_countries_used": false, "lags": null, "forward_lags": null}, "holiday_params": null, "trend_method": "rolling_mean"}, "2": {"window_size": 90, "alpha": 2.5, "grouping_forward_limit": 3, "max_level_shifts": 5, "alignment": "average"}}}
Model Number: 34 with model SectionalMotif in generation 0 of 1 with params {"window": 10, "point_method": "median", "distance_metric": "cityblock", "include_differenced": true, "k": 10, "stride_size": 1, "regression_type": "User"} and transformations {"fillna": "ffill_mean_biased", "transformations": {"0": "EWMAFilter", "1": "StandardScaler"}, "transformation_params": {"0": {"span": 12}, "1": {}}}
Model Number: 35 with model AverageValueNaive in generation 0 of 1 with params {"method": "Weighted_Mean", "window": 2} and transformations {"fillna": "KNNImputer", "transformations": {"0": "Slice", "1": "AlignLastValue"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}
Model Number: 36 with model GLS in generation 0 of 1 with params {} and transformations {"fillna": "linear", "transformations": {"0": "ClipOutliers", "1": "Detrend", "2": "PowerTransformer", "3": "AlignLastValue"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 3, "fillna": null}, "1": {"model": "Linear", "phi": 1, "window": null, "transform_dict": {"fillna": null, "transformations": {"0": "EWMAFilter"}, "transformation_params": {"0": {"span": 2}}}}, "2": {}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.7, "first_value_only": true, "threshold": null, "threshold_method": "max"}}}
Model Number: 37 with model ConstantNaive in generation 0 of 1 with params {"constant": 1} and transformations {"fillna": "fake_date", "transformations": {"0": "AlignLastValue", "1": "KalmanSmoothing"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": true, "threshold": null, "threshold_method": "mean"}, "1": {"model_name": "spline", "state_transition": [[2, -1], [1, 0]], "process_noise": [[1, 0], [0, 0]], "observation_model": [[1, 0]], "observation_noise": 0.1, "em_iter": null, "on_transform": true, "on_inverse": false}}}
Model Number: 38 with model GLS in generation 0 of 1 with params {} and transformations {"fillna": "fake_date", "transformations": {"0": "HolidayTransformer", "1": "FFTFilter", "2": "CumSumTransformer", "3": "SeasonalDifference"}, "transformation_params": {"0": {"threshold": 0.8, "splash_threshold": null, "use_dayofmonth_holidays": true, "use_wkdom_holidays": true, "use_wkdeom_holidays": false, "use_lunar_holidays": false, "use_lunar_weekday": false, "use_islamic_holidays": true, "use_hebrew_holidays": false, "anomaly_detector_params": {"method": "mad", "method_params": {"distribution": "uniform", "alpha": 0.05}, "fillna": "ffill", "transform_dict": {"transformations": {"0": "DatepartRegression"}, "transformation_params": {"0": {"datepart_method": "simple_3", "regression_model": {"model": "FastRidge", "model_params": {}}}}}, "isolated_only": false}, "remove_excess_anomalies": true, "impact": "regression", "regression_params": {}}, "1": {"cutoff": 0.1, "reverse": false}, "2": {}, "3": {"lag_1": 7, "method": "Mean"}}}
Model Number: 39 with model ConstantNaive in generation 0 of 1 with params {"constant": 0} and transformations {"fillna": "ffill", "transformations": {"0": "StandardScaler", "1": "Round", "2": "IntermittentOccurrence"}, "transformation_params": {"0": {}, "1": {"decimals": 1, "on_transform": true, "on_inverse": false}, "2": {"center": "mean"}}}
Model Number: 40 with model GLS in generation 0 of 1 with params {} and transformations {"fillna": "cubic", "transformations": {"0": "ClipOutliers", "1": "Detrend", "2": "DiffSmoother", "3": "AlignLastValue"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 3, "fillna": null}, "1": {"model": "Linear", "phi": 1, "window": 900, "transform_dict": null}, "2": {"method": null, "method_params": null, "transform_dict": null, "reverse_alignment": true, "isolated_only": false, "fillna": 1.0}, "3": {"rows": 7, "lag": 28, "method": "additive", "strength": 0.7, "first_value_only": false, "threshold": null, "threshold_method": "max"}}}
/home/runner/work/covid19-sir/covid19-sir/.venv/lib/python3.12/site-packages/sklearn/linear_model/_ridge.py:216: LinAlgWarning: Ill-conditioned matrix (rcond=9.11298e-26): result may not be accurate.
return linalg.solve(A, Xy, assume_a="pos", overwrite_a=True).T
Model Number: 41 with model AverageValueNaive in generation 0 of 1 with params {"method": "Mean", "window": null} and transformations {"fillna": "akima", "transformations": {"0": "LevelShiftTransformer"}, "transformation_params": {"0": {"window_size": 364, "alpha": 3.0, "grouping_forward_limit": 4, "max_level_shifts": 5, "alignment": "average"}}}
Model Number: 42 with model SeasonalityMotif in generation 0 of 1 with params {"window": 10, "point_method": "median", "distance_metric": "minkowski", "k": 20, "datepart_method": "simple_2", "independent": false} and transformations {"fillna": "time", "transformations": {"0": "bkfilter", "1": "MaxAbsScaler", "2": "StandardScaler", "3": "QuantileTransformer", "4": "cffilter"}, "transformation_params": {"0": {}, "1": {}, "2": {}, "3": {"output_distribution": "uniform", "n_quantiles": 20}, "4": {}}}
Model Number: 43 with model SeasonalityMotif in generation 0 of 1 with params {"window": 10, "point_method": "median", "distance_metric": "mse", "k": 10, "datepart_method": "expanded_binarized", "independent": false} and transformations {"fillna": "fake_date", "transformations": {"0": "MinMaxScaler"}, "transformation_params": {"0": {}}}
Model Number: 44 with model AverageValueNaive in generation 0 of 1 with params {"method": "Mean", "window": 420} and transformations {"fillna": "ffill", "transformations": {"0": "ClipOutliers", "1": "Detrend"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 1, "fillna": null}, "1": {"model": "GLS", "phi": 1, "window": null, "transform_dict": {"fillna": null, "transformations": {"0": "ClipOutliers"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 4}}}}}}
Model Number: 45 with model LastValueNaive in generation 0 of 1 with params {} and transformations {"fillna": "ffill", "transformations": {"0": "AlignLastValue", "1": "DifferencedTransformer", "2": "MinMaxScaler"}, "transformation_params": {"0": {"rows": 1, "lag": 1, "method": "multiplicative", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "max"}, "1": {"lag": 1, "fill": "bfill"}, "2": {}}}
Model Number: 46 with model GLS in generation 0 of 1 with params {} and transformations {"fillna": "fake_date", "transformations": {"0": "LevelShiftTransformer", "1": "FFTDecomposition", "2": "AlignLastValue", "3": "PositiveShift"}, "transformation_params": {"0": {"window_size": 30, "alpha": 3.5, "grouping_forward_limit": 3, "max_level_shifts": 10, "alignment": "average"}, "1": {"n_harmonics": null, "detrend": null}, "2": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 10, "threshold_method": "max"}, "3": {}}}
Model Number: 47 with model AverageValueNaive in generation 0 of 1 with params {"method": "Weighted_Mean", "window": null} and transformations {"fillna": "ffill_mean_biased", "transformations": {"0": "QuantileTransformer", "1": "SeasonalDifference", "2": "cffilter", "3": "AlignLastValue", "4": "PositiveShift"}, "transformation_params": {"0": {"output_distribution": "uniform", "n_quantiles": 1000}, "1": {"lag_1": 7, "method": "Mean"}, "2": {}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "max"}, "4": {}}}
Model Number: 48 with model SeasonalityMotif in generation 0 of 1 with params {"window": 7, "point_method": "median", "distance_metric": "minkowski", "k": 10, "datepart_method": "recurring", "independent": false} and transformations {"fillna": "ffill_mean_biased", "transformations": {"0": "ClipOutliers"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 3, "fillna": null}}}
Model Number: 49 with model SeasonalityMotif in generation 0 of 1 with params {"window": 10, "point_method": "weighted_mean", "distance_metric": "chebyshev", "k": 10, "datepart_method": "expanded_binarized", "independent": false} and transformations {"fillna": "median", "transformations": {"0": "Round", "1": "SeasonalDifference"}, "transformation_params": {"0": {"decimals": -2, "on_transform": true, "on_inverse": true}, "1": {"lag_1": 7, "method": "LastValue"}}}
Model Number: 50 with model ConstantNaive in generation 0 of 1 with params {"constant": 0} and transformations {"fillna": "cubic", "transformations": {"0": "StandardScaler", "1": "DifferencedTransformer", "2": "SinTrend", "3": "AlignLastValue"}, "transformation_params": {"0": {}, "1": {"lag": 1, "fill": "zero"}, "2": {}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "mean"}}}
Model Number: 51 with model LastValueNaive in generation 0 of 1 with params {} and transformations {"fillna": "rolling_mean", "transformations": {"0": "SinTrend", "1": "StandardScaler", "2": "Discretize"}, "transformation_params": {"0": {}, "1": {}, "2": {"discretization": "center", "n_bins": 20}}}
Model Number: 52 with model ConstantNaive in generation 0 of 1 with params {"constant": 0.1} and transformations {"fillna": "mean", "transformations": {"0": "ClipOutliers", "1": "Detrend", "2": "SeasonalDifference", "3": "AlignLastValue"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 2, "fillna": null}, "1": {"model": "GLS", "phi": 1, "window": null, "transform_dict": null}, "2": {"lag_1": 12, "method": "Median"}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}}}
Model Number: 53 with model GLS in generation 0 of 1 with params {} and transformations {"fillna": "ffill", "transformations": {"0": "QuantileTransformer", "1": "EWMAFilter", "2": "MinMaxScaler", "3": "convolution_filter"}, "transformation_params": {"0": {"output_distribution": "normal", "n_quantiles": "quarter"}, "1": {"span": 3}, "2": {}, "3": {}}}
Model Number: 54 with model SeasonalNaive in generation 0 of 1 with params {"method": "mean", "lag_1": 96, "lag_2": 364} and transformations {"fillna": "ffill", "transformations": {"0": "KalmanSmoothing"}, "transformation_params": {"0": {"model_name": "factor", "state_transition": [[1, 1, 0, 0, 0, 0], [0, 1, 0, 0, 0, 0], [0, 0, 1, 0, 0, 0], [0, 0, 0, 1, 1, 0], [0, 0, 0, 0, 1, 0], [0, 0, 0, 0, 0, 1]], "process_noise": [[1, 0, 0, 0, 0, 0], [0, 1, 0, 0, 0, 0], [0, 0, 0, 0, 0, 0], [0, 0, 1, 0, 0, 0], [0, 0, 0, 1, 0, 0], [0, 0, 0, 0, 0, 0]], "observation_model": [[1, 0, 0, 0, 0, 0]], "observation_noise": 0.04, "em_iter": null, "on_transform": false, "on_inverse": true}}}
New Generation: 1 of 1
Model Number: 55 with model LastValueNaive in generation 1 of 1 with params {} and transformations {"fillna": "KNNImputer", "transformations": {"0": "ClipOutliers", "1": "AlignLastValue"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 4, "fillna": null}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}
Model Number: 56 with model AverageValueNaive in generation 1 of 1 with params {"method": "Weighted_Mean", "window": null} and transformations {"fillna": "ffill_mean_biased", "transformations": {"0": "ClipOutliers", "1": "SeasonalDifference"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 1, "fillna": null}, "1": {"lag_1": 7, "method": "Mean"}}}
Model Number: 57 with model SeasonalityMotif in generation 1 of 1 with params {"window": 10, "point_method": "weighted_mean", "distance_metric": "minkowski", "k": 100, "datepart_method": "simple_2", "independent": false} and transformations {"fillna": "ffill", "transformations": {"0": "RobustScaler", "1": "AlignLastValue", "2": "SeasonalDifference"}, "transformation_params": {"0": {}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "max"}, "2": {"lag_1": 81, "method": "Median"}}}
Model Number: 58 with model ConstantNaive in generation 1 of 1 with params {"constant": 0} and transformations {"fillna": "ffill", "transformations": {"0": "PowerTransformer", "1": "QuantileTransformer", "2": "FFTFilter"}, "transformation_params": {"0": {}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {"cutoff": 0.2, "reverse": false}}}
Model Number: 59 with model LastValueNaive in generation 1 of 1 with params {} and transformations {"fillna": "KNNImputer", "transformations": {"0": "CumSumTransformer", "1": "AlignLastValue"}, "transformation_params": {"0": {}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}
Model Number: 60 with model AverageValueNaive in generation 1 of 1 with params {"method": "Weighted_Mean", "window": 12} and transformations {"fillna": "ffill_mean_biased", "transformations": {"0": "Slice", "1": "AlignLastValue"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}
Model Number: 61 with model AverageValueNaive in generation 1 of 1 with params {"method": "Midhinge", "window": null} and transformations {"fillna": "KNNImputer", "transformations": {"0": "ClipOutliers", "1": "QuantileTransformer"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 3, "fillna": null}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}}}
Model Number: 62 with model SeasonalNaive in generation 1 of 1 with params {"method": "mean", "lag_1": 7, "lag_2": 2} and transformations {"fillna": "zero", "transformations": {"0": "PowerTransformer", "1": "ClipOutliers"}, "transformation_params": {"0": {}, "1": {"method": "clip", "std_threshold": 2, "fillna": null}}}
Model Number: 63 with model SeasonalityMotif in generation 1 of 1 with params {"window": 5, "point_method": "midhinge", "distance_metric": "mse", "k": 10, "datepart_method": "expanded_binarized", "independent": false} and transformations {"fillna": "KNNImputer", "transformations": {"0": "RobustScaler", "1": "AlignLastValue", "2": "SeasonalDifference"}, "transformation_params": {"0": {}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "max"}, "2": {"lag_1": 81, "method": "Median"}}}
Model Number: 64 with model SeasonalityMotif in generation 1 of 1 with params {"window": 7, "point_method": "median", "distance_metric": "canberra", "k": 20, "datepart_method": "simple_2", "independent": false} and transformations {"fillna": "fake_date", "transformations": {"0": "MinMaxScaler", "1": "StandardScaler", "2": "cffilter"}, "transformation_params": {"0": {}, "1": {}, "2": {}}}
Model Number: 65 with model SectionalMotif in generation 1 of 1 with params {"window": 50, "point_method": "weighted_mean", "distance_metric": "jaccard", "include_differenced": true, "k": 10, "stride_size": 1, "regression_type": null} and transformations {"fillna": "rolling_mean_24", "transformations": {"0": "SinTrend", "1": "RegressionFilter", "2": "SeasonalDifference"}, "transformation_params": {"0": {}, "1": {"sigma": 3, "rolling_window": 90, "run_order": "season_first", "regression_params": {"regression_model": {"model": "ElasticNet", "model_params": {"l1_ratio": 0.5, "fit_intercept": true, "selection": "cyclic"}}, "datepart_method": "recurring", "polynomial_degree": null, "transform_dict": {"fillna": null, "transformations": {"0": "EWMAFilter"}, "transformation_params": {"0": {"span": 7}}}, "holiday_countries_used": false, "lags": null, "forward_lags": null}, "holiday_params": null, "trend_method": "rolling_mean"}, "2": {"lag_1": 364, "method": 20}}}
Model Number: 66 with model SeasonalNaive in generation 1 of 1 with params {"method": "lastvalue", "lag_1": 4, "lag_2": 28} and transformations {"fillna": "ffill", "transformations": {"0": "RollingMean100thN", "1": "MaxAbsScaler"}, "transformation_params": {"0": {}, "1": {}}}
Model Number: 67 with model AverageValueNaive in generation 1 of 1 with params {"method": "trimmed_mean_20", "window": null} and transformations {"fillna": "mean", "transformations": {"0": "QuantileTransformer", "1": "QuantileTransformer", "2": "cffilter", "3": "PositiveShift"}, "transformation_params": {"0": {"output_distribution": "uniform", "n_quantiles": 1000}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {}, "3": {}}}
Model Number: 68 with model GLS in generation 1 of 1 with params {} and transformations {"fillna": "time", "transformations": {"0": "RollingMeanTransformer", "1": "DifferencedTransformer"}, "transformation_params": {"0": {"fixed": true, "window": 364, "macro_micro": false, "center": false}, "1": {"lag": 1, "fill": "zero"}}}
Model Number: 69 with model ConstantNaive in generation 1 of 1 with params {"constant": 0} and transformations {"fillna": "mean", "transformations": {"0": "ClipOutliers", "1": "StandardScaler", "2": "SeasonalDifference"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 2, "fillna": null}, "1": {}, "2": {"lag_1": 12, "method": "Median"}}}
Model Number: 70 with model ConstantNaive in generation 1 of 1 with params {"constant": -1} and transformations {"fillna": "ffill", "transformations": {"0": "PowerTransformer", "1": "RegressionFilter", "2": "FFTFilter", "3": "HolidayTransformer"}, "transformation_params": {"0": {}, "1": {"sigma": 0.5, "rolling_window": 90, "run_order": "season_first", "regression_params": {"regression_model": {"model": "DecisionTree", "model_params": {"max_depth": 3, "min_samples_split": 2}}, "datepart_method": "common_fourier", "polynomial_degree": null, "transform_dict": null, "holiday_countries_used": false, "lags": null, "forward_lags": 4}, "holiday_params": null, "trend_method": "local_linear"}, "2": {"cutoff": 0.2, "reverse": false}, "3": {"threshold": 0.8, "splash_threshold": null, "use_dayofmonth_holidays": true, "use_wkdom_holidays": true, "use_wkdeom_holidays": false, "use_lunar_holidays": false, "use_lunar_weekday": false, "use_islamic_holidays": false, "use_hebrew_holidays": false, "anomaly_detector_params": {"method": "zscore", "method_params": {"distribution": "chi2", "alpha": 0.03}, "fillna": "linear", "transform_dict": {"transformations": {"0": "DatepartRegression"}, "transformation_params": {"0": {"datepart_method": "simple_3", "regression_model": {"model": "ElasticNet", "model_params": {}}}}}, "isolated_only": false}, "remove_excess_anomalies": true, "impact": null, "regression_params": {}}}}
Model Number: 71 with model SeasonalNaive in generation 1 of 1 with params {"method": "lastvalue", "lag_1": 364, "lag_2": 30} and transformations {"fillna": "mean", "transformations": {"0": "StandardScaler", "1": "ClipOutliers"}, "transformation_params": {"0": {}, "1": {"method": "clip", "std_threshold": 2, "fillna": null}}}
Model Number: 72 with model AverageValueNaive in generation 1 of 1 with params {"method": "Mean", "window": null} and transformations {"fillna": "ffill_mean_biased", "transformations": {"0": "Slice", "1": "Round"}, "transformation_params": {"0": {"method": 0.5}, "1": {"decimals": 0, "on_transform": false, "on_inverse": true}}}
Model Number: 73 with model GLS in generation 1 of 1 with params {} and transformations {"fillna": "ffill_mean_biased", "transformations": {"0": "ReplaceConstant", "1": "bkfilter"}, "transformation_params": {"0": {"constant": 0, "reintroduction_model": null, "fillna": "linear"}, "1": {}}}
Model Number: 74 with model SeasonalNaive in generation 1 of 1 with params {"method": "lastvalue", "lag_1": 364, "lag_2": 28} and transformations {"fillna": "ffill_mean_biased", "transformations": {"0": "QuantileTransformer", "1": "DifferencedTransformer", "2": "AnomalyRemoval"}, "transformation_params": {"0": {"output_distribution": "uniform", "n_quantiles": 1000}, "1": {"lag": 7, "fill": "bfill"}, "2": {"method": "rolling_zscore", "method_params": {"distribution": "uniform", "alpha": 0.03, "rolling_periods": 200, "center": true}, "fillna": "linear", "transform_dict": {"transformations": {"0": "DifferencedTransformer"}, "transformation_params": {"0": {}}}, "isolated_only": false}}}
Model Number: 75 with model SeasonalNaive in generation 1 of 1 with params {"method": "lastvalue", "lag_1": 24, "lag_2": 28} and transformations {"fillna": "fake_date", "transformations": {"0": "Slice", "1": "AlignLastValue", "2": "Round"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}, "2": {"decimals": 0, "on_transform": false, "on_inverse": true}}}
Model Number: 76 with model SeasonalNaive in generation 1 of 1 with params {"method": "mean", "lag_1": 24, "lag_2": 28} and transformations {"fillna": "KNNImputer", "transformations": {"0": "Slice", "1": "AlignLastValue"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}
Model Number: 77 with model AverageValueNaive in generation 1 of 1 with params {"method": "Median", "window": 2} and transformations {"fillna": "akima", "transformations": {"0": "HPFilter", "1": "AlignLastValue", "2": "cffilter", "3": "AlignLastValue", "4": "PositiveShift"}, "transformation_params": {"0": {"part": "trend", "lamb": 104976000000}, "1": {"rows": 7, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "max"}, "2": {}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "max"}, "4": {}}}
Model Number: 78 with model LastValueNaive in generation 1 of 1 with params {} and transformations {"fillna": "KNNImputer", "transformations": {"0": "Slice", "1": "AlignLastValue", "2": "AlignLastValue"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}, "2": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "max"}}}
Model Number: 79 with model SeasonalNaive in generation 1 of 1 with params {"method": "mean", "lag_1": 24, "lag_2": 28} and transformations {"fillna": "ffill", "transformations": {"0": "bkfilter", "1": "MaxAbsScaler", "2": "StandardScaler"}, "transformation_params": {"0": {}, "1": {}, "2": {}}}
Model Number: 80 with model AverageValueNaive in generation 1 of 1 with params {"method": "Weighted_Mean", "window": 168} and transformations {"fillna": "linear", "transformations": {"0": "PCA", "1": "LevelShiftTransformer", "2": "AlignLastValue", "3": "SeasonalDifference"}, "transformation_params": {"0": {"whiten": true, "n_components": null}, "1": {"window_size": 90, "alpha": 2.5, "grouping_forward_limit": 4, "max_level_shifts": 5, "alignment": "average"}, "2": {"rows": 1, "lag": 28, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "max"}, "3": {"lag_1": 7, "method": "LastValue"}}}
Model Number: 81 with model SeasonalityMotif in generation 1 of 1 with params {"window": 10, "point_method": "median", "distance_metric": "minkowski", "k": 20, "datepart_method": "expanded_binarized", "independent": false} and transformations {"fillna": "time", "transformations": {"0": "bkfilter", "1": "AlignLastValue", "2": "QuantileTransformer", "3": "cffilter"}, "transformation_params": {"0": {}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}, "2": {"output_distribution": "uniform", "n_quantiles": 20}, "3": {}}}
Model Number: 82 with model SeasonalNaive in generation 1 of 1 with params {"method": "lastvalue", "lag_1": 7, "lag_2": 168} and transformations {"fillna": "zero", "transformations": {"0": "SeasonalDifference", "1": "RegressionFilter", "2": "Round"}, "transformation_params": {"0": {"lag_1": 7, "method": "LastValue"}, "1": {"sigma": 2, "rolling_window": 90, "run_order": "trend_first", "regression_params": {"regression_model": {"model": "DecisionTree", "model_params": {"max_depth": null, "min_samples_split": 0.05}}, "datepart_method": ["weekdaymonthofyear", "quarter", "dayofweek"], "polynomial_degree": null, "transform_dict": null, "holiday_countries_used": true, "lags": null, "forward_lags": null}, "holiday_params": null, "trend_method": "local_linear"}, "2": {"decimals": 0, "on_transform": false, "on_inverse": true}}}
Model Number: 83 with model SeasonalityMotif in generation 1 of 1 with params {"window": 5, "point_method": "closest", "distance_metric": "chebyshev", "k": 10, "datepart_method": "expanded_binarized", "independent": true} and transformations {"fillna": "ffill", "transformations": {"0": "bkfilter", "1": "MaxAbsScaler", "2": "StandardScaler", "3": "QuantileTransformer"}, "transformation_params": {"0": {}, "1": {}, "2": {}, "3": {"output_distribution": "uniform", "n_quantiles": 20}}}
Model Number: 84 with model LastValueNaive in generation 1 of 1 with params {} and transformations {"fillna": "KNNImputer", "transformations": {"0": "Slice", "1": "AlignLastValue"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}
Model Number: 85 with model SectionalMotif in generation 1 of 1 with params {"window": 10, "point_method": "mean", "distance_metric": "russellrao", "include_differenced": true, "k": 10, "stride_size": 1, "regression_type": null} and transformations {"fillna": "pchip", "transformations": {"0": "PCA", "1": "AlignLastValue", "2": "DiffSmoother", "3": "QuantileTransformer"}, "transformation_params": {"0": {"whiten": false, "n_components": null}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}, "2": {"method": "rolling_zscore", "method_params": {"distribution": "gamma", "alpha": 0.1, "rolling_periods": 200, "center": true}, "transform_dict": null, "reverse_alignment": true, "isolated_only": true, "fillna": "linear"}, "3": {"output_distribution": "uniform", "n_quantiles": 292}}}
Model Number: 86 with model SeasonalityMotif in generation 1 of 1 with params {"window": 10, "point_method": "weighted_mean", "distance_metric": "mse", "k": 10, "datepart_method": "common_fourier", "independent": true} and transformations {"fillna": "time", "transformations": {"0": "bkfilter", "1": "SeasonalDifference", "2": "StandardScaler", "3": "QuantileTransformer"}, "transformation_params": {"0": {}, "1": {"lag_1": 7, "method": "Mean"}, "2": {}, "3": {"output_distribution": "uniform", "n_quantiles": 20}}}
Model Number: 87 with model SectionalMotif in generation 1 of 1 with params {"window": 10, "point_method": "median", "distance_metric": "cityblock", "include_differenced": true, "k": 10, "stride_size": 1, "regression_type": "User"} and transformations {"fillna": "ffill", "transformations": {"0": "Slice", "1": "RegressionFilter", "2": "LevelShiftTransformer"}, "transformation_params": {"0": {"method": 0.5}, "1": {"sigma": 3, "rolling_window": 90, "run_order": "season_first", "regression_params": {"regression_model": {"model": "ElasticNet", "model_params": {"l1_ratio": 0.5, "fit_intercept": true, "selection": "cyclic"}}, "datepart_method": "recurring", "polynomial_degree": null, "transform_dict": {"fillna": null, "transformations": {"0": "EWMAFilter"}, "transformation_params": {"0": {"span": 7}}}, "holiday_countries_used": false, "lags": null, "forward_lags": null}, "holiday_params": null, "trend_method": "rolling_mean"}, "2": {"window_size": 90, "alpha": 2.5, "grouping_forward_limit": 3, "max_level_shifts": 5, "alignment": "average"}}}
Model Number: 88 with model AverageValueNaive in generation 1 of 1 with params {"method": "Weighted_Mean", "window": null} and transformations {"fillna": "ffill_mean_biased", "transformations": {"0": "ClipOutliers", "1": "QuantileTransformer", "2": "cffilter", "3": "AlignLastValue", "4": "PositiveShift"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 3, "fillna": null}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "max"}, "4": {}}}
Model Number: 89 with model ConstantNaive in generation 1 of 1 with params {"constant": 0} and transformations {"fillna": "ffill", "transformations": {"0": "StandardScaler", "1": "Constraint", "2": "FastICA", "3": "DifferencedTransformer"}, "transformation_params": {"0": {}, "1": {"constraint_method": "quantile", "constraint_direction": "lower", "constraint_regularization": 1.0, "constraint_value": 0.01}, "2": {"algorithm": "deflation", "fun": "exp", "max_iter": 500, "whiten": "unit-variance"}, "3": {"lag": 1, "fill": "bfill"}}}
TotalRuntime missing in 2!
Validation Round: 1
Validation train index is DatetimeIndex(['2020-02-23', '2020-02-24', '2020-02-25', '2020-02-26',
'2020-02-27', '2020-02-28', '2020-02-29', '2020-03-01',
'2020-03-02', '2020-03-03',
...
'2023-03-30', '2023-03-31', '2023-04-01', '2023-04-02',
'2023-04-03', '2023-04-04', '2023-04-05', '2023-04-06',
'2023-04-07', '2023-04-08'],
dtype='datetime64[ns]', name='Date', length=1141, freq=None)
Model Number: 1 of 14 with model AverageValueNaive for Validation 1 with params {"method": "Weighted_Mean", "window": 2} and transformations {"fillna": "KNNImputer", "transformations": {"0": "Slice", "1": "AlignLastValue"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}
📈 1 - AverageValueNaive with avg smape 21.02:
Model Number: 2 of 14 with model AverageValueNaive for Validation 1 with params {"method": "Weighted_Mean", "window": 12} and transformations {"fillna": "ffill_mean_biased", "transformations": {"0": "Slice", "1": "AlignLastValue"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}
2 - AverageValueNaive with avg smape 25.53:
Model Number: 3 of 14 with model SeasonalNaive for Validation 1 with params {"method": "lastvalue", "lag_1": 7, "lag_2": 168} and transformations {"fillna": "zero", "transformations": {"0": "SeasonalDifference", "1": "RegressionFilter", "2": "Round"}, "transformation_params": {"0": {"lag_1": 7, "method": "LastValue"}, "1": {"sigma": 2, "rolling_window": 90, "run_order": "trend_first", "regression_params": {"regression_model": {"model": "DecisionTree", "model_params": {"max_depth": null, "min_samples_split": 0.05}}, "datepart_method": ["weekdaymonthofyear", "quarter", "dayofweek"], "polynomial_degree": null, "transform_dict": null, "holiday_countries_used": true, "lags": null, "forward_lags": null}, "holiday_params": null, "trend_method": "local_linear"}, "2": {"decimals": 0, "on_transform": false, "on_inverse": true}}}
3 - SeasonalNaive with avg smape 63.63:
Model Number: 4 of 14 with model SeasonalityMotif for Validation 1 with params {"window": 5, "point_method": "closest", "distance_metric": "chebyshev", "k": 10, "datepart_method": "expanded_binarized", "independent": true} and transformations {"fillna": "ffill", "transformations": {"0": "bkfilter", "1": "MaxAbsScaler", "2": "StandardScaler", "3": "QuantileTransformer"}, "transformation_params": {"0": {}, "1": {}, "2": {}, "3": {"output_distribution": "uniform", "n_quantiles": 20}}}
4 - SeasonalityMotif with avg smape 69.36:
Model Number: 5 of 14 with model ConstantNaive for Validation 1 with params {"constant": 0} and transformations {"fillna": "ffill", "transformations": {"0": "PowerTransformer", "1": "QuantileTransformer", "2": "SeasonalDifference"}, "transformation_params": {"0": {}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {"lag_1": 7, "method": "LastValue"}}}
5 - ConstantNaive with avg smape 63.63:
Model Number: 6 of 14 with model ConstantNaive for Validation 1 with params {"constant": 0} and transformations {"fillna": "ffill", "transformations": {"0": "StandardScaler", "1": "Constraint", "2": "FastICA", "3": "DifferencedTransformer"}, "transformation_params": {"0": {}, "1": {"constraint_method": "quantile", "constraint_direction": "lower", "constraint_regularization": 1.0, "constraint_value": 0.01}, "2": {"algorithm": "deflation", "fun": "exp", "max_iter": 500, "whiten": "unit-variance"}, "3": {"lag": 1, "fill": "bfill"}}}
6 - ConstantNaive with avg smape 21.02:
Model Number: 7 of 14 with model AverageValueNaive for Validation 1 with params {"method": "Mean", "window": null} and transformations {"fillna": "mean", "transformations": {"0": "ClipOutliers", "1": "QuantileTransformer", "2": "DifferencedTransformer"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 3, "fillna": null}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {}}}
7 - AverageValueNaive with avg smape 22.15:
Model Number: 8 of 14 with model SeasonalityMotif for Validation 1 with params {"window": 10, "point_method": "median", "distance_metric": "mse", "k": 10, "datepart_method": "expanded_binarized", "independent": false} and transformations {"fillna": "fake_date", "transformations": {"0": "MinMaxScaler"}, "transformation_params": {"0": {}}}
8 - SeasonalityMotif with avg smape 44.34:
Model Number: 9 of 14 with model ConstantNaive for Validation 1 with params {"constant": 0.1} and transformations {"fillna": "mean", "transformations": {"0": "ClipOutliers", "1": "Detrend", "2": "SeasonalDifference", "3": "AlignLastValue"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 2, "fillna": null}, "1": {"model": "GLS", "phi": 1, "window": null, "transform_dict": null}, "2": {"lag_1": 12, "method": "Median"}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}}}
9 - ConstantNaive with avg smape 22.3:
Model Number: 10 of 14 with model LastValueNaive for Validation 1 with params {} and transformations {"fillna": "mean", "transformations": {"0": "ClipOutliers", "1": "QuantileTransformer"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 1, "fillna": null}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}}}
10 - LastValueNaive with avg smape 21.02:
Model Number: 11 of 14 with model LastValueNaive for Validation 1 with params {} and transformations {"fillna": "rolling_mean_24", "transformations": {"0": "EWMAFilter", "1": "MinMaxScaler", "2": "LevelShiftTransformer", "3": "AlignLastValue"}, "transformation_params": {"0": {"span": 3}, "1": {}, "2": {"window_size": 90, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 30, "alignment": "last_value"}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "max"}}}
11 - LastValueNaive with avg smape 46.88:
Model Number: 12 of 14 with model SeasonalityMotif for Validation 1 with params {"window": 10, "point_method": "median", "distance_metric": "minkowski", "k": 20, "datepart_method": "simple_2", "independent": false} and transformations {"fillna": "time", "transformations": {"0": "bkfilter", "1": "MaxAbsScaler", "2": "StandardScaler", "3": "QuantileTransformer", "4": "cffilter"}, "transformation_params": {"0": {}, "1": {}, "2": {}, "3": {"output_distribution": "uniform", "n_quantiles": 20}, "4": {}}}
12 - SeasonalityMotif with avg smape 57.12:
Model Number: 13 of 14 with model LastValueNaive for Validation 1 with params {} and transformations {"fillna": "KNNImputer", "transformations": {"0": "Slice", "1": "AlignLastValue", "2": "AlignLastValue"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}, "2": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "max"}}}
13 - LastValueNaive with avg smape 21.02:
Model Number: 14 of 14 with model SeasonalNaive for Validation 1 with params {"method": "lastvalue", "lag_1": 24, "lag_2": 28} and transformations {"fillna": "fake_date", "transformations": {"0": "Slice", "1": "AlignLastValue", "2": "Round"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}, "2": {"decimals": 0, "on_transform": false, "on_inverse": true}}}
14 - SeasonalNaive with avg smape 24.21:
Validation Round: 2
Validation train index is DatetimeIndex(['2020-02-23', '2020-02-24', '2020-02-25', '2020-02-26',
'2020-02-27', '2020-02-28', '2020-02-29', '2020-03-01',
'2020-03-02', '2020-03-03',
...
'2023-02-28', '2023-03-01', '2023-03-02', '2023-03-03',
'2023-03-04', '2023-03-05', '2023-03-06', '2023-03-07',
'2023-03-08', '2023-03-09'],
dtype='datetime64[ns]', name='Date', length=1111, freq=None)
Model Number: 1 of 14 with model AverageValueNaive for Validation 2 with params {"method": "Weighted_Mean", "window": 2} and transformations {"fillna": "KNNImputer", "transformations": {"0": "Slice", "1": "AlignLastValue"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}
📈 1 - AverageValueNaive with avg smape 61.53:
Model Number: 2 of 14 with model AverageValueNaive for Validation 2 with params {"method": "Weighted_Mean", "window": 12} and transformations {"fillna": "ffill_mean_biased", "transformations": {"0": "Slice", "1": "AlignLastValue"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}}}
2 - AverageValueNaive with avg smape 61.53:
Model Number: 3 of 14 with model SeasonalNaive for Validation 2 with params {"method": "lastvalue", "lag_1": 7, "lag_2": 168} and transformations {"fillna": "zero", "transformations": {"0": "SeasonalDifference", "1": "RegressionFilter", "2": "Round"}, "transformation_params": {"0": {"lag_1": 7, "method": "LastValue"}, "1": {"sigma": 2, "rolling_window": 90, "run_order": "trend_first", "regression_params": {"regression_model": {"model": "DecisionTree", "model_params": {"max_depth": null, "min_samples_split": 0.05}}, "datepart_method": ["weekdaymonthofyear", "quarter", "dayofweek"], "polynomial_degree": null, "transform_dict": null, "holiday_countries_used": true, "lags": null, "forward_lags": null}, "holiday_params": null, "trend_method": "local_linear"}, "2": {"decimals": 0, "on_transform": false, "on_inverse": true}}}
3 - SeasonalNaive with avg smape 61.53:
Model Number: 4 of 14 with model SeasonalityMotif for Validation 2 with params {"window": 5, "point_method": "closest", "distance_metric": "chebyshev", "k": 10, "datepart_method": "expanded_binarized", "independent": true} and transformations {"fillna": "ffill", "transformations": {"0": "bkfilter", "1": "MaxAbsScaler", "2": "StandardScaler", "3": "QuantileTransformer"}, "transformation_params": {"0": {}, "1": {}, "2": {}, "3": {"output_distribution": "uniform", "n_quantiles": 20}}}
4 - SeasonalityMotif with avg smape 61.53:
Model Number: 5 of 14 with model ConstantNaive for Validation 2 with params {"constant": 0} and transformations {"fillna": "ffill", "transformations": {"0": "PowerTransformer", "1": "QuantileTransformer", "2": "SeasonalDifference"}, "transformation_params": {"0": {}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {"lag_1": 7, "method": "LastValue"}}}
5 - ConstantNaive with avg smape 61.53:
Model Number: 6 of 14 with model ConstantNaive for Validation 2 with params {"constant": 0} and transformations {"fillna": "ffill", "transformations": {"0": "StandardScaler", "1": "Constraint", "2": "FastICA", "3": "DifferencedTransformer"}, "transformation_params": {"0": {}, "1": {"constraint_method": "quantile", "constraint_direction": "lower", "constraint_regularization": 1.0, "constraint_value": 0.01}, "2": {"algorithm": "deflation", "fun": "exp", "max_iter": 500, "whiten": "unit-variance"}, "3": {"lag": 1, "fill": "bfill"}}}
6 - ConstantNaive with avg smape 61.53:
Model Number: 7 of 14 with model AverageValueNaive for Validation 2 with params {"method": "Mean", "window": null} and transformations {"fillna": "mean", "transformations": {"0": "ClipOutliers", "1": "QuantileTransformer", "2": "DifferencedTransformer"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 3, "fillna": null}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}, "2": {}}}
📈 7 - AverageValueNaive with avg smape 61.17:
Model Number: 8 of 14 with model SeasonalityMotif for Validation 2 with params {"window": 10, "point_method": "median", "distance_metric": "mse", "k": 10, "datepart_method": "expanded_binarized", "independent": false} and transformations {"fillna": "fake_date", "transformations": {"0": "MinMaxScaler"}, "transformation_params": {"0": {}}}
8 - SeasonalityMotif with avg smape 63.2:
Model Number: 9 of 14 with model ConstantNaive for Validation 2 with params {"constant": 0.1} and transformations {"fillna": "mean", "transformations": {"0": "ClipOutliers", "1": "Detrend", "2": "SeasonalDifference", "3": "AlignLastValue"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 2, "fillna": null}, "1": {"model": "GLS", "phi": 1, "window": null, "transform_dict": null}, "2": {"lag_1": 12, "method": "Median"}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": 1, "threshold_method": "mean"}}}
9 - ConstantNaive with avg smape 61.82:
Model Number: 10 of 14 with model LastValueNaive for Validation 2 with params {} and transformations {"fillna": "mean", "transformations": {"0": "ClipOutliers", "1": "QuantileTransformer"}, "transformation_params": {"0": {"method": "clip", "std_threshold": 1, "fillna": null}, "1": {"output_distribution": "uniform", "n_quantiles": 1000}}}
10 - LastValueNaive with avg smape 61.38:
Model Number: 11 of 14 with model LastValueNaive for Validation 2 with params {} and transformations {"fillna": "rolling_mean_24", "transformations": {"0": "EWMAFilter", "1": "MinMaxScaler", "2": "LevelShiftTransformer", "3": "AlignLastValue"}, "transformation_params": {"0": {"span": 3}, "1": {}, "2": {"window_size": 90, "alpha": 2.0, "grouping_forward_limit": 4, "max_level_shifts": 30, "alignment": "last_value"}, "3": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "max"}}}
11 - LastValueNaive with avg smape 61.53:
Model Number: 12 of 14 with model SeasonalityMotif for Validation 2 with params {"window": 10, "point_method": "median", "distance_metric": "minkowski", "k": 20, "datepart_method": "simple_2", "independent": false} and transformations {"fillna": "time", "transformations": {"0": "bkfilter", "1": "MaxAbsScaler", "2": "StandardScaler", "3": "QuantileTransformer", "4": "cffilter"}, "transformation_params": {"0": {}, "1": {}, "2": {}, "3": {"output_distribution": "uniform", "n_quantiles": 20}, "4": {}}}
12 - SeasonalityMotif with avg smape 69.04:
Model Number: 13 of 14 with model LastValueNaive for Validation 2 with params {} and transformations {"fillna": "KNNImputer", "transformations": {"0": "Slice", "1": "AlignLastValue", "2": "AlignLastValue"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}, "2": {"rows": 1, "lag": 1, "method": "additive", "strength": 1.0, "first_value_only": false, "threshold": null, "threshold_method": "max"}}}
13 - LastValueNaive with avg smape 61.53:
Model Number: 14 of 14 with model SeasonalNaive for Validation 2 with params {"method": "lastvalue", "lag_1": 24, "lag_2": 28} and transformations {"fillna": "fake_date", "transformations": {"0": "Slice", "1": "AlignLastValue", "2": "Round"}, "transformation_params": {"0": {"method": 0.5}, "1": {"rows": 1, "lag": 1, "method": "additive", "strength": 0.9, "first_value_only": false, "threshold": 3, "threshold_method": "mean"}, "2": {"decimals": 0, "on_transform": false, "on_inverse": true}}}
14 - SeasonalNaive with avg smape 61.22:
TotalRuntime missing in 3!
Validation Round: 1
Validation train index is DatetimeIndex(['2020-02-23', '2020-02-24', '2020-02-25', '2020-02-26',
'2020-02-27', '2020-02-28', '2020-02-29', '2020-03-01',
'2020-03-02', '2020-03-03',
...
'2023-03-30', '2023-03-31', '2023-04-01', '2023-04-02',
'2023-04-03', '2023-04-04', '2023-04-05', '2023-04-06',
'2023-04-07', '2023-04-08'],
dtype='datetime64[ns]', name='Date', length=1141, freq=None)
TotalRuntime missing in 0!
Validation Round: 2
Validation train index is DatetimeIndex(['2020-02-23', '2020-02-24', '2020-02-25', '2020-02-26',
'2020-02-27', '2020-02-28', '2020-02-29', '2020-03-01',
'2020-03-02', '2020-03-03',
...
'2023-02-28', '2023-03-01', '2023-03-02', '2023-03-03',
'2023-03-04', '2023-03-05', '2023-03-06', '2023-03-07',
'2023-03-08', '2023-03-09'],
dtype='datetime64[ns]', name='Date', length=1111, freq=None)
TotalRuntime missing in 0!
Model Number: 1 with model Ensemble in generation 0 of Horizontal Ensembles with params {"model_name": "Horizontal", "model_count": 3, "model_metric": "Score", "models": {"7a14af550afa2194472cfc2e4e1440fb": {"Model": "LastValueNaive", "ModelParameters": "{}", "TransformationParameters": "{\"fillna\": \"mean\", \"transformations\": {\"0\": \"ClipOutliers\", \"1\": \"QuantileTransformer\"}, \"transformation_params\": {\"0\": {\"method\": \"clip\", \"std_threshold\": 1, \"fillna\": null}, \"1\": {\"output_distribution\": \"uniform\", \"n_quantiles\": 1000}}}"}, "7220b939d0383941d61ca4c852183d41": {"Model": "LastValueNaive", "ModelParameters": "{}", "TransformationParameters": "{\"fillna\": \"rolling_mean_24\", \"transformations\": {\"0\": \"EWMAFilter\", \"1\": \"MinMaxScaler\", \"2\": \"LevelShiftTransformer\", \"3\": \"AlignLastValue\"}, \"transformation_params\": {\"0\": {\"span\": 3}, \"1\": {}, \"2\": {\"window_size\": 90, \"alpha\": 2.0, \"grouping_forward_limit\": 4, \"max_level_shifts\": 30, \"alignment\": \"last_value\"}, \"3\": {\"rows\": 1, \"lag\": 1, \"method\": \"additive\", \"strength\": 1.0, \"first_value_only\": false, \"threshold\": null, \"threshold_method\": \"max\"}}}"}, "d3cd21cdc05a6c00362cb79eafa32840": {"Model": "ConstantNaive", "ModelParameters": "{\"constant\": 0}", "TransformationParameters": "{\"fillna\": \"ffill\", \"transformations\": {\"0\": \"StandardScaler\", \"1\": \"Constraint\", \"2\": \"FastICA\", \"3\": \"DifferencedTransformer\"}, \"transformation_params\": {\"0\": {}, \"1\": {\"constraint_method\": \"quantile\", \"constraint_direction\": \"lower\", \"constraint_regularization\": 1.0, \"constraint_value\": 0.01}, \"2\": {\"algorithm\": \"deflation\", \"fun\": \"exp\", \"max_iter\": 500, \"whiten\": \"unit-variance\"}, \"3\": {\"lag\": 1, \"fill\": \"bfill\"}}}"}}, "series": {"theta": "7220b939d0383941d61ca4c852183d41", "kappa": "7a14af550afa2194472cfc2e4e1440fb", "rho": "d3cd21cdc05a6c00362cb79eafa32840", "sigma": "7a14af550afa2194472cfc2e4e1440fb"}} and transformations {}
Ensemble Horizontal component 1 of 3 LastValueNaive started
Ensemble Horizontal component 2 of 3 LastValueNaive started
Ensemble Horizontal component 3 of 3 ConstantNaive started
Ensemble Horizontal component 1 of 3 LastValueNaive started
Ensemble Horizontal component 2 of 3 LastValueNaive started
Ensemble Horizontal component 3 of 3 ConstantNaive started
2024-10-03 at 13:27:20 | ERROR | validation of end failed
3. Compare scenarios
As explained with Tutorial: Scenario analysis, we can compare scenarios.
[14]:
# Adjust the last date, appending a phase
snr.append();
2024-10-03 at 13:27:20 | ERROR | validation of end failed
2024-10-03 at 13:27:20 | ERROR | validation of end failed
2024-10-03 at 13:27:20 | ERROR | validation of end failed
[15]:
# Compare reproduction number
ymin = snr.compare_param("Rt", date_range=(future_start_date, None), display=False).min().min()
snr.compare_param("Rt", date_range=(future_start_date, None), ylim=(ymin, None));
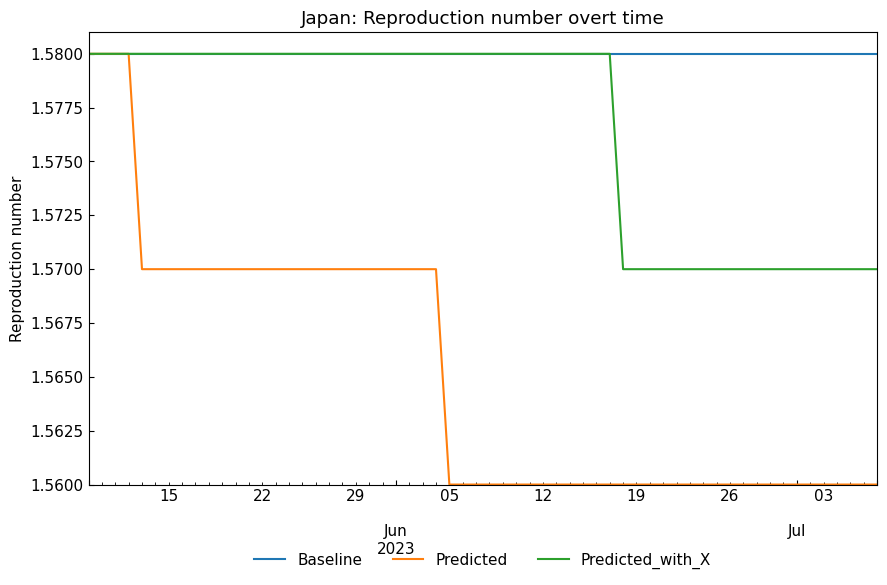
Note that minimum value of y in the figure was changed to focus on the differences of the scenarios.
[16]:
ymin_value = snr.compare_cases("Confirmed", date_range=(future_start_date, None), display=False).Predicted.min()
snr.compare_cases("Confirmed", date_range=(future_start_date, None), ylim=(ymin_value, None));
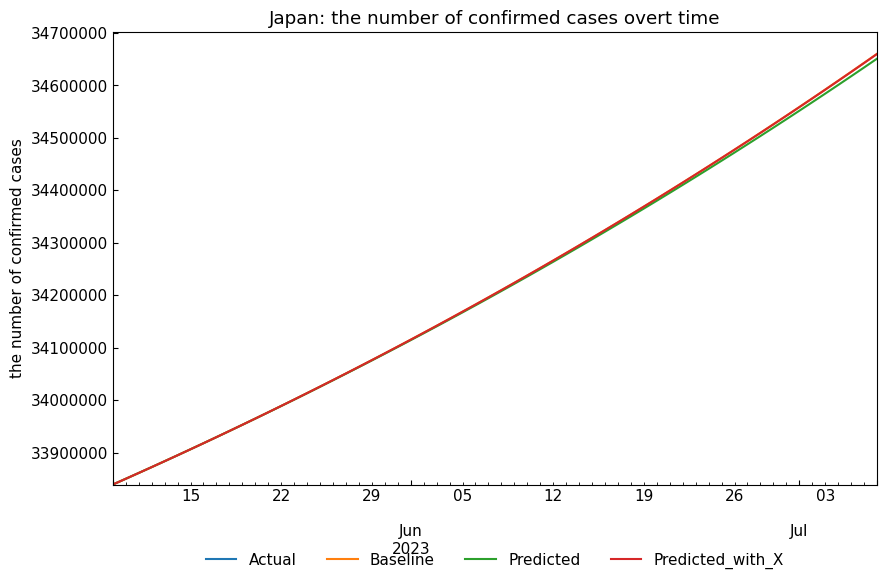
[17]:
# Show representative values
snr.describe()
[17]:
max(Infected) | argmax(Infected) | Confirmed on 07Jul2023 | Infected on 07Jul2023 | Fatal on 07Jul2023 | |
---|---|---|---|---|---|
Baseline | 3763514.0 | 2022-08-15 | 34522070.0 | 297356.0 | 76222.0 |
Predicted | 3763514.0 | 2022-08-15 | 34482366.0 | 262120.0 | 76129.0 |
Predicted_with_X | 3763514.0 | 2022-08-15 | 34516873.0 | 289729.0 | 76210.0 |
Thank you!